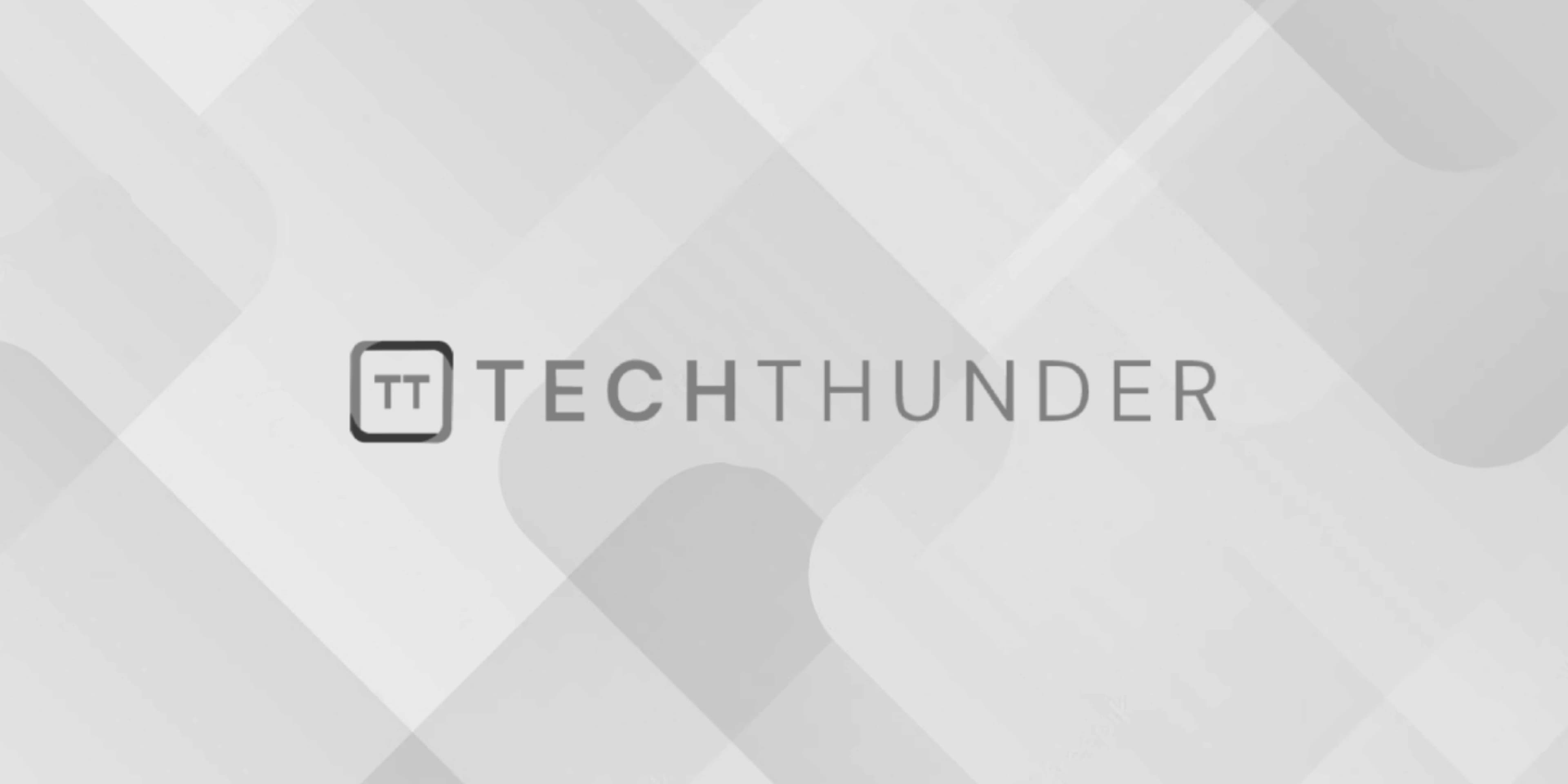
320 views
Arrays of Vectors in C++ STL
The C++ can create an array of vectors by using a vector to store vectors as its elements. This can be useful when you need a dynamic number of vectors, and each vector can have a different size. Here’s how you can create and use an array of vectors in C++ using the Standard Template Library (STL):
C++
#include <iostream>
#include <vector>
int main() {
const int arraySize = 3; // Size of the array of vectors
// Create an array of vectors
std::vector<int> arrayOfVectors[arraySize];
// Populate the vectors in the array
for (int i = 0; i < arraySize; ++i) {
for (int j = 0; j < i + 1; ++j) {
arrayOfVectors[i].push_back(i * 10 + j);
}
}
// Access and print elements from each vector
for (int i = 0; i < arraySize; ++i) {
std::cout << "Vector " << i << ": ";
for (int j = 0; j < arrayOfVectors[i].size(); ++j) {
std::cout << arrayOfVectors[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
In this example:
- We define
arraySize
to determine the number of vectors in the array. - We create an array
arrayOfVectors
of vectors. Each element ofarrayOfVectors
is a vector that can store integers. - We populate each vector in the array with some sample values using a nested loop.
- We access and print the elements from each vector in the array.
This demonstrates how you can create and work with an array of vectors in C++. Each vector in the array can have a different size, making it a flexible data structure for various applications.