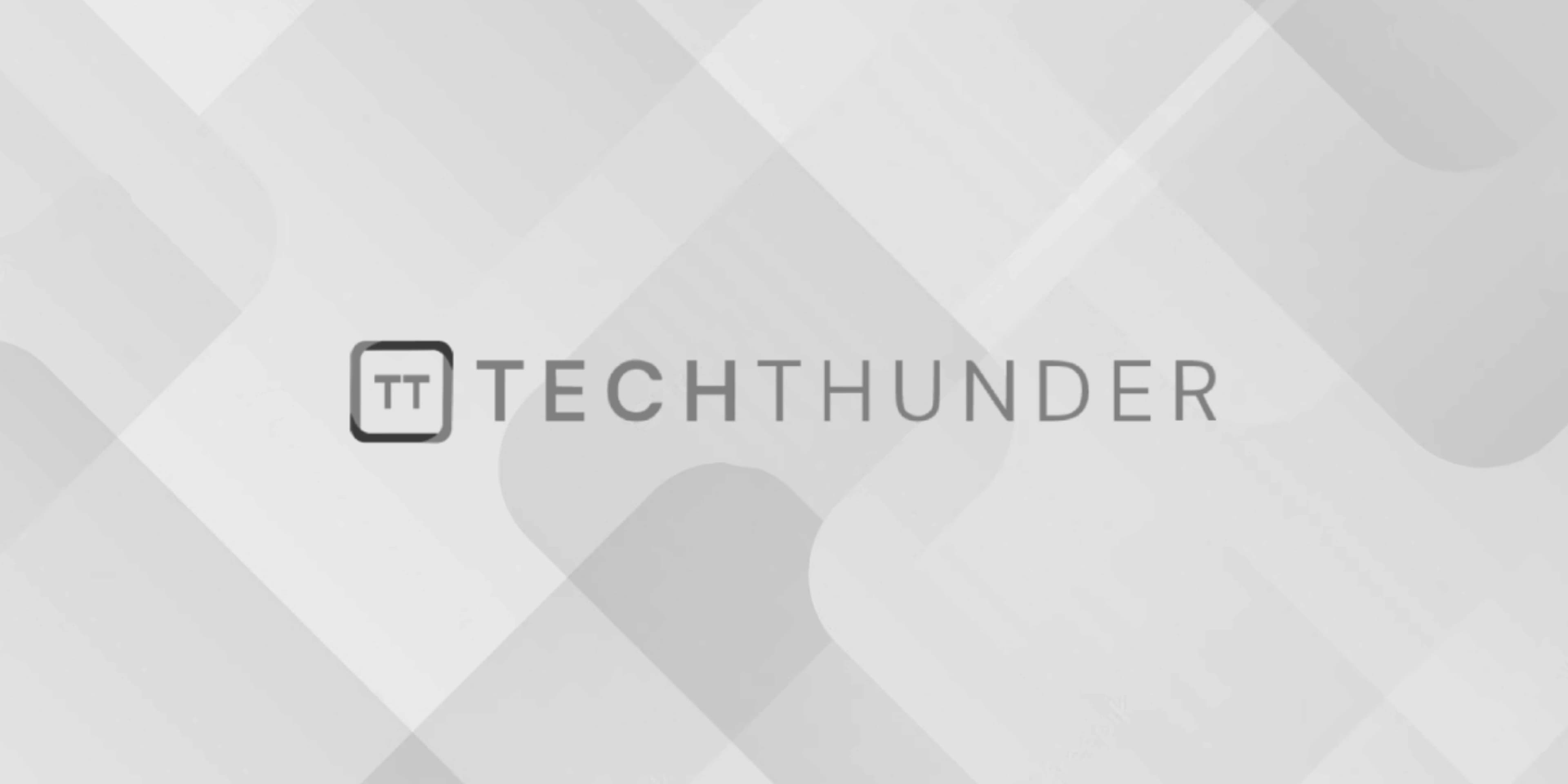
C++ Interfaces
The C++ is no direct concept of interfaces like you would find in languages such as Java or C#. However, you can achieve a similar form of interface-like behavior through abstract classes, pure virtual functions, and multiple inheritance. Here’s how you can create interfaces in C++:
- Abstract Classes with Pure Virtual Functions: An abstract class is a class that cannot be instantiated directly and is typically used as a base class for other classes. It may contain one or more pure virtual functions, which are declared with the
virtual
keyword and have no implementation. Pure virtual functions provide a blueprint for derived classes to implement.
class Shape {
public:
virtual double area() const = 0; // Pure virtual function
virtual double perimeter() const = 0; // Pure virtual function
};
Derived classes that inherit from the Shape
class must provide concrete implementations for the area
and perimeter
functions:
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) { }
double area() const override {
return 3.14159265358979323846 * radius * radius;
}
double perimeter() const override {
return 2 * 3.14159265358979323846 * radius;
}
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) { }
double area() const override {
return width * height;
}
double perimeter() const override {
return 2 * (width + height);
}
};
- Multiple Inheritance: C++ supports multiple inheritance, allowing a class to inherit from multiple base classes. You can use this feature to achieve interface-like behavior by creating classes that inherit from multiple classes.
class Flyable {
public:
virtual void fly() = 0;
};
class Swimmable {
public:
virtual void swim() = 0;
};
class Bird : public Flyable {
public:
void fly() override {
// Implementation for flying
}
};
class Fish : public Swimmable {
public:
void swim() override {
// Implementation for swimming
}
};
class Duck : public Flyable, public Swimmable {
public:
void fly() override {
// Implementation for flying
}
void swim() override {
// Implementation for swimming
}
};
In this example, Bird
and Fish
classes implement the Flyable
and Swimmable
interfaces, respectively. The Duck
class inherits from both Flyable
and Swimmable
, and it must provide implementations for both fly
and swim
.
Remember that C++ allows you to define multiple interfaces via base classes and pure virtual functions, but it doesn’t enforce the separation of interface and implementation as strictly as some other languages. Therefore, it’s up to the programmer to adhere to the intended interface behavior.