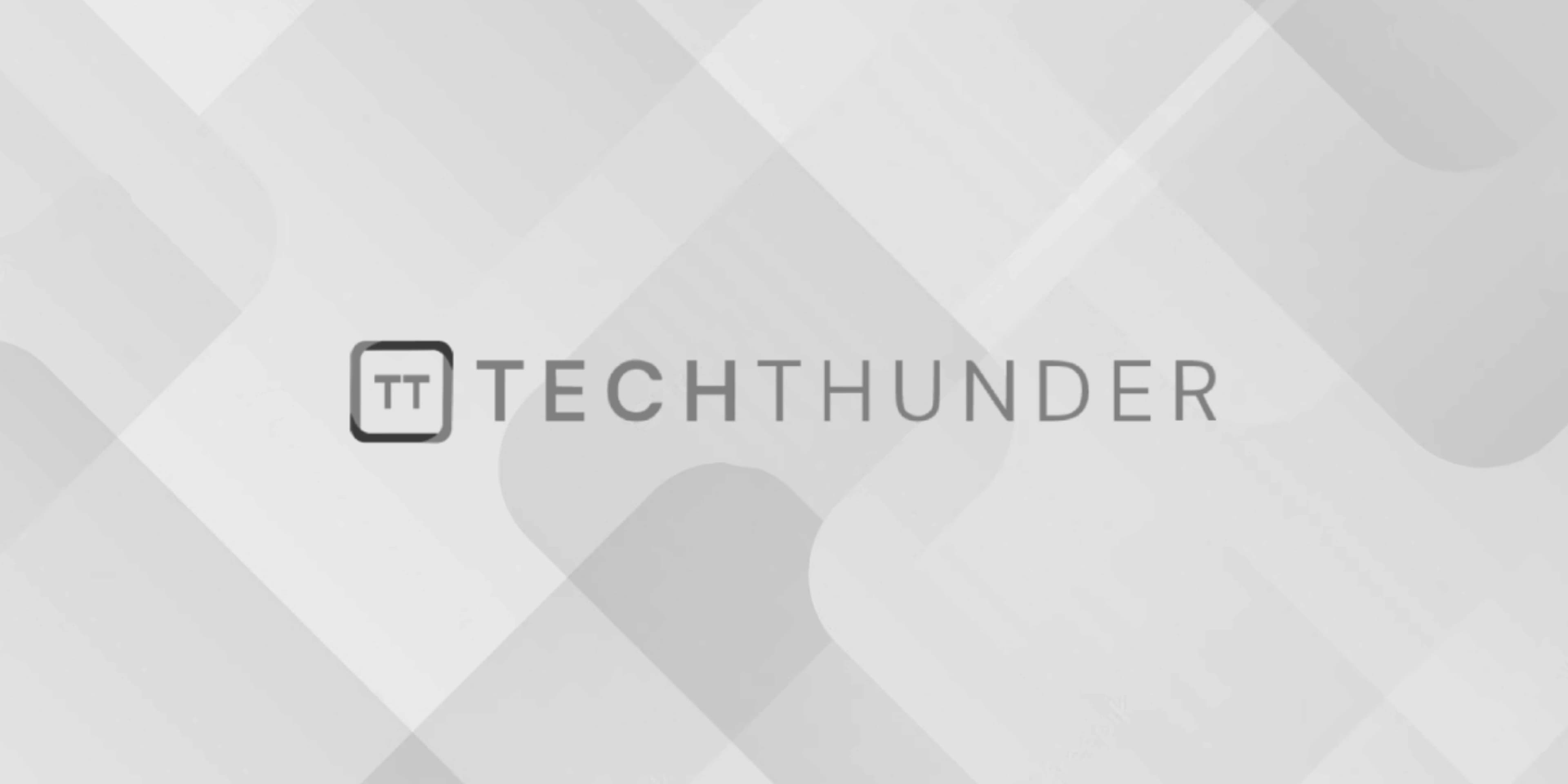
163 views
C++ Program to Draw Histogram
A histogram is a graphical representation of data that shows the distribution of values in a dataset. In this C++ program, I’ll provide a basic example of how to draw a histogram using asterisks (*) to represent the data. We’ll assume you have a dataset of values, and you want to visualize their distribution.
Here’s a simple C++ program to draw a histogram:
C++
#include <iostream>
#include <vector>
void drawHistogram(const std::vector<int>& data) {
// Find the maximum value in the dataset to determine the scale
int max_value = 0;
for (int value : data) {
if (value > max_value) {
max_value = value;
}
}
// Determine the number of asterisks per unit on the scale
double scale_factor = 40.0 / max_value;
// Draw the histogram
for (int i = 1; i <= max_value; i++) {
std::cout << i << ": ";
int asterisks_to_draw = static_cast<int>(data[i - 1] * scale_factor);
for (int j = 0; j < asterisks_to_draw; j++) {
std::cout << "*";
}
std::cout << std::endl;
}
}
int main() {
// Sample dataset (you can replace this with your own data)
std::vector<int> data = {5, 8, 12, 3, 15, 7, 10};
std::cout << "Histogram:" << std::endl;
drawHistogram(data);
return 0;
}
In this program:
- We define a
drawHistogram
function that takes a vector of integers (data
) representing your dataset. - We find the maximum value in the dataset to determine the scale for the histogram.
- We calculate a scale factor to determine how many asterisks should represent each unit on the scale. In this example, we use 40 asterisks as the maximum width of the histogram.
- We iterate through each value in the dataset, calculate the number of asterisks to draw for each value, and print the histogram using asterisks.
- In the
main
function, we provide a sample dataset (data
) and calldrawHistogram
to visualize it.
You can replace the data
vector with your own dataset to draw a histogram for your specific data.