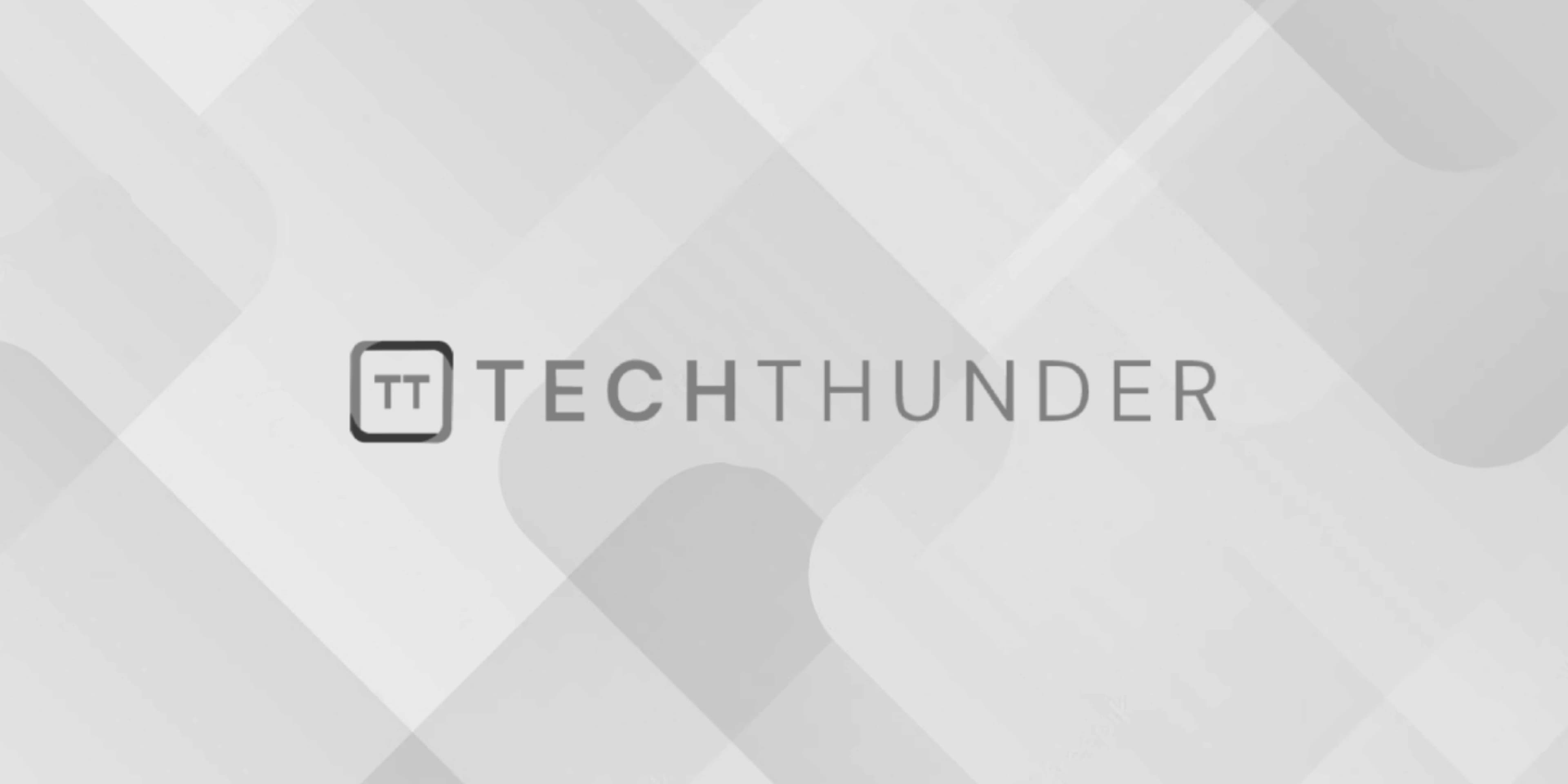
116 views
C++ program to find greatest of four numbers
You can find the greatest of four numbers in C++ by comparing them using conditional statements. Here’s a C++ program to find the greatest of four numbers:
C++
#include <iostream>
int main() {
int num1, num2, num3, num4;
// Input four numbers
std::cout << "Enter four numbers: ";
std::cin >> num1 >> num2 >> num3 >> num4;
int greatest = num1; // Assume the first number is the greatest initially
// Compare the other three numbers with the greatest
if (num2 > greatest) {
greatest = num2;
}
if (num3 > greatest) {
greatest = num3;
}
if (num4 > greatest) {
greatest = num4;
}
// Print the greatest number
std::cout << "The greatest number is: " << greatest << std::endl;
return 0;
}
In this program:
- We declare four integer variables
num1
,num2
,num3
, andnum4
to store the four input numbers. - We use
std::cout
to prompt the user to enter the four numbers and then usestd::cin
to read those numbers into the respective variables. - We assume that the first number (
num1
) is the greatest initially and store it in thegreatest
variable. - We compare the other three numbers (
num2
,num3
, andnum4
) with the greatest number using a series ofif
statements. If any of these numbers are greater than the currentgreatest
, we update thegreatest
variable accordingly. - Finally, we print the greatest number to the console.
When you run this program, it will determine and display the greatest of the four input numbers.