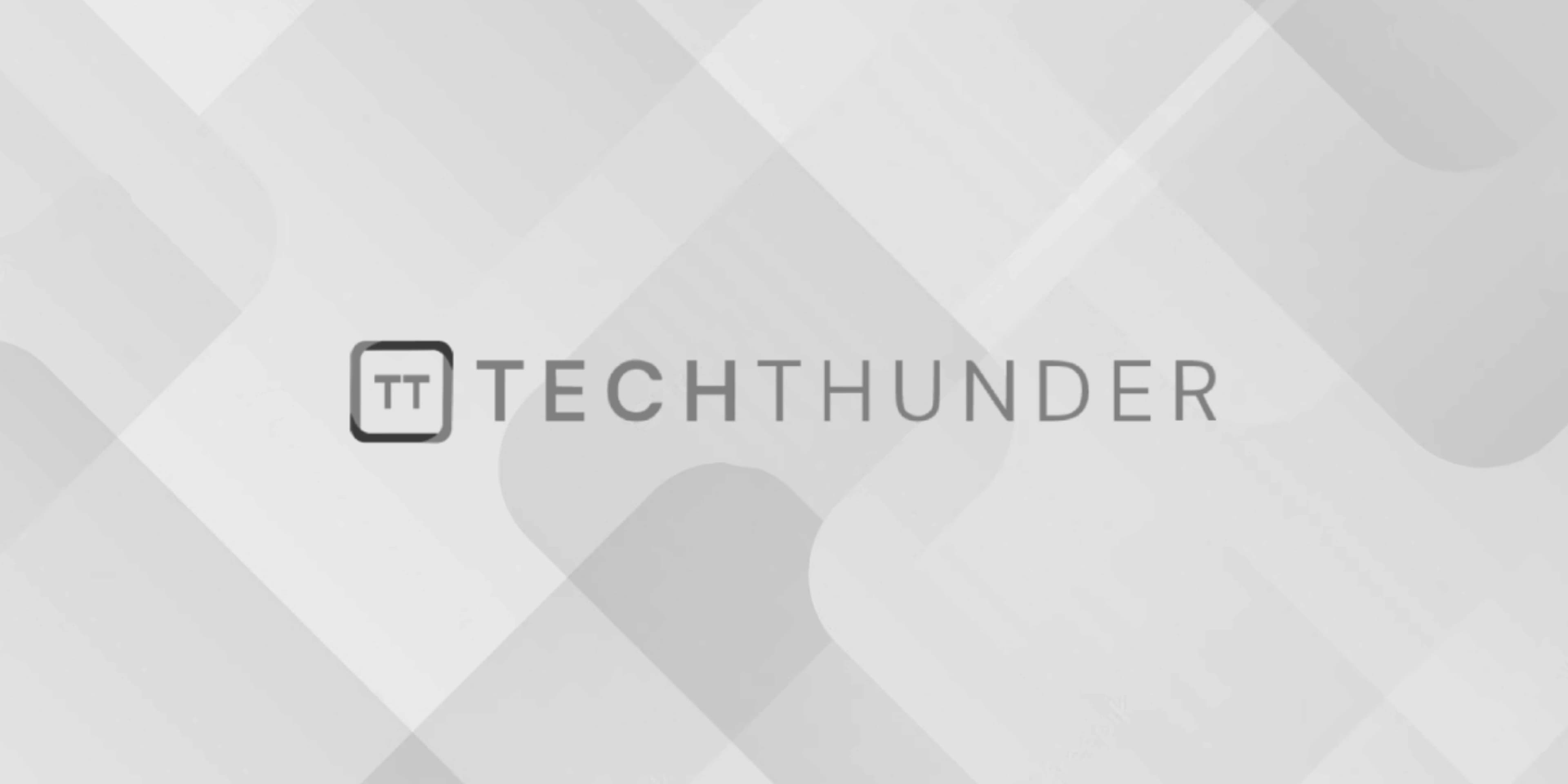
258 views
Boost::split in C++
The C++ use boost::split
function from the Boost C++ Libraries to split a string into substrings based on a specified delimiter. To use this function, make sure you have the Boost Libraries installed and included in your project. Here’s how to use boost::split
:
C++
#include <iostream>
#include <vector>
#include <string>
#include <boost/algorithm/string.hpp>
int main() {
std::string input_string = "apple,banana,cherry,grape";
std::vector<std::string> tokens;
// Use boost::split to split the input string by comma (',') delimiter
boost::split(tokens, input_string, boost::is_any_of(","));
// Iterate over the resulting tokens and print them
for (const std::string& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
In this example:
- Include the necessary headers:
<iostream>
,<vector>
,<string>
, and<boost/algorithm/string.hpp>
. - Create a
std::string
namedinput_string
that contains the string you want to split. - Create a
std::vector<std::string>
namedtokens
to store the resulting substrings. - Use
boost::split
to split theinput_string
into substrings based on the comma (‘,’) delimiter. Theboost::is_any_of(",")
function specifies the delimiter. - Iterate over the
tokens
vector to access and print each of the resulting substrings.
Remember to install and link the Boost Libraries to your project, as they are not part of the C++ Standard Library. You might also need to set up your development environment to correctly find the Boost libraries if they are not in standard library directories.