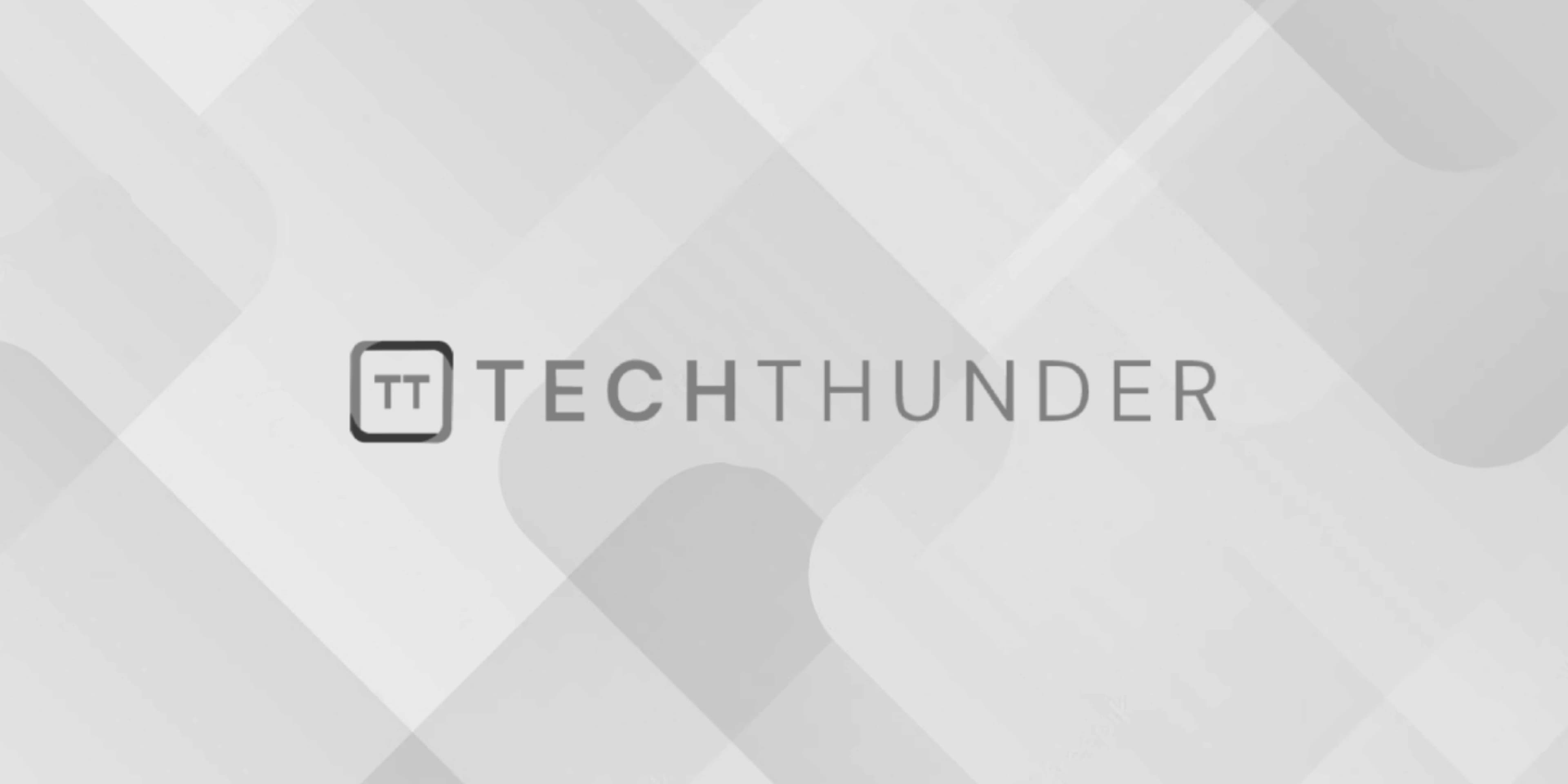
Char array to string in C++
Converting a char array to a string in C++ can be done using various methods. Below are a few common ways to accomplish this:
Method 1: Using a Constructor
You can use the constructor of the std::string
class to initialize a string from a char array. Here’s an example:
#include <iostream>
#include <string>
int main() {
char charArray[] = "Hello, World!";
// Convert char array to string using constructor
std::string str(charArray);
std::cout << "String: " << str << std::endl;
return 0;
}
In this method, the std::string
constructor takes the char array as an argument and creates a string from it.
Method 2: Using the Assignment Operator
You can assign a char array to a string using the assignment operator (=
). Here’s an example:
#include <iostream>
#include <string>
int main() {
char charArray[] = "Hello, World!";
// Convert char array to string using assignment
std::string str = charArray;
std::cout << "String: " << str << std::endl;
return 0;
}
In this method, the assignment operator assigns the value of the char array to the string.
Method 3: Using a Loop
You can manually iterate through the char array and append each character to a string. Here’s an example:
#include <iostream>
#include <string>
int main() {
char charArray[] = "Hello, World!";
// Convert char array to string using a loop
std::string str;
for (int i = 0; charArray[i] != '\0'; ++i) {
str += charArray[i];
}
std::cout << "String: " << str << std::endl;
return 0;
}
In this method, a loop is used to iterate through the char array and append each character to the string until the null-terminator ('\0'
) is encountered.
Any of these methods can be used to convert a char array to a string in C++. Choose the one that best fits your needs and programming style.