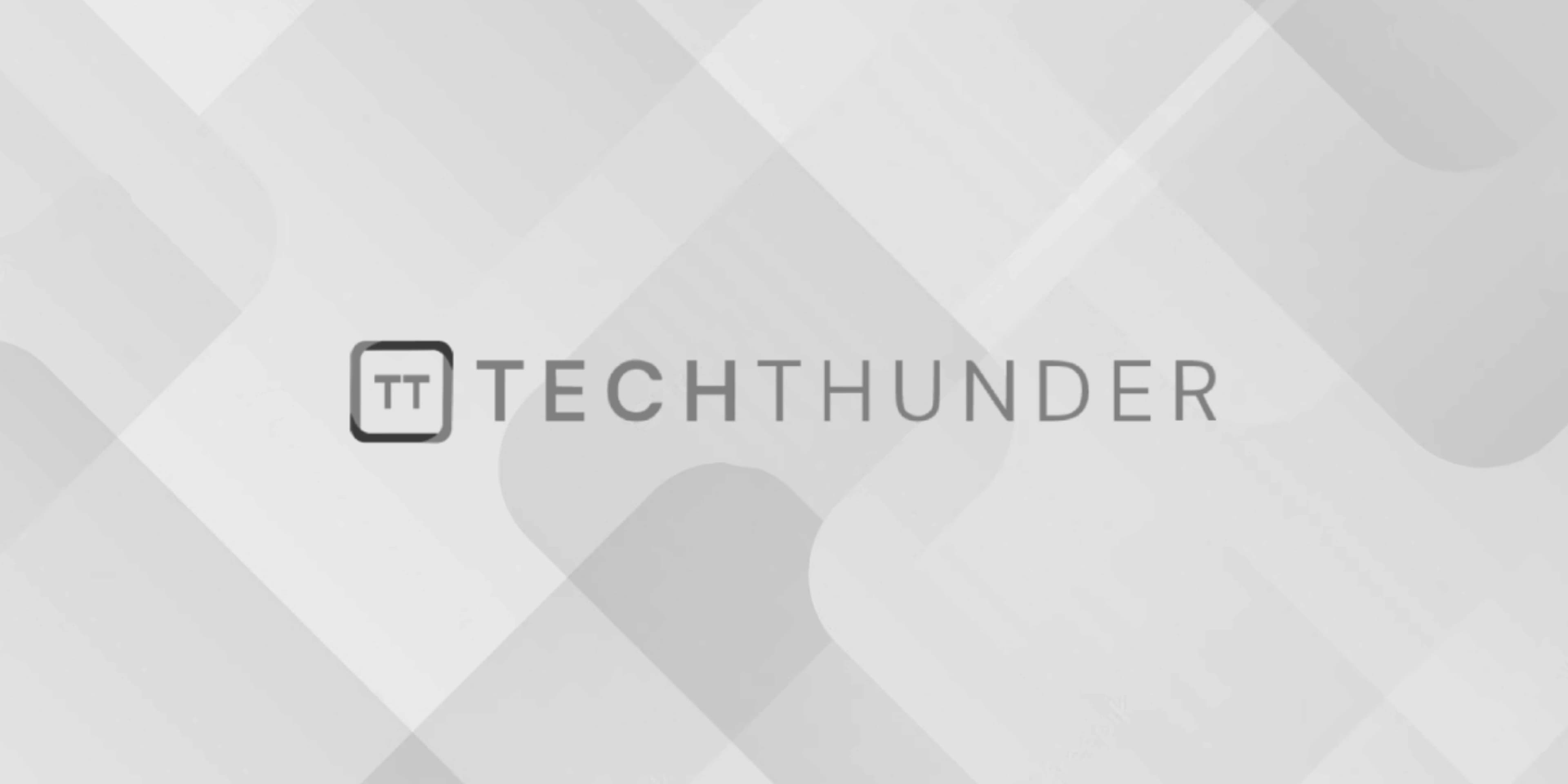
Acess Specifiers in C++
Access specifiers in C++ are keywords used to control the visibility and accessibility of class members (variables and functions) from outside the class. There are three main access specifiers in C++:
- Public:
- Members declared as public are accessible from anywhere in the program, both from within the class and from external code.
- Public members are part of the class’s public interface, and they are used to interact with objects of the class. Example:
class MyClass {
public:
int publicVar;
void publicFunction() {
// Code here
}
};
- Private:
- Members declared as private are only accessible from within the class itself.
- They are not visible or accessible from outside the class, including derived classes (unless friend functions or friend classes are used). Example:
class MyClass {
private:
int privateVar;
void privateFunction() {
// Code here
}
};
- Protected:
- Members declared as protected are accessible from within the class and by derived classes.
- They are not accessible from external code that is not part of the derived class. Example:
class Base {
protected:
int protectedVar;
void protectedFunction() {
// Code here
}
};
class Derived : public Base {
public:
void accessProtected() {
protectedVar = 42; // Access protected member from the derived class
protectedFunction(); // Access protected function from the derived class
}
};
Access specifiers allow you to control the encapsulation and data hiding in your classes. By making members private, you hide the internal implementation details from the outside world, providing a clear interface for users of the class. Public members define how the class can be interacted with, while protected members are used when creating base classes for inheritance hierarchies.
In practice, it’s a good practice to make data members private and provide public member functions (getters and setters) to access and modify them when necessary. This encapsulation helps maintain the integrity of the class and allows you to change the implementation details without affecting external code.