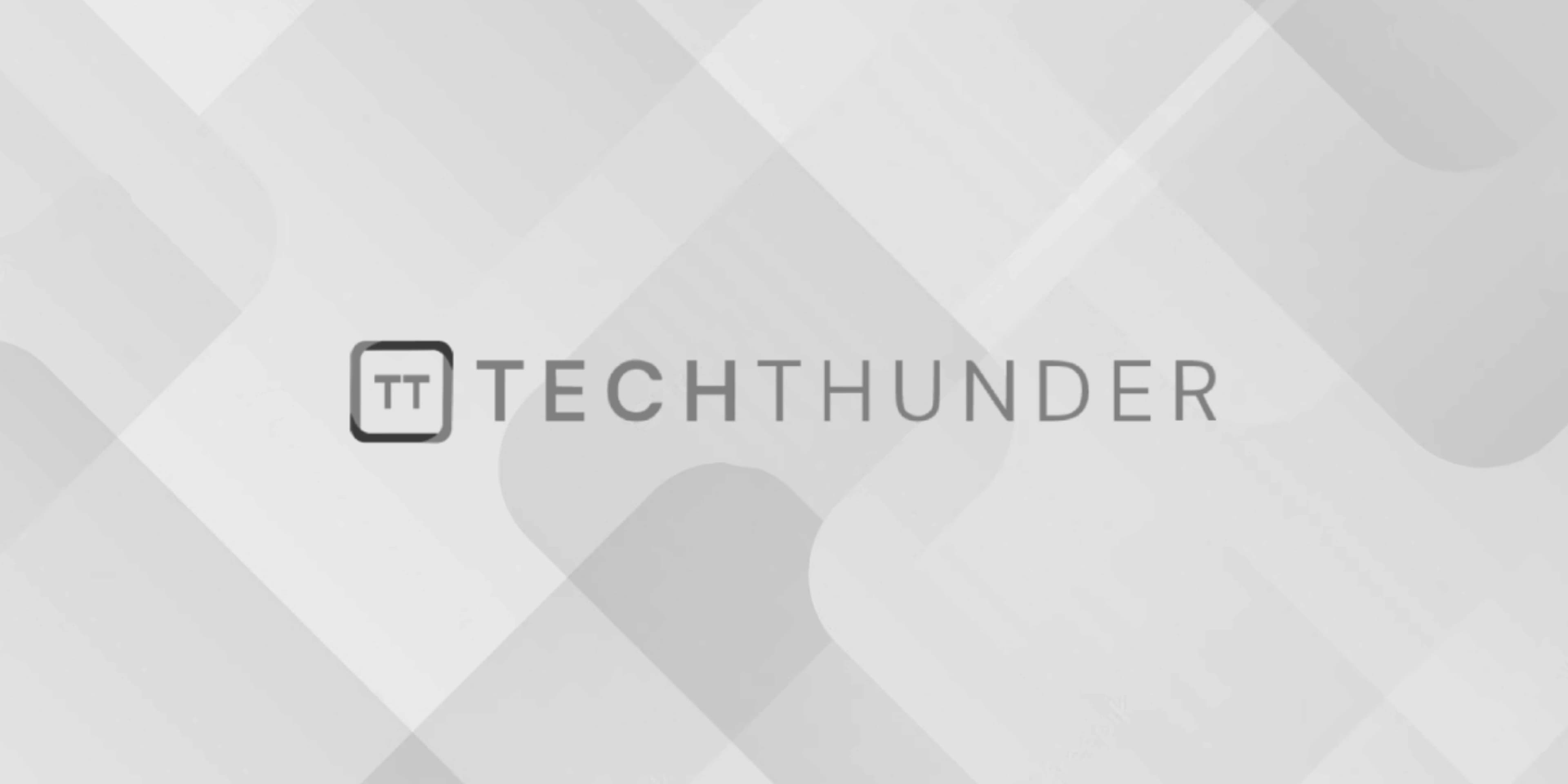
Static Member Function in C++
The C++ static member function is a function that belongs to a class rather than an instance of the class. Static member functions are called on the class itself rather than on objects of the class. They are typically used for operations that do not depend on the state of a particular object but are related to the class as a whole. Here’s how you declare and use static member functions in C++:
Declaration:
To declare a static member function, you use the static
keyword in the function declaration within the class definition. Static member functions have the following characteristics:
- They do not have access to non-static (instance) members of the class unless they are explicitly passed as parameters.
- They can be called using the class name and the scope resolution operator
::
or on an object of the class. - They do not have a
this
pointer, as they are not associated with a specific instance of the class.
Here’s an example of declaring a static member function in a C++ class:
class MyClass {
public:
static int staticFunction(); // Declaration of a static member function
};
Definition:
To define a static member function outside of the class definition, you use the class name followed by the scope resolution operator ::
. You do not use the static
keyword in the definition.
int MyClass::staticFunction() {
// Implementation of the static member function
// This function can't access non-static data members of MyClass
return 42;
}
Usage:
You can call a static member function either on the class itself or on an object of the class. Here’s how you can use it:
int main() {
int result1 = MyClass::staticFunction();
// Calling the static function using the class name
MyClass obj;
int result2 = obj.staticFunction();
// Calling the static function on an object of the class
return 0;
}
Static member functions are often used for utility functions that don’t depend on the state of any particular object but are related to the class. They can be called without creating an instance of the class and are sometimes used for operations like factory methods, helper functions, or to access class-level data or constants.