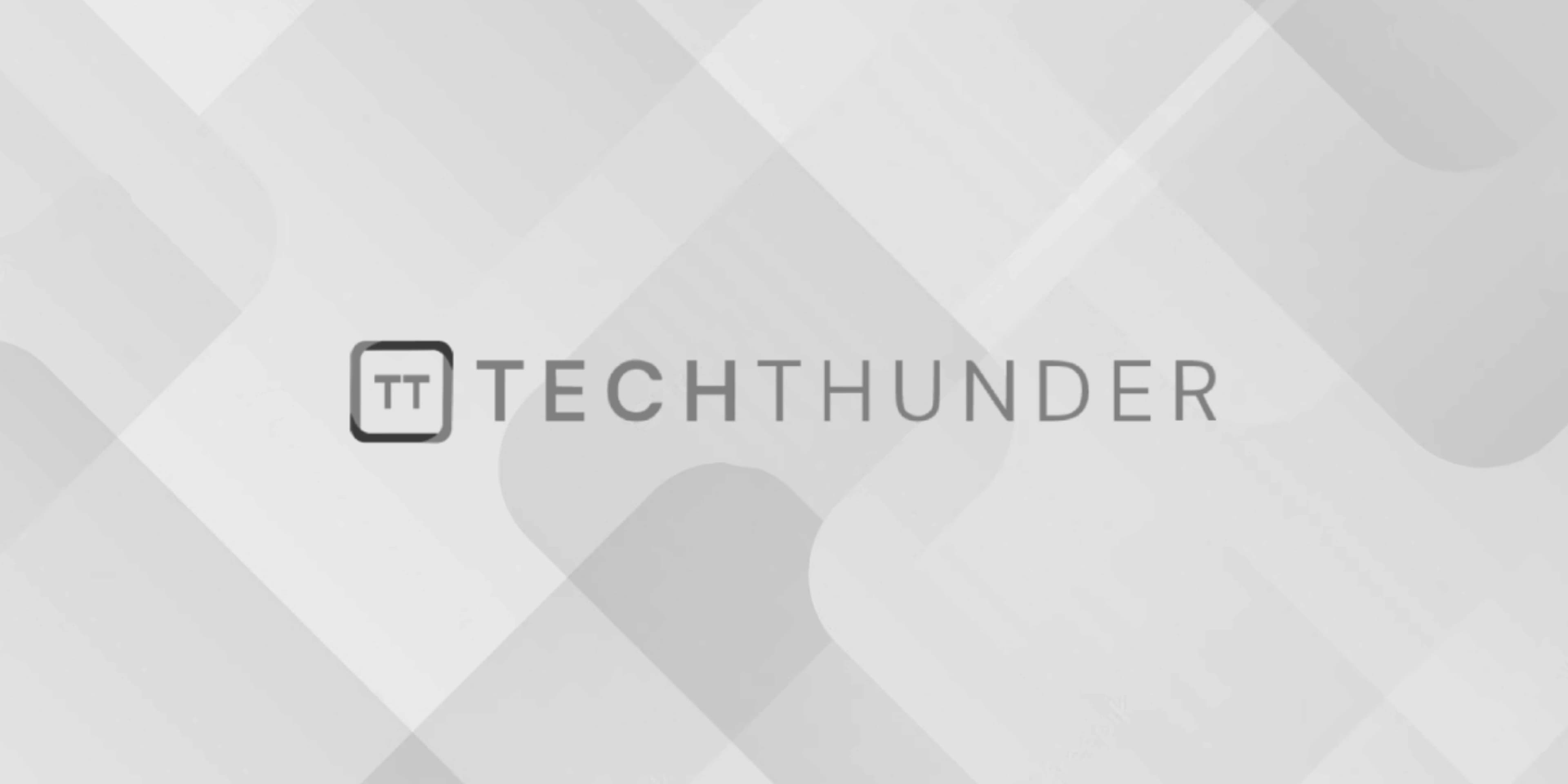
308 views
C++ Storage Classes
The C++ storage classes determine the scope, lifetime, and visibility of variables and functions within a program. C++ provides several storage classes that allow you to control how variables and functions are stored and accessed. The main storage classes in C++ are:
- Auto (Not Recommended): The
auto
storage class is rarely used in modern C++. It automatically deduces the type of a variable based on its initializer. Its usage is overshadowed by type inference provided byauto
in C++11 and later.
C++
auto x = 42; // Inferred as an integer
- Register (Obsolete): The
register
storage class suggests that a variable should be stored in a CPU register for faster access. However, this suggestion is rarely followed by modern compilers, and usingregister
has no significant impact on performance.
C++
register int count = 0;
- Static: Variables declared with the
static
storage class have a lifespan that extends throughout the program’s execution. They retain their values between function calls and have internal linkage by default, meaning they are only accessible within the translation unit (source file).
C++
static int counter = 0; // Static variable
- Extern: The
extern
storage class is used to declare a variable or function that is defined in another translation unit (source file). It allows you to use variables or functions from other files.
C++
extern int globalVar; // Declaration of an external variable
- Thread Local Storage (C++11 and later): The
thread_local
keyword is used to create thread-local storage. Variables declared asthread_local
have a separate instance for each thread in a multi-threaded program.
C++
thread_local int threadVar = 0; // Thread-local variable (C++11 and later)
- Mutable (Member Variables): In the context of class member variables, the
mutable
keyword allows a member variable to be modified even in aconst
member function of the class.
C++
class MyClass {
public:
mutable int counter; // Mutable member variable
void constFunction() const {
counter++; // Modifying a mutable variable in a const function is allowed
}
};
- Constexpr (C++11 and later): The
constexpr
keyword is used to declare variables and functions as compile-time constants. Variables declared asconstexpr
must be initialized with constant expressions, and they have a lifetime that extends for the entire program execution.
C++
constexpr int constValue = 42; // Compile-time constant (C++11 and later)
These storage classes help you control variable and function visibility, lifetime, and behavior in different parts of your program. Depending on your programming requirements, you may use one or more of these storage classes to achieve the desired behavior for your variables and functions.