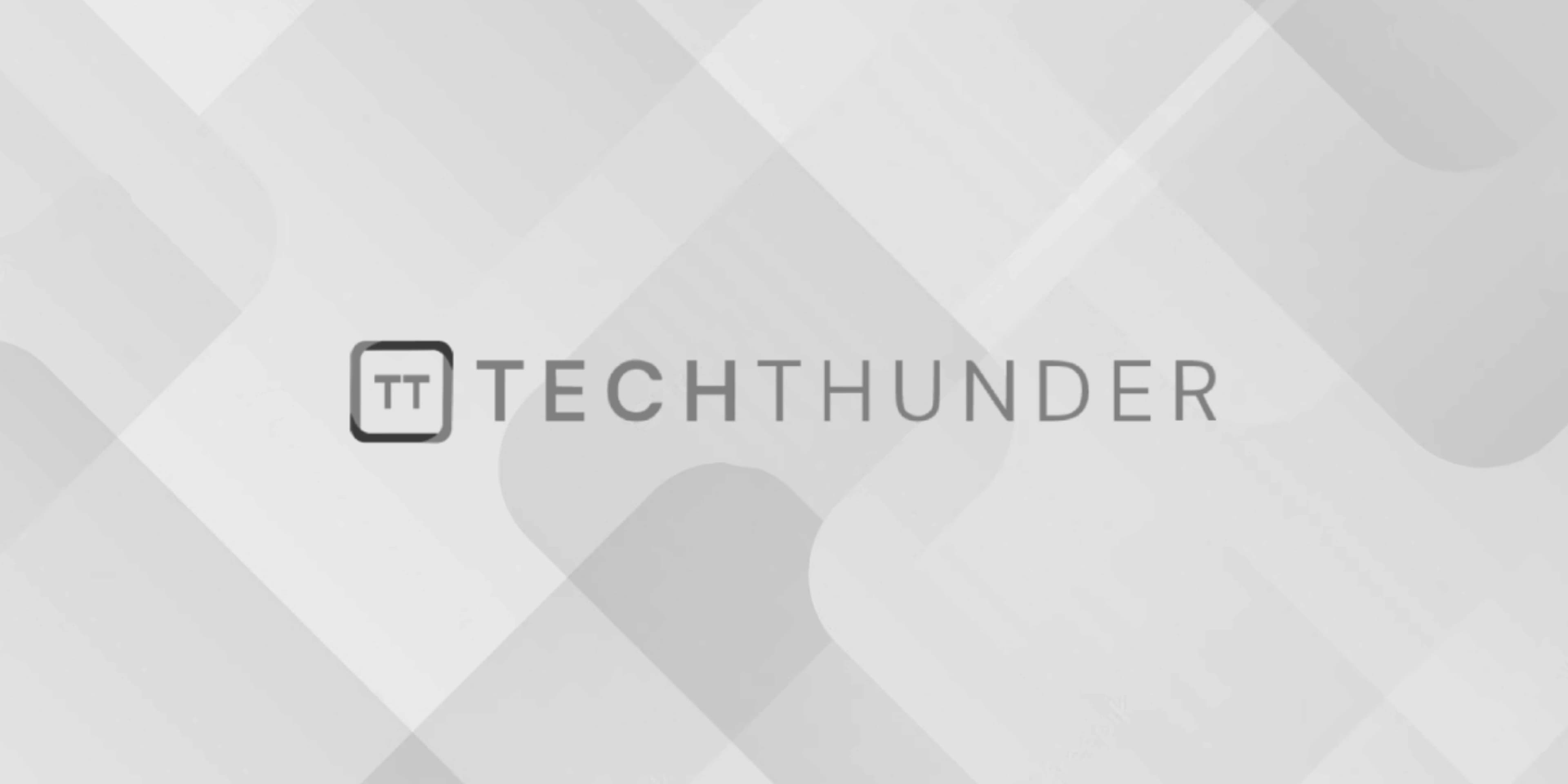
Factory Design Pattern C++
The Factory Design Pattern is a creational design pattern in C++ (and other programming languages) that provides an interface for creating objects in a super class, but allows subclasses to alter the type of objects that will be created. This pattern is useful when you want to create objects without specifying the exact class of object that will be created at runtime.
Here’s how the Factory Design Pattern works in C++:
- Define an Abstract Base Class: Create an abstract base class that declares a pure virtual method for creating objects. This method will be overridden by concrete subclasses.
- Create Concrete Subclasses: Create concrete subclasses that inherit from the abstract base class. Each subclass implements the factory method to create specific instances of objects.
- Factory Class: Create a factory class (often called the “factory” or “creator” class) that contains a method for creating objects of the abstract base class. This method internally uses the factory methods of the concrete subclasses to create objects.
- Client Code: The client code uses the factory class to create objects without needing to know the specific implementation classes.
Here’s a simple example of the Factory Design Pattern in C++:
#include <iostream>
// Abstract base class
class Product {
public:
virtual void operation() = 0;
};
// Concrete product classes
class ConcreteProductA : public Product {
public:
void operation() override {
std::cout << "ConcreteProductA operation." << std::endl;
}
};
class ConcreteProductB : public Product {
public:
void operation() override {
std::cout << "ConcreteProductB operation." << std::endl;
}
};
// Factory class
class Factory {
public:
virtual Product* createProduct() = 0;
};
class ConcreteFactoryA : public Factory {
public:
Product* createProduct() override {
return new ConcreteProductA();
}
};
class ConcreteFactoryB : public Factory {
public:
Product* createProduct() override {
return new ConcreteProductB();
}
};
int main() {
Factory* factoryA = new ConcreteFactoryA();
Product* productA = factoryA->createProduct();
productA->operation();
Factory* factoryB = new ConcreteFactoryB();
Product* productB = factoryB->createProduct();
productB->operation();
delete factoryA;
delete productA;
delete factoryB;
delete productB;
return 0;
}
In this example, Product
is the abstract base class for the objects that will be created. ConcreteProductA
and ConcreteProductB
are concrete subclasses of Product
. The Factory
class declares the factory method createProduct()
, which is overridden by ConcreteFactoryA
and ConcreteFactoryB
to create instances of their respective products.
Using the Factory Design Pattern, client code can create objects through the factory without having to know the specific implementation classes. This promotes loose coupling and flexibility in object creation.