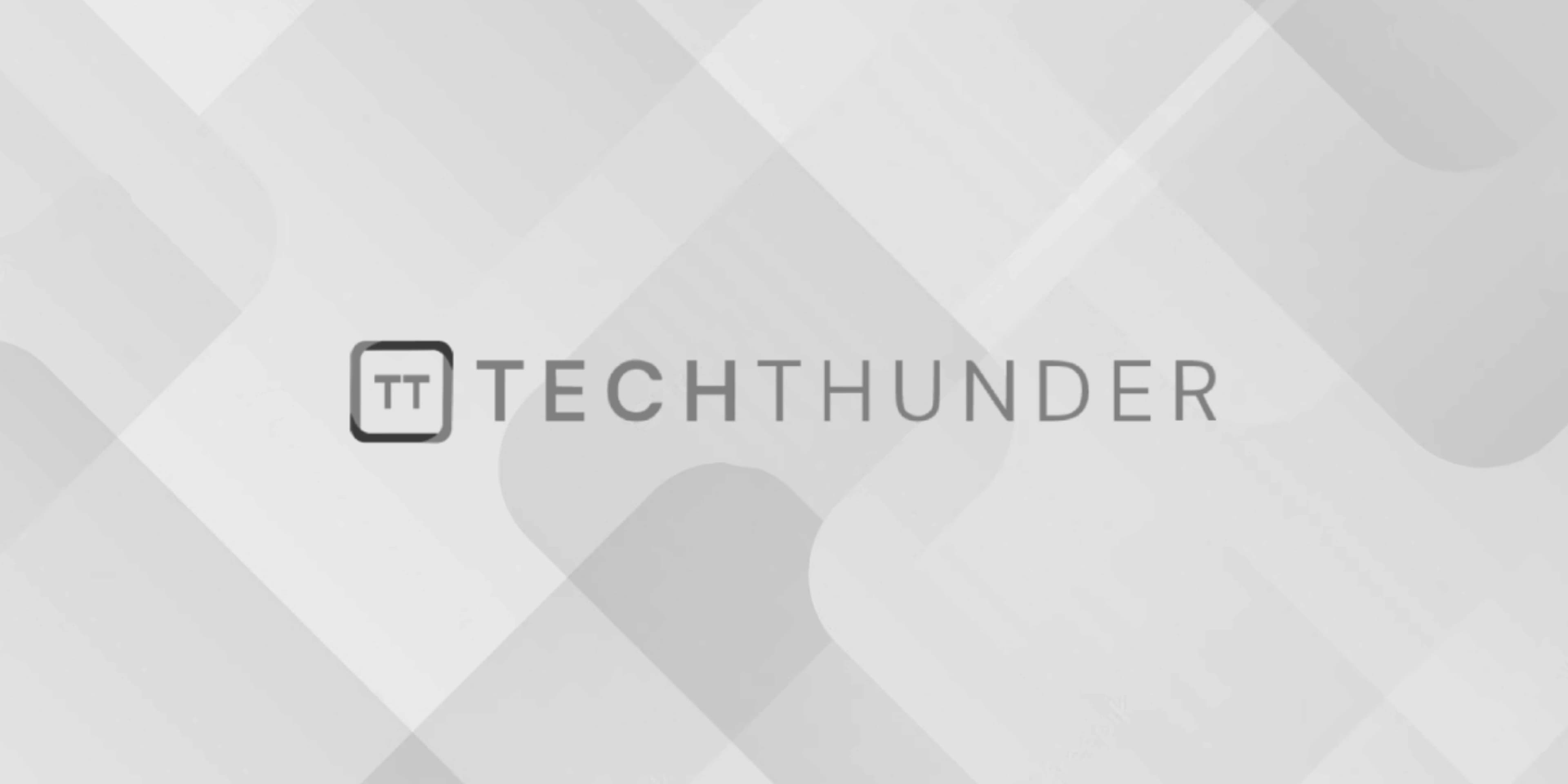
Private Inheritance in C++
Private inheritance is one of the three types of class inheritance in C++, along with public and protected inheritance. With private inheritance, a derived class inherits the members of a base class, but these inherited members become private members in the derived class, effectively hiding them from the outside world. This means that the derived class can access the inherited members, but they are not visible to external code.
Here’s how private inheritance works in C++:
class Base {
public:
void publicFunction() {
std::cout << "Public function in Base" << std::endl;
}
void sharedFunction() {
std::cout << "Shared function in Base" << std::endl;
}
};
class Derived : private Base {
public:
void callBaseFunctions() {
publicFunction(); // Accesses publicFunction from Base
sharedFunction(); // Accesses sharedFunction from Base
}
void derivedFunction() {
std::cout << "Derived function" << std::endl;
}
};
int main() {
Derived derived;
// The following line will result in a compilation error
// derived.publicFunction();
// The following line will result in a compilation error
// derived.sharedFunction();
derived.callBaseFunctions(); // Calls the Base class functions through Derived
derived.derivedFunction(); // Calls the Derived class function
return 0;
}
In this example:
- The
Base
class has two public member functions:publicFunction()
andsharedFunction()
. - The
Derived
class privately inherits fromBase
using the syntaxclass Derived : private Base
. This means that all public and protected members ofBase
become private members ofDerived
. - The
Derived
class can still access the inherited members ofBase
internally. In thecallBaseFunctions()
member function, it demonstrates how the private members ofBase
can be used withinDerived
. - Outside of the
Derived
class, attempts to accesspublicFunction()
andsharedFunction()
directly result in compilation errors because these functions are treated as private members ofDerived
.
Private inheritance is useful in situations where you want to reuse the implementation of a base class but don’t want to expose its interface directly to the outside world. It allows you to encapsulate and control access to the base class’s functionality while still benefiting from code reuse.
Common use cases for private inheritance include:
- Implementation Inheritance: When you want to reuse the implementation details of a base class without exposing its interface.
- Implementing Interfaces: When you want to implement an interface but don’t want to expose all of the interface’s methods.
- Enhancing Encapsulation: When you want to tightly control access to the base class’s members and only expose selected functionality to the outside world.
However, private inheritance should be used with caution because it can make the code less intuitive and may require more careful design and documentation. In most cases, composition (using an instance of the base class as a member of the derived class) or public/protected inheritance is preferred.