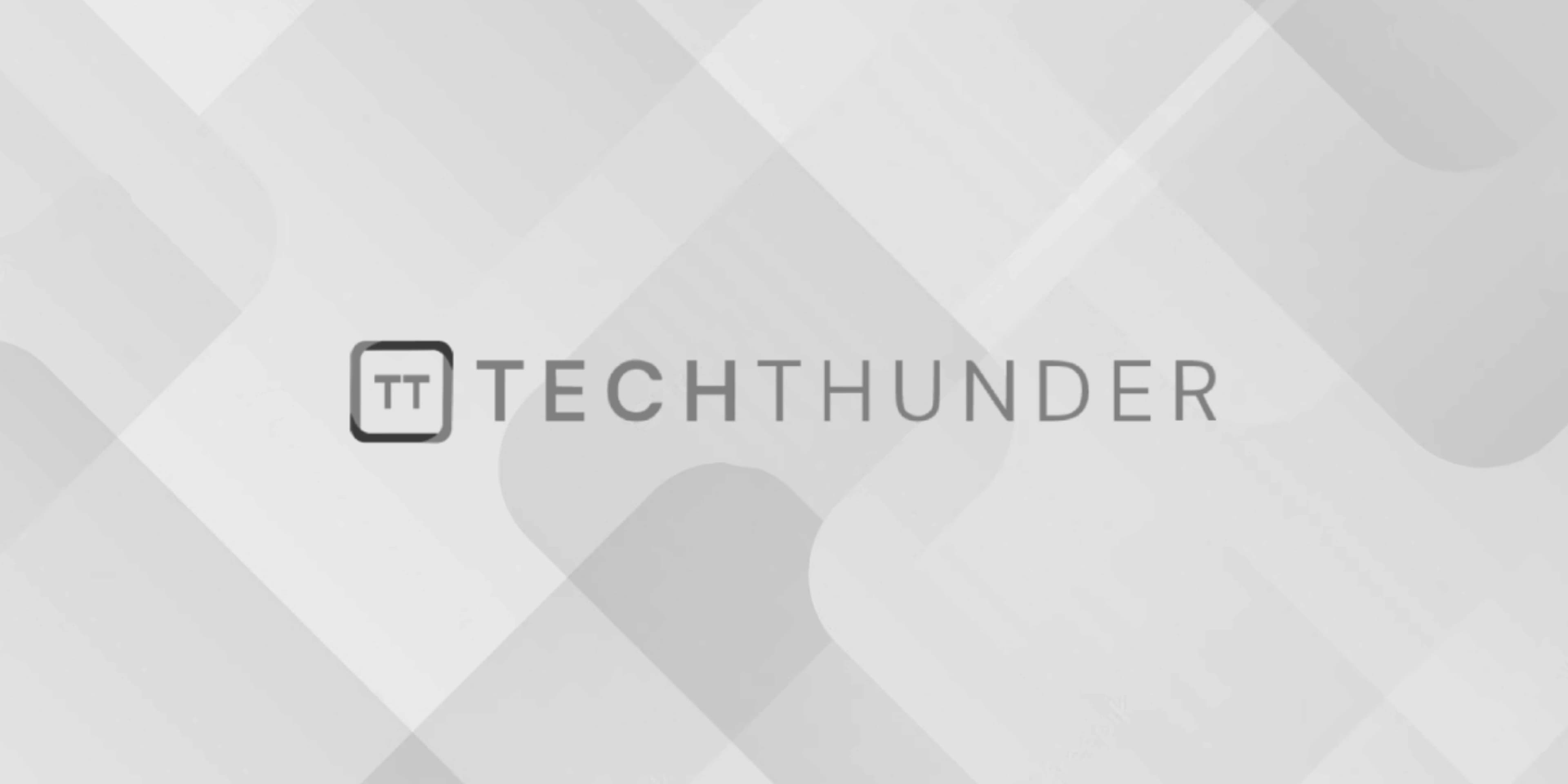
C++ Vector
The vector is a dynamic array-like container provided by the C++ Standard Library (part of the Standard Template Library, or STL). Vectors are similar to arrays but offer dynamic sizing, automatic memory management, and a range of convenient methods for manipulating data.
Here’s how to work with vectors in C++:
1. Include the necessary header:
#include <vector>
2. Declare a vector:
std::vector<T> myVector;
- Replace
T
with the data type of the elements you want to store in the vector.
3. Add elements to the vector:
You can add elements to a vector using various methods:
push_back()
: Adds an element to the end of the vector.
myVector.push_back(42);
emplace_back()
: Constructs an element in place at the end of the vector (allows you to avoid unnecessary copies or moves).
myVector.emplace_back(42);
4. Access elements:
You can access vector elements using indexing (like arrays) or using iterators:
- Using indexing:
int element = myVector[0];
- Using iterators (preferred when iterating through the vector):
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
int element = *it;
}
5. Size and capacity:
size()
: Returns the number of elements in the vector.
int size = myVector.size();
capacity()
: Returns the current storage capacity of the vector (how many elements it can hold before resizing).
int capacity = myVector.capacity();
6. Remove elements:
You can remove elements from the vector using methods like pop_back()
or erase()
.
pop_back()
: Removes the last element from the vector.
myVector.pop_back();
erase()
: Removes one or more elements by specifying an iterator or a range.
myVector.erase(myVector.begin() + 2); // Removes the third element
7. Clear the vector:
You can remove all elements from the vector using the clear()
method:
myVector.clear();
8. Vector initialization:
You can initialize vectors with values using various constructors:
std::vector<int> numbers = {1, 2, 3, 4, 5};
9. Other operations:
Vectors support a wide range of operations, including sorting, searching, and more. You can use standard algorithms from the C++ Standard Library with vectors.
#include <algorithm>
// Sort the vector in ascending order
std::sort(myVector.begin(), myVector.end());
Vectors in C++ provide a flexible and convenient way to manage collections of data. They automatically handle memory allocation and resizing, making them a popular choice for dynamic arrays in C++ programs.