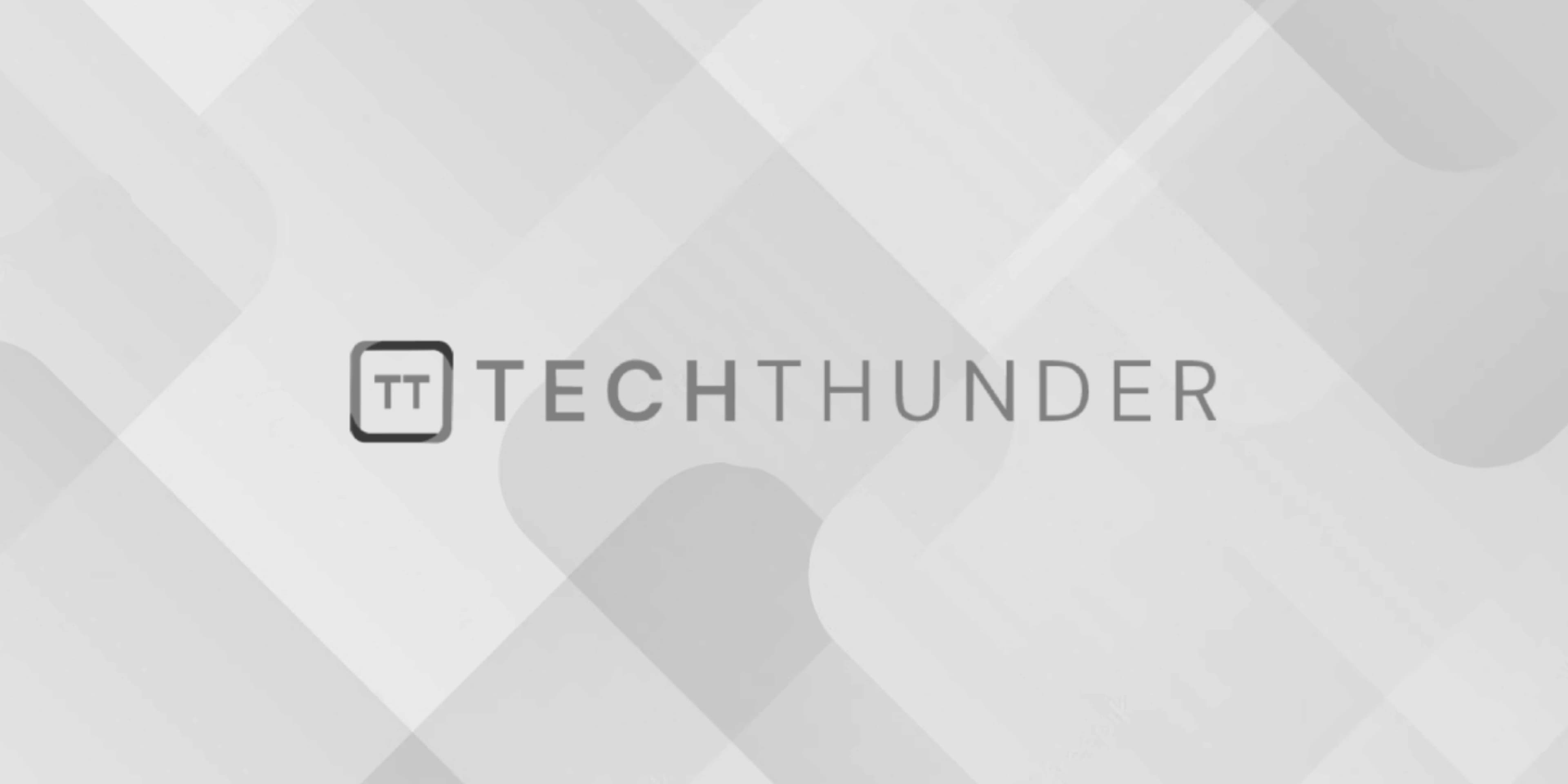
162 views
Static Object in C++
The C++ static object is an instance of a class or variable that is associated with the class itself rather than with a specific instance of the class. Static objects have a few distinctive characteristics:
- Static Member Variables: A common use of static objects in C++ is as static member variables of a class. Static member variables are shared among all instances of the class, and there is only one copy of the static member variable in memory.
- Static Member Functions: Static objects can also be static member functions of a class. Static member functions do not operate on specific instances of the class and can be called using the class name, not an object instance.
Here’s how to define and use static member variables and functions in C++:
C++
#include <iostream>
class MyClass {
public:
// Static member variable shared among all instances of MyClass
static int staticVar;
// Static member function that doesn't operate on instances
static void staticFunction() {
std::cout << "This is a static function." << std::endl;
}
// Regular member function
void regularFunction() {
std::cout << "This is a regular function." << std::endl;
}
};
// Initialize the static member variable
int MyClass::staticVar = 0;
int main() {
// Create instances of MyClass
MyClass obj1, obj2;
// Access the static member variable using the class name or an instance
MyClass::staticVar = 42;
std::cout << "Static variable value: " << MyClass::staticVar << std::endl;
obj1.staticVar = 24; // Also possible but not recommended
// Call the static member function using the class name or an instance
MyClass::staticFunction();
obj1.staticFunction();
// Call a regular member function using an instance
obj1.regularFunction();
return 0;
}
In this example:
staticVar
is a static member variable shared among all instances of theMyClass
class. It is initialized outside the class definition.staticFunction()
is a static member function that doesn’t operate on specific instances and can be called using the class name or an instance.regularFunction()
is a regular member function that operates on specific instances and can only be called using an instance of the class.
Static member variables are useful when you want to share data among all instances of a class or keep track of some global state within the class. Static member functions are often used for utility functions that don’t need access to instance-specific data.
Keep in mind that the use of static member variables should be carefully considered, as they can lead to global state and potential issues with thread safety.