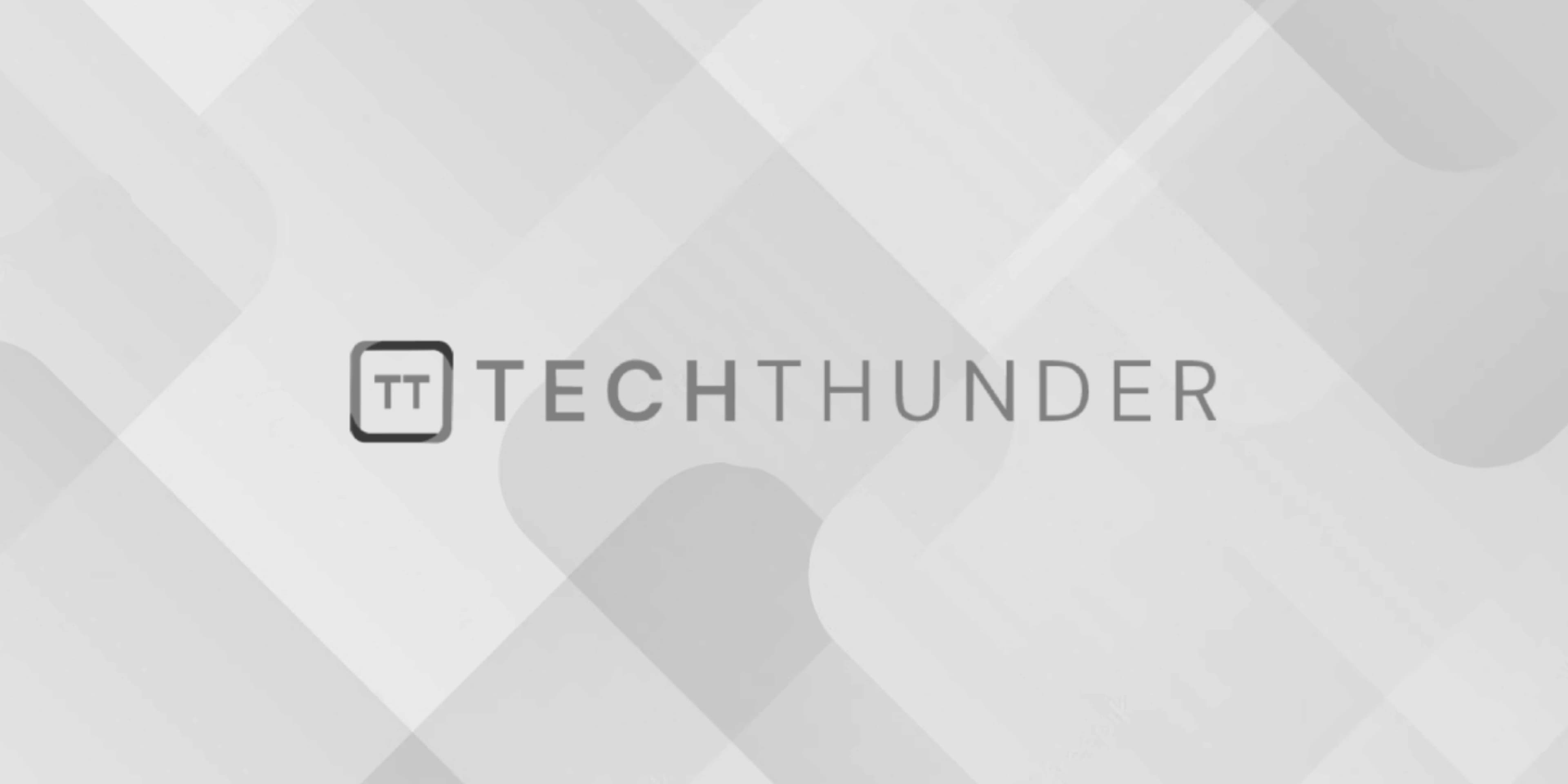
355 views
Boolean to String in C++
The C++ can convert a boolean value to a string using several methods. Here are a few common ways to convert a boolean to a string:
- Using a Conditional Operator:
C++
#include <iostream>
#include <string>
int main() {
bool flag = true;
// Convert a boolean to a string using a conditional operator
std::string result = flag ? "true" : "false";
std::cout << "Boolean as string: " << result << std::endl;
return 0;
}
In this example, we use a conditional (ternary) operator to check the value of the boolean variable flag
and assign the appropriate string representation to the result
variable.
- Using a Simple If-Else Statement:
C++
#include <iostream>
#include <string>
int main() {
bool flag = false;
std::string result;
// Convert a boolean to a string using an if-else statement
if (flag) {
result = "true";
} else {
result = "false";
}
std::cout << "Boolean as string: " << result << std::endl;
return 0;
}
Here, we use a simple if-else statement to check the value of flag
and assign the appropriate string representation to the result
variable.
- Using the
std::to_string
Function:
C++
#include <iostream>
#include <string>
int main() {
bool flag = true;
// Convert a boolean to a string using std::to_string
std::string result = std::to_string(flag);
std::cout << "Boolean as string: " << result << std::endl;
return 0;
}
The std::to_string
function is a convenient way to convert various types, including boolean values, to strings.
All of these methods will allow you to convert a boolean value to its string representation in C++. Choose the method that fits your coding style and requirements best.