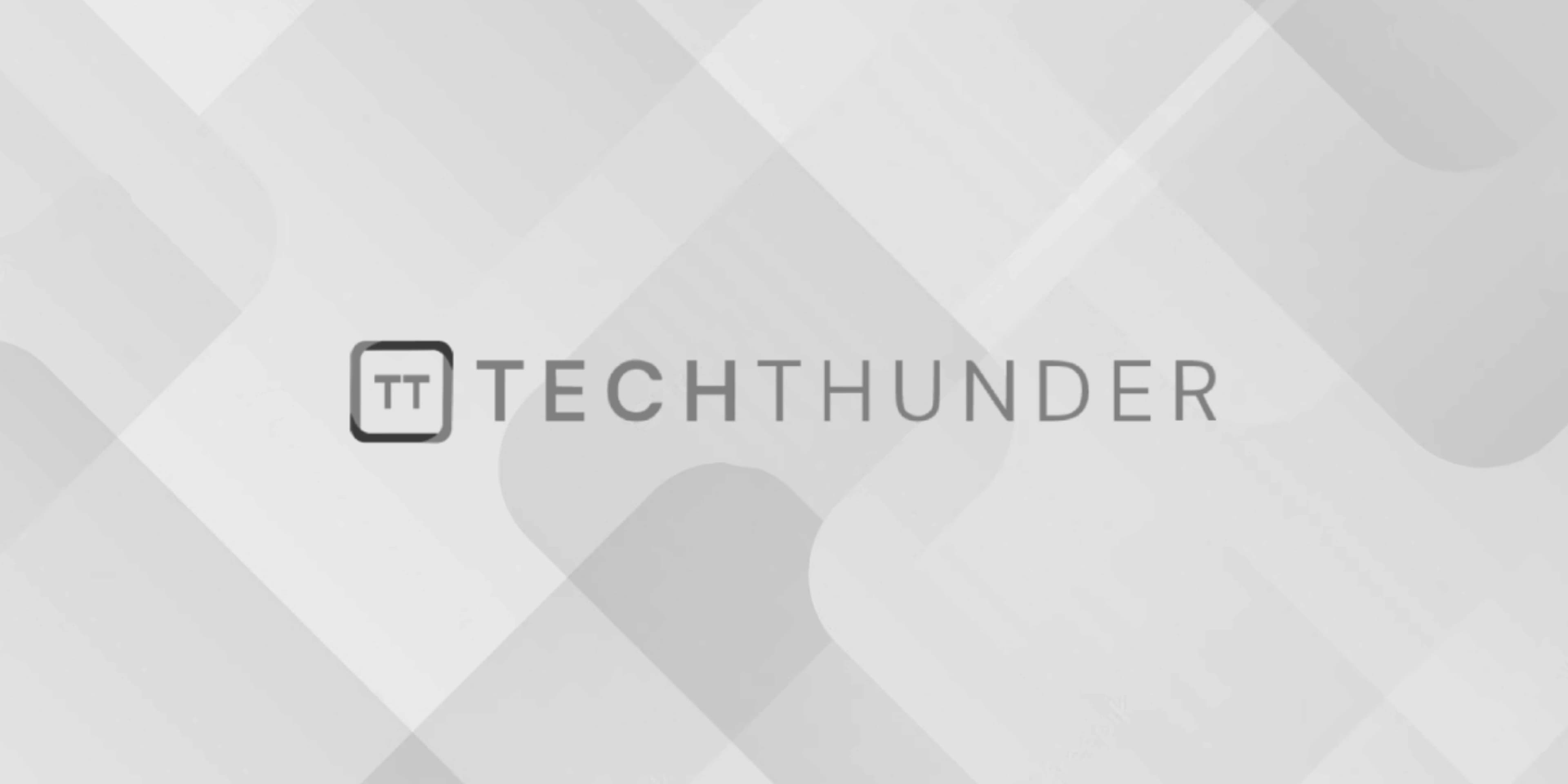
261 views
malloc() vs new in C++
The malloc()
and new
are both used in C++ to allocate memory dynamically, but they are different in several important ways:
- Origin:
malloc()
: It is a standard C library function. It’s also available in C++, but it’s not specific to C++.new
: It is a C++ operator designed for dynamic memory allocation. It’s part of the C++ language itself.
- Return Type:
malloc()
: It returns avoid*
pointer, which must be cast to the appropriate type when used.new
: It returns a pointer to the allocated type, and you don’t need to cast it.
- Initialization:
malloc()
: It does not call constructors for classes. The allocated memory contains raw, uninitialized data.new
: It calls the constructor for classes, ensuring proper initialization.
- Memory Size Calculation:
malloc()
: You must manually specify the number of bytes to allocate.new
: You specify the type you want to allocate, and the compiler calculates the size based on the type.
- Failure Handling:
malloc()
: It returns a null pointer (nullptr
in C++) when it fails to allocate memory.new
: It throws an exception of typestd::bad_alloc
when it fails to allocate memory. You can also use thenothrow
version ofnew
to make it returnnullptr
on failure.
Here’s a comparison of how you would use malloc()
and new
to allocate memory for an integer:
Using malloc()
:
C++
int* ptr = (int*)malloc(sizeof(int));
if (ptr == nullptr) {
// Handle memory allocation failure
} else {
*ptr = 42;
// ...
free(ptr); // Don't forget to free the memory when done
}
Using new
:
C++
int* ptr = new int;
if (ptr == nullptr) {
// Handle memory allocation failure (unlikely due to exception)
} else {
*ptr = 42;
// No need to explicitly free memory; it's automatically done when the variable goes out of scope or you use delete
}
It’s generally recommended to use new
(or better yet, smart pointers like std::shared_ptr
or std::unique_ptr
) when working with classes and objects, as it ensures proper initialization and automatic memory management. However, for low-level memory manipulation or compatibility with C code, you might still encounter malloc()
and free()
. Just be cautious when mixing them, as they have different behaviors.