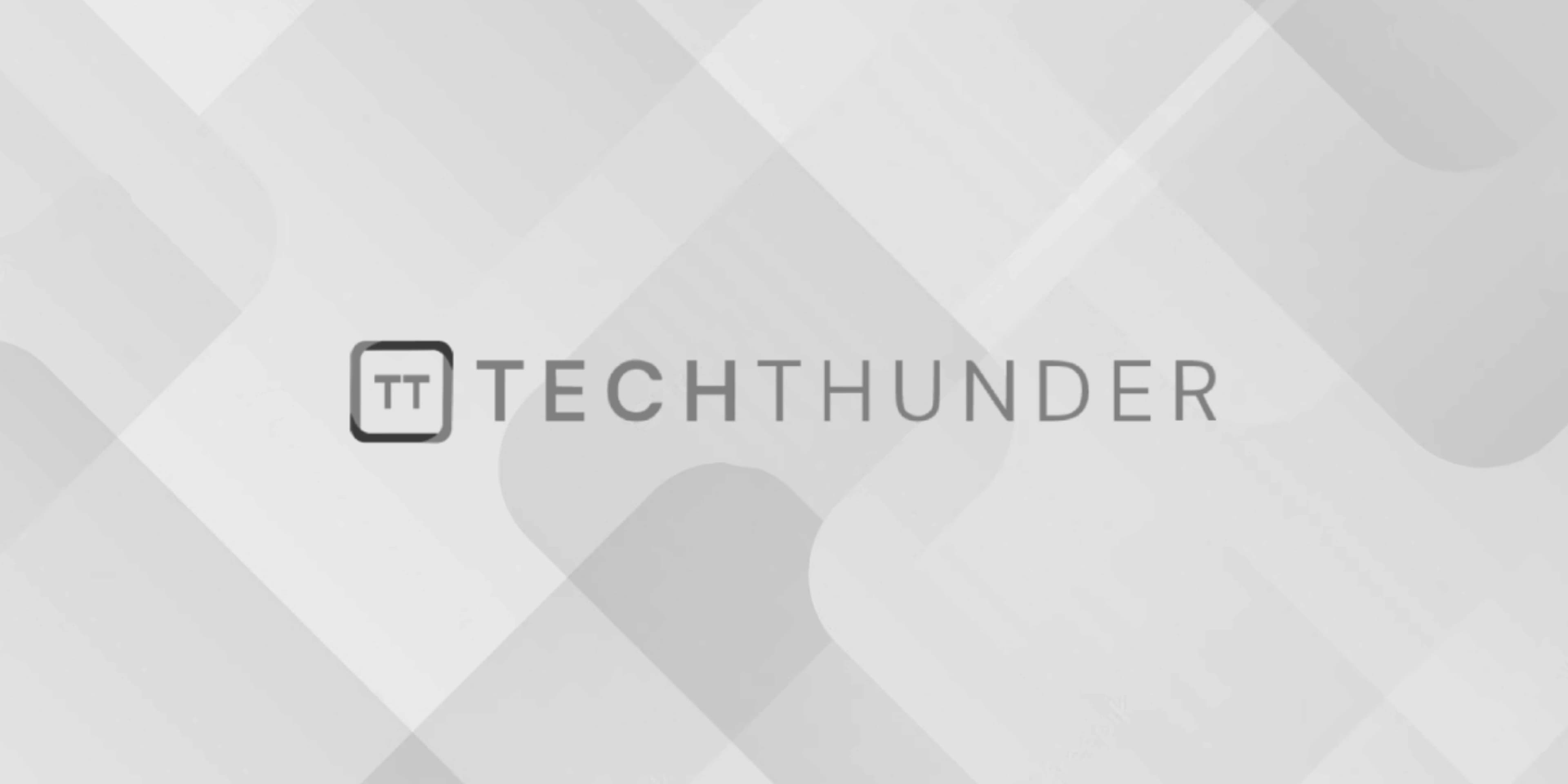
C++ Deque
The C++ has deque
(pronounced “deck”) stands for “double-ended queue.” It is a data structure that supports insertion and deletion of elements from both ends efficiently. The std::deque
is provided by the C++ Standard Library and is part of the C++ Standard Template Library (STL).
Here’s how you can work with a deque
in C++:
Including the Required Header:
You need to include the <deque>
header to use the std::deque
container.
#include <iostream>
#include <deque>
Declaring a deque
:
You can declare a deque
like this:
std::deque<int> myDeque;
This declares a deque named myDeque
that can store integers.
Adding Elements:
You can add elements to the front or back of the deque
using push_front
and push_back
functions, respectively:
myDeque.push_back(42); // Adds 42 to the back of the deque
myDeque.push_front(17); // Adds 17 to the front of the deque
Removing Elements:
You can remove elements from the front or back of the deque
using pop_front
and pop_back
functions:
myDeque.pop_back(); // Removes the last element
myDeque.pop_front(); // Removes the first element
Accessing Elements:
You can access elements in a deque
by index, just like an array:
int value = myDeque[2]; // Accesses the element at index 2
Checking Size:
You can check the size of the deque
using the size()
function:
int size = myDeque.size();
Iterating Over a deque
:
You can iterate through the elements of a deque
using iterators or range-based for loops:
for (auto it = myDeque.begin(); it != myDeque.end(); ++it) {
std::cout << *it << " ";
}
// or using a range-based for loop
for (const int& element : myDeque) {
std::cout << element << " ";
}
std::deque
provides efficient insertion and deletion at both ends compared to std::vector
, which provides efficient operations at the back but not at the front. Therefore, if you need a container that supports these operations efficiently, std::deque
is a good choice.