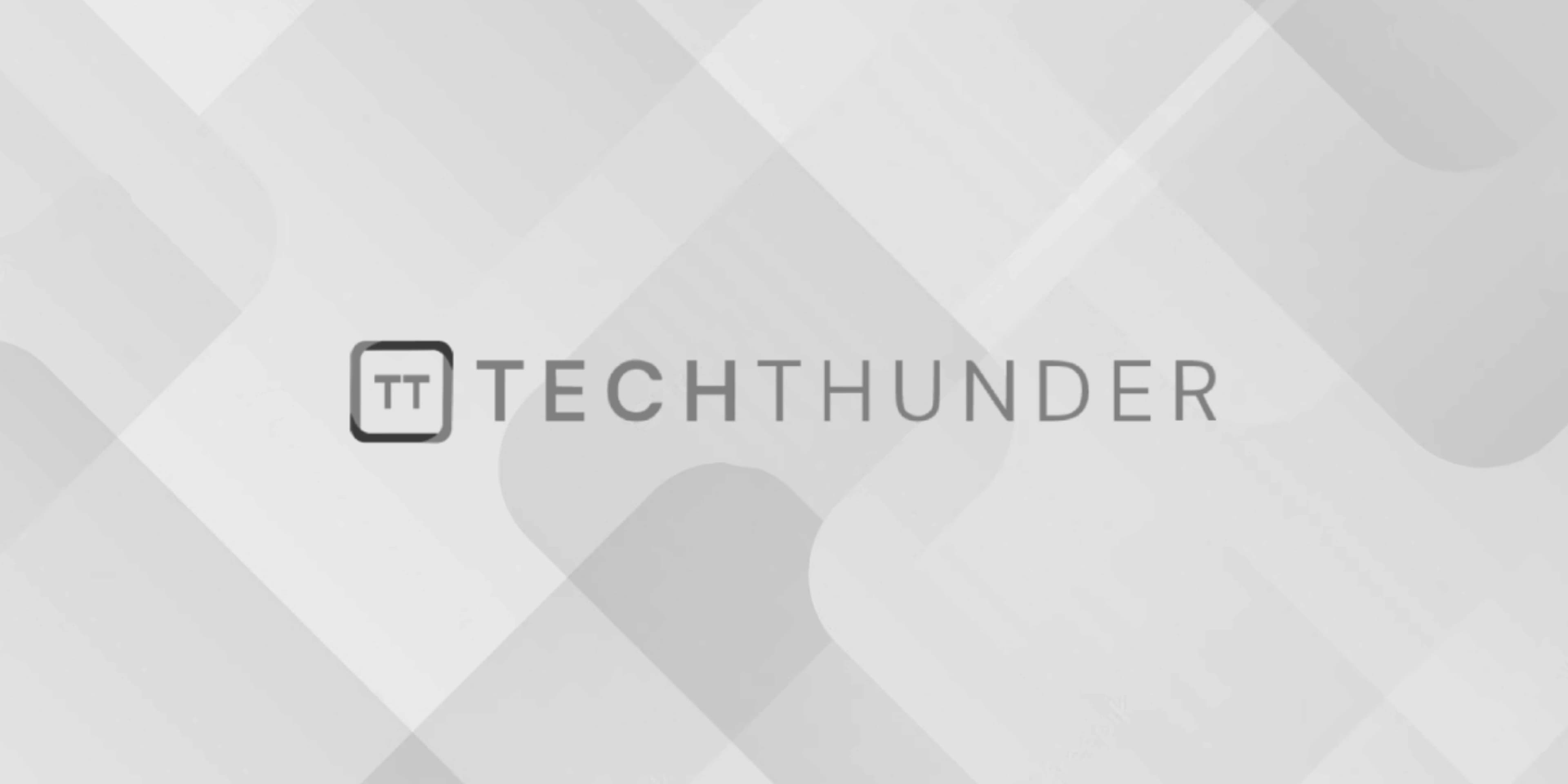
263 views
Password Validation in C++
To implement password validation in C++, you can create a program that checks whether a given password meets certain criteria, such as length, complexity, and character requirements. Here’s a simple C++ program to validate a password:
C++
#include <iostream>
#include <string>
#include <cctype> // Include the <cctype> header for character checks
bool isStrongPassword(const std::string& password) {
// Check the length of the password
if (password.length() < 8) {
return false;
}
// Initialize flags for character types
bool hasDigit = false;
bool hasUpperCase = false;
bool hasLowerCase = false;
// Check each character in the password
for (char ch : password) {
if (std::isdigit(ch)) {
hasDigit = true;
} else if (std::isupper(ch)) {
hasUpperCase = true;
} else if (std::islower(ch)) {
hasLowerCase = true;
}
}
// Check if all required character types are present
return hasDigit && hasUpperCase && hasLowerCase;
}
int main() {
std::string password;
// Input the password from the user
std::cout << "Enter a password: ";
std::cin >> password;
// Validate the password
if (isStrongPassword(password)) {
std::cout << "Password is strong and valid." << std::endl;
} else {
std::cout << "Password is weak or invalid." << std::endl;
}
return 0;
}
In this program:
- We define a
isStrongPassword
function that takes a string (password
) as input and returnstrue
if the password is strong (meets the criteria) andfalse
otherwise. - The password is considered strong if it meets the following criteria:
- It has a minimum length of 8 characters.
- It contains at least one digit.
- It contains at least one uppercase letter.
- It contains at least one lowercase letter.
- In the
main
function, we input a password from the user and then call theisStrongPassword
function to validate it. We display a message indicating whether the password is strong and valid or weak and invalid.
You can modify the criteria for password strength according to your requirements by adjusting the conditions in the isStrongPassword
function.