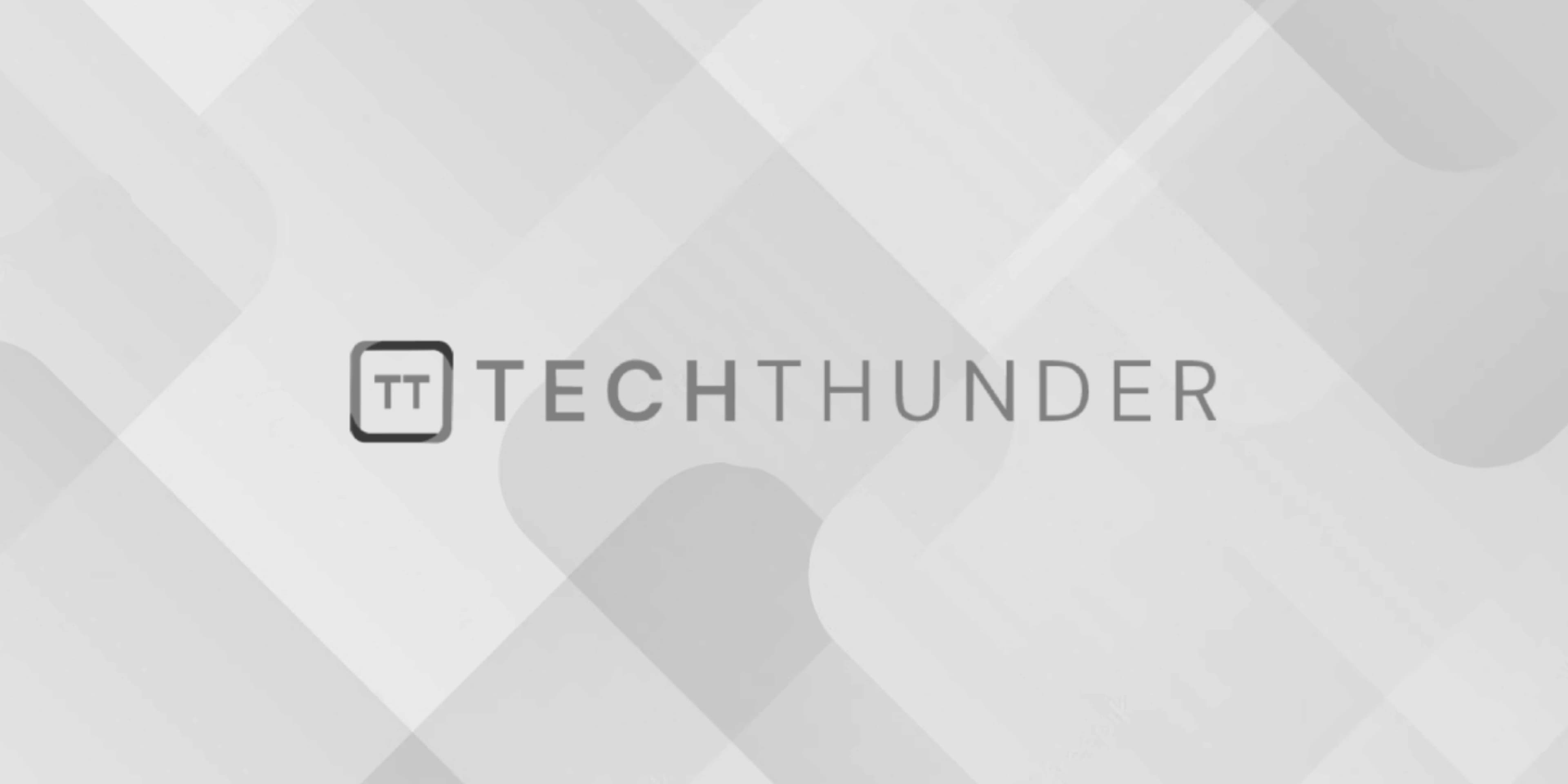
SJF CPU Scheduling Program in C++
Shortest Job First (SJF) is a CPU scheduling algorithm that selects the ready process with the smallest execution time next. In C++, you can create a simple SJF CPU scheduling program using arrays or data structures to represent processes and their attributes. Here’s an example using an array of structures:
#include <iostream>
#include <algorithm>
struct Process {
int id;
int burstTime;
};
// Function to perform SJF scheduling
void sjfScheduling(Process processes[], int n) {
// Sort processes by burst time in ascending order
std::sort(processes, processes + n, [](const Process& a, const Process& b) {
return a.burstTime < b.burstTime;
});
int currentTime = 0;
double totalWaitingTime = 0;
double totalTurnaroundTime = 0;
std::cout << "Process\tBurst Time\tWaiting Time\tTurnaround Time\n";
for (int i = 0; i < n; i++) {
totalWaitingTime += currentTime;
totalTurnaroundTime += currentTime + processes[i].burstTime;
std::cout << processes[i].id << "\t\t" << processes[i].burstTime << "\t\t" << currentTime << "\t\t" << (currentTime + processes[i].burstTime) << "\n";
currentTime += processes[i].burstTime;
}
double averageWaitingTime = totalWaitingTime / n;
double averageTurnaroundTime = totalTurnaroundTime / n;
std::cout << "\nAverage Waiting Time: " << averageWaitingTime << std::endl;
std::cout << "Average Turnaround Time: " << averageTurnaroundTime << std::endl;
}
int main() {
int n;
std::cout << "Enter the number of processes: ";
std::cin >> n;
Process processes[n];
for (int i = 0; i < n; i++) {
processes[i].id = i + 1;
std::cout << "Enter burst time for process " << processes[i].id << ": ";
std::cin >> processes[i].burstTime;
}
sjfScheduling(processes, n);
return 0;
}
This program defines a Process
structure to store the process ID and burst time. The sjfScheduling
function performs SJF scheduling by sorting the processes based on burst time and then calculating waiting and turnaround times. Finally, it prints the scheduling results and the average waiting and turnaround times.
Compile and run the program, and it will prompt you to enter the number of processes and their burst times. It will then display the scheduling results, including the average waiting and turnaround times.