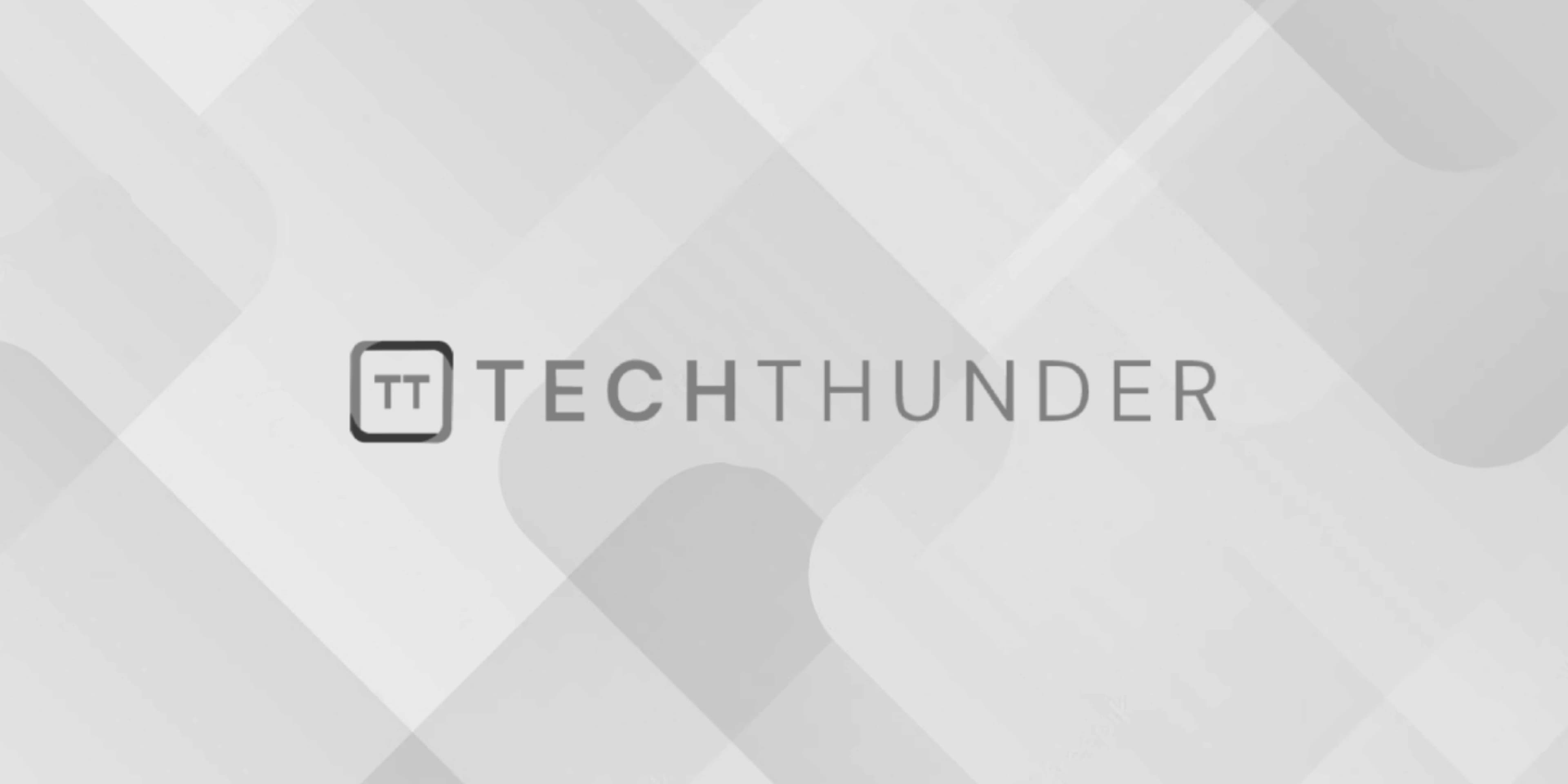
182 views
Type Conversion in C++
Type conversion in C++ refers to the process of converting a value from one data type to another. There are several ways to perform type conversion in C++, and they can be categorized into two main types: implicit and explicit type conversions.
- Implicit Type Conversion (Type Coercion):
- This type of conversion is performed automatically by the compiler when it’s safe to do so.
- It typically occurs in situations where a narrower data type is assigned to a wider data type, and no data loss is expected.
- Examples of implicit type conversion include:
- Converting an integer to a float or double.
- Promoting a smaller integer type (e.g.,
char
orshort
) to a larger integer type (e.g.,int
orlong
).
C++
int num1 = 42;
double num2 = num1; // Implicit conversion from int to double
- Explicit Type Conversion (Type Casting):
- This type of conversion is performed explicitly by the programmer and may result in data loss or undefined behavior.
- It is often used when you need to override the default behavior of implicit conversions.
- Examples of explicit type conversion include:
- C-style casting:
(type)value
- C++ casting operators:
static_cast
,dynamic_cast
,const_cast
, andreinterpret_cast
.
- C-style casting:
C++
double num1 = 3.14;
int num2 = static_cast<int>(num1); // Explicit conversion from double to int
There are four primary C++ casting operators:
static_cast
: Used for most common type conversions, such as converting between numeric types or upcasting in class hierarchies.dynamic_cast
: Used for safe downcasting in polymorphic class hierarchies (requires a polymorphic class with a virtual function).const_cast
: Used to add or remove theconst
qualifier from a variable.reinterpret_cast
: Used for low-level, unsafe type conversions between unrelated pointer types.
- User-Defined Type Conversion (Operator Overloading):
- In C++, you can define your own type conversions by overloading operators like
operator type()
. - This allows you to specify how objects of your custom class can be converted to other data types.
C++
class MyString {
public:
// User-defined conversion to const char*
operator const char*() const {
return str.c_str();
}
private:
std::string str = "Hello, World!";
};
int main() {
MyString myStr;
const char* cstr = myStr; // User-defined conversion to const char*
std::cout << cstr << std::endl;
return 0;
}
It’s essential to use type conversion carefully to avoid unexpected behavior or data loss, especially when performing explicit type conversions. Additionally, consider using type-safe alternatives like static_cast
and other C++ casting operators whenever possible, as they provide better compile-time checks and are less error-prone compared to C-style casting.