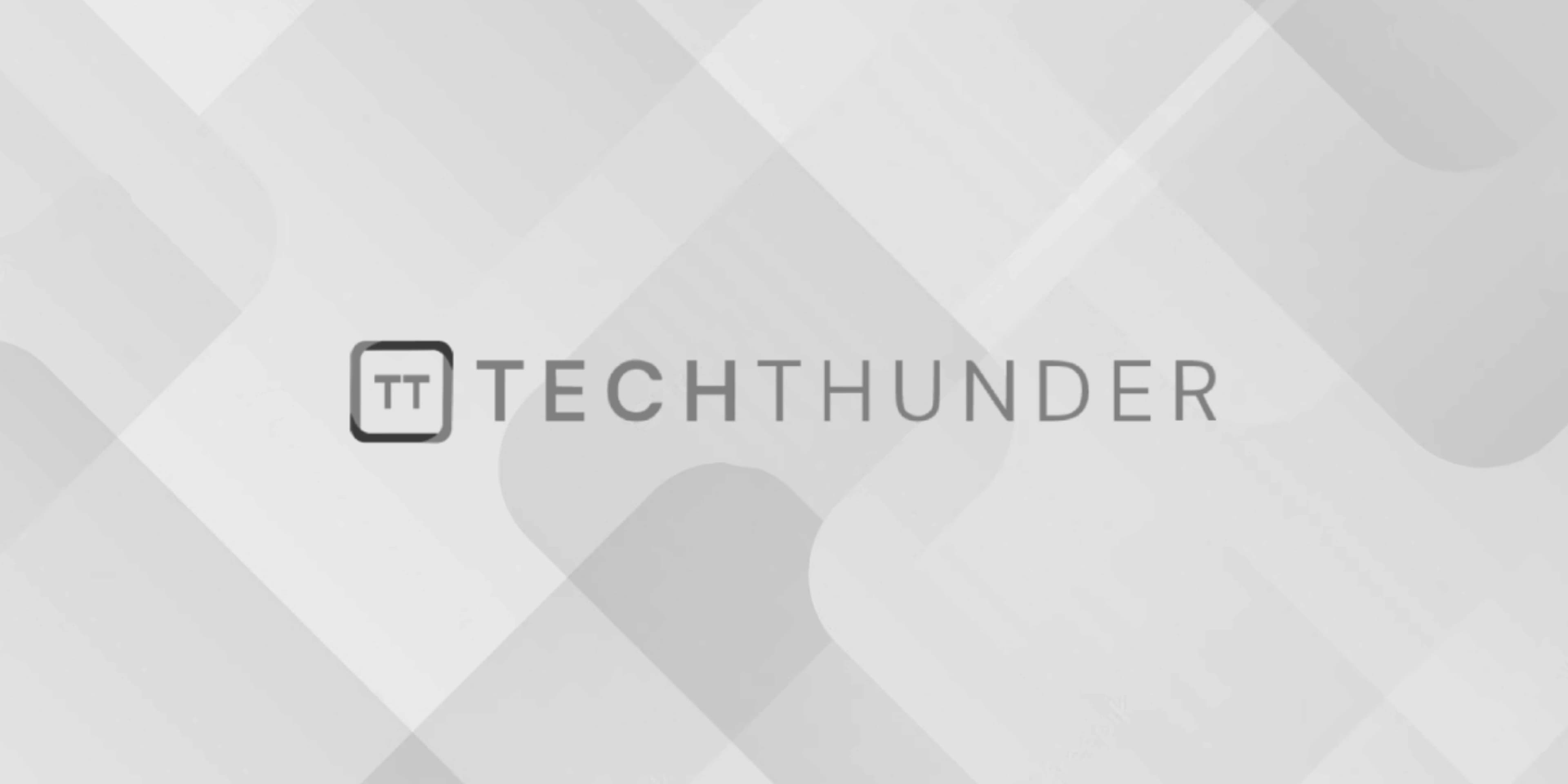
196 views
Palindrome Number in C++
A palindrome number is a number that remains the same when its digits are reversed. Here’s a C++ program to check if a given integer is a palindrome:
C++
#include <iostream>
int main() {
int number, originalNumber, reversedNumber = 0;
// Input an integer
std::cout << "Enter an integer: ";
std::cin >> number;
originalNumber = number;
while (number > 0) {
int digit = number % 10; // Get the last digit
reversedNumber = reversedNumber * 10 + digit; // Add the digit to the reversed number
number /= 10; // Remove the last digit
}
if (originalNumber == reversedNumber) {
std::cout << originalNumber << " is a palindrome." << std::endl;
} else {
std::cout << originalNumber << " is not a palindrome." << std::endl;
}
return 0;
}
In this program:
- The user is prompted to enter an integer.
- A
while
loop is used to reverse the number digit by digit. In each iteration, the last digit of the original number is extracted using the modulo operator (% 10
) and added to the reversed number after shifting the reversed number one position to the left by multiplying it by 10. The last digit is then removed from the original number using integer division (/ 10
). - The result is compared with the original number to check if they are the same. If they are the same, the number is a palindrome; otherwise, it’s not.
Compile and run this program, and it will check if the entered integer is a palindrome.