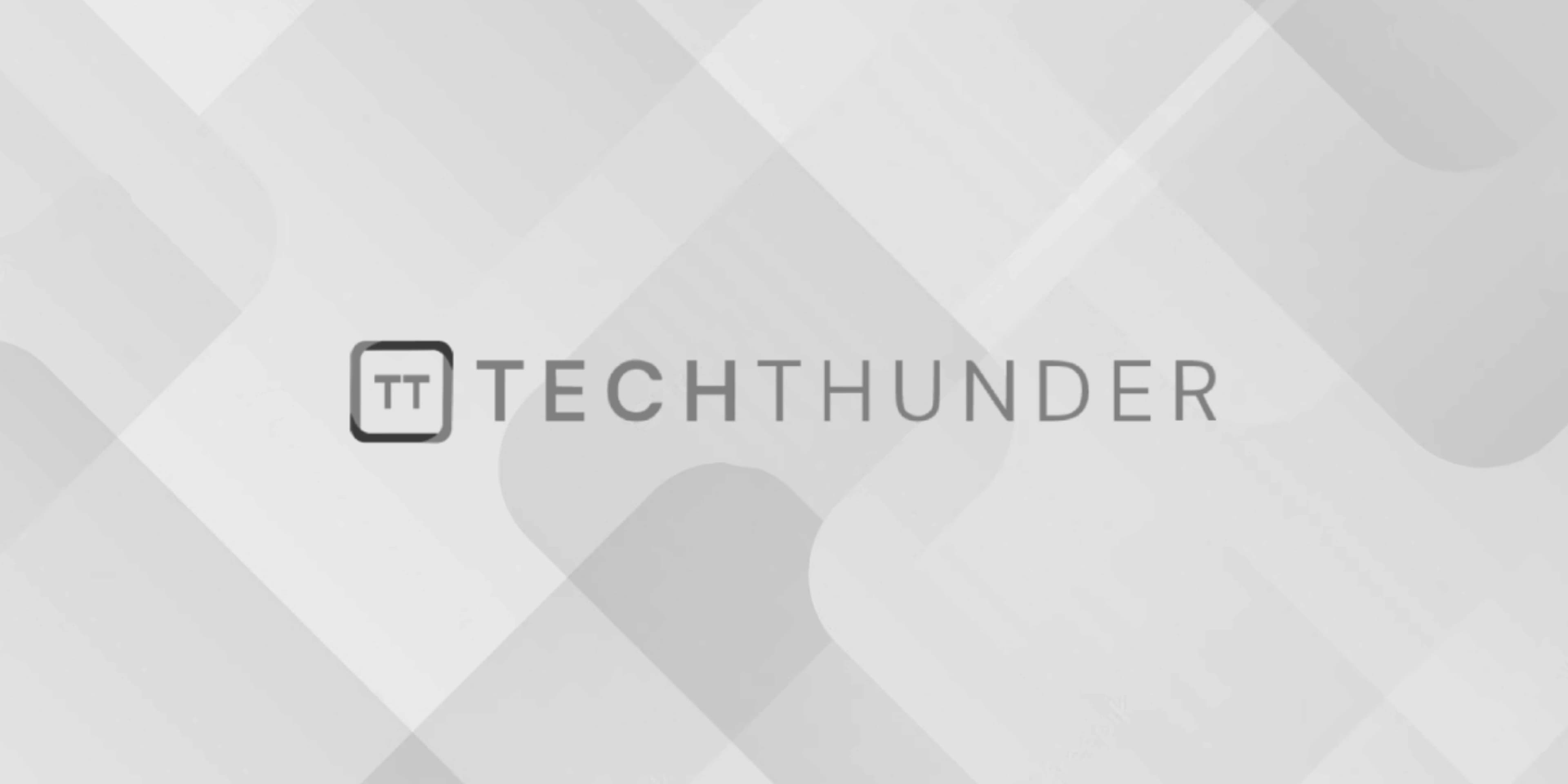
111 views
How does void* differ in C++
The C++ void*
is a special pointer type that can hold the address of an object of any data type but without knowing its type at compile time. It is a type of “generic” pointer that doesn’t have any specific type information associated with it until it’s explicitly cast to the correct type. Here are some key differences and characteristics of void*
in C++:
- Type-agnostic:
void*
can point to objects of any data type, including fundamental types (integers, floats, etc.), user-defined types (classes, structs), and even functions. - No Type Information:
void*
does not store any type information. It doesn’t know the size or layout of the data it points to. - Explicit Casting: To use the data pointed to by a
void*
, you need to cast it to the correct type explicitly. This casting is often referred to as “type casting” or “type conversion.”
C++
int x = 42;
void* ptr = &x;
int* intPtr = static_cast<int*>(ptr); // Explicit cast to int*
- Common Use Cases:
- Memory Allocation:
void*
is often used with functions likemalloc()
ornew
to allocate memory for objects of unknown types. - Passing Data to Generic Functions: When you want to pass data of different types to a generic function, you can use
void*
. However, this should be done with caution to avoid type-related errors. - Interoperability:
void*
can be used for interoperation with external libraries or code that expects a generic pointer type.
- Type Safety: Because
void*
lacks type information, it can lead to type-related errors if not used carefully. Casting it to the wrong type can result in undefined behavior and memory corruption. - Pointer Arithmetic: You cannot perform pointer arithmetic (e.g., increment, decrement) directly on a
void*
. To manipulate the address it points to, you need to cast it to an appropriate pointer type first.
C++
void* ptr = /* some address */;
// This is not allowed: ptr++;
char* charPtr = static_cast<char*>(ptr);
charPtr++; // Incrementing char* is allowed
- No Dereferencing: You cannot dereference a
void*
directly. To access the data it points to, you must cast it to the correct pointer type first.
C++
void* ptr = /* some address */;
// This is not allowed: int value = *ptr;
int* intPtr = static_cast<int*>(ptr);
int value = *intPtr; // Access the data after casting
In summary, void*
is a type-agnostic pointer that allows you to work with data of different types but requires careful handling through explicit casting. It is a powerful tool for certain tasks, but misuse or improper type casting can lead to errors and undefined behavior, so it should be used judiciously.