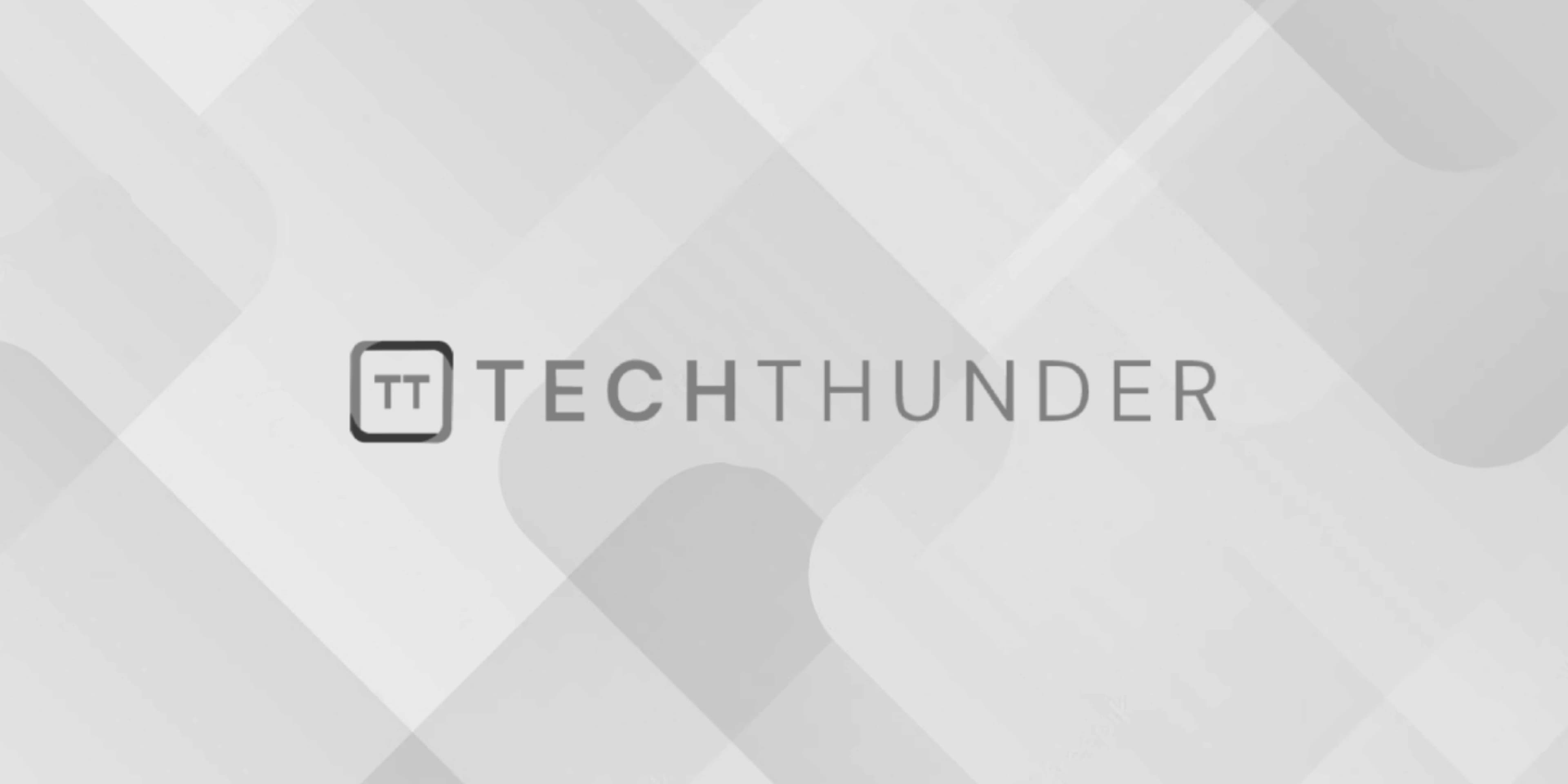
206 views
List back() function in C++ STL
The C++ STL (Standard Template Library), the back()
function is a member function of the std::list
container. It is used to access the last element of a linked list (doubly linked list) container.
Here’s how you can use the back()
function:
C++
#include <iostream>
#include <list>
int main() {
std::list<int> myList;
// Adding elements to the list
myList.push_back(1);
myList.push_back(2);
myList.push_back(3);
// Accessing the last element using back()
int lastElement = myList.back();
std::cout << "Last element of the list: " << lastElement << std::endl;
return 0;
}
In this example:
- We include the
<list>
header to use thestd::list
container. - We create a
std::list<int>
calledmyList
and add elements to it using thepush_back()
function. - To access the last element of the list, we use the
back()
function, which returns a reference to the last element. - Finally, we print the last element to the standard output.
Keep in mind that back()
assumes that the list is not empty. If the list is empty and you attempt to call back()
, it will result in undefined behavior. Therefore, it’s a good practice to check if the list is empty before using back()
, especially in production code. You can use the empty()
function to check if the list is empty.