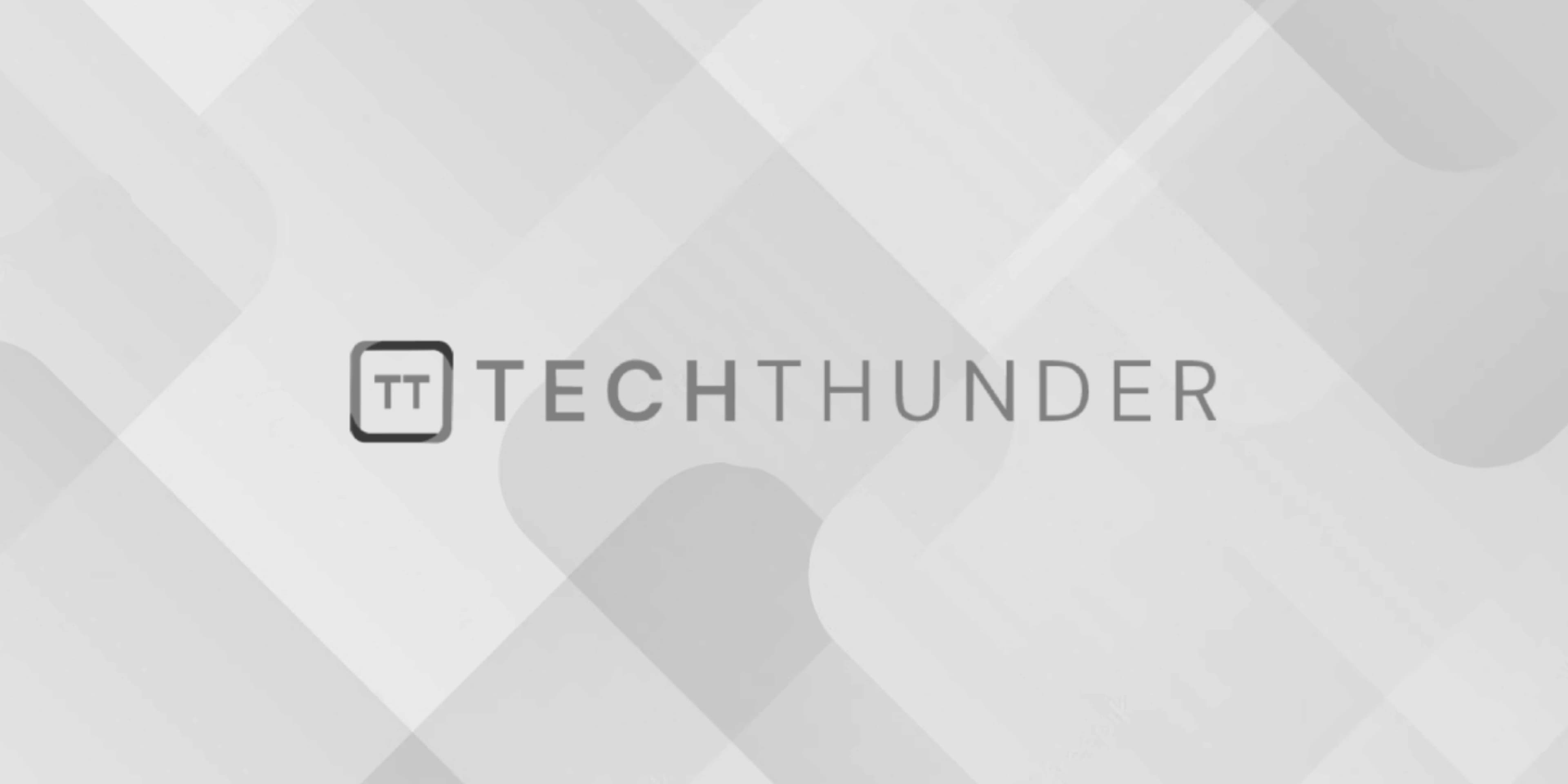
223 views
Adding Two Strings in C++
The C++ concatenate (add) two strings together using the +
operator or various string member functions provided by the C++ Standard Library. Here are several ways to concatenate two strings:
- Using the
+
Operator:
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // Concatenate str1 and str2 using the + operator
std::cout << "Concatenated string: " << result << std::endl;
return 0;
}
- Using the
append
Member Function:
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
str1.append(str2); // Concatenate str2 to str1 using the append function
std::cout << "Concatenated string: " << str1 << std::endl;
return 0;
}
- Using the
+=
Operator:
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
str1 += str2; // Concatenate str2 to str1 using the += operator
std::cout << "Concatenated string: " << str1 << std::endl;
return 0;
}
All of these methods achieve the same result, which is the concatenation of str1
and str2
into the result
string. You can choose the method that you find most convenient for your specific use case.