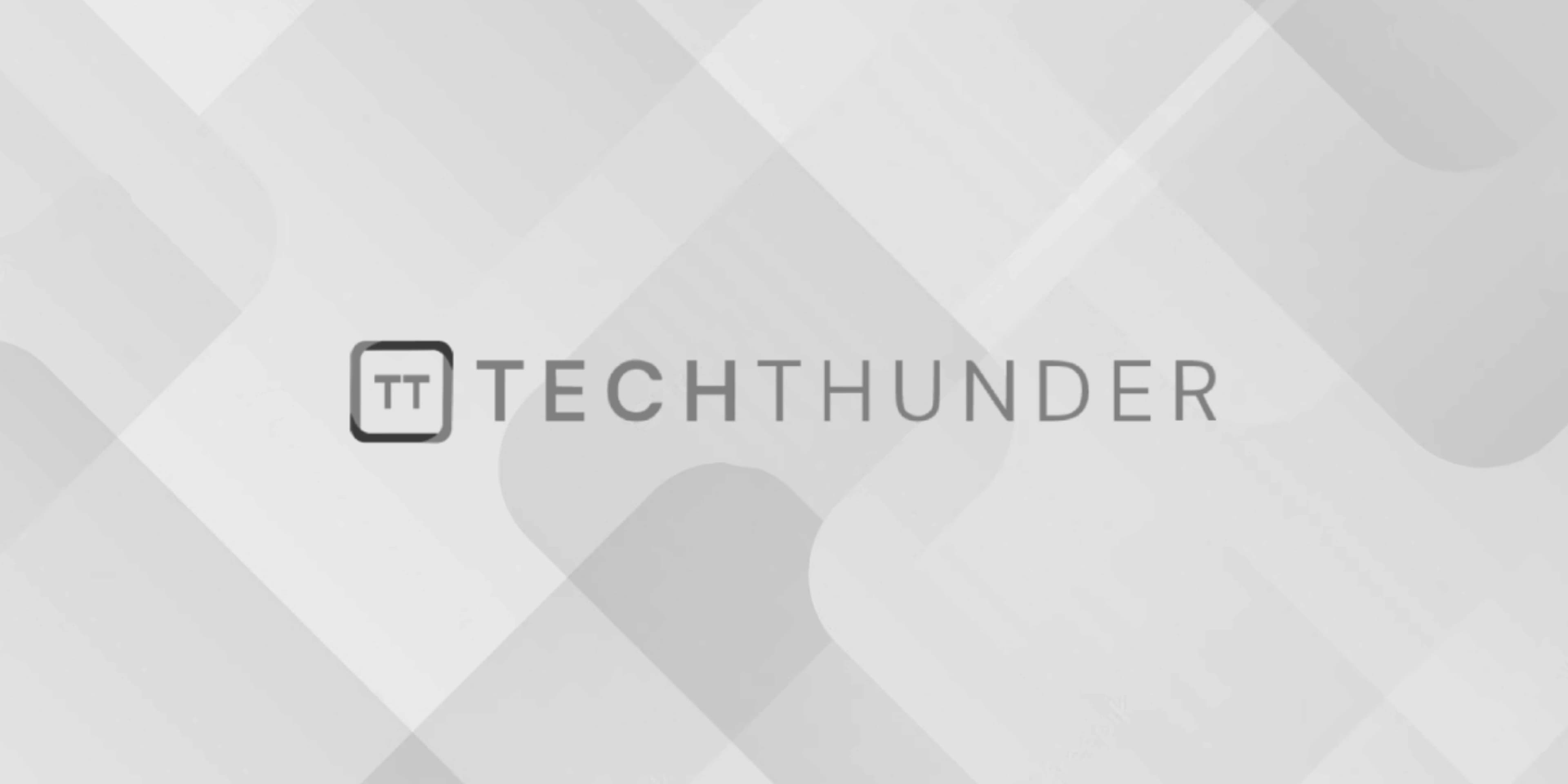
C++ Overloading
Function overloading in C++ is a feature that allows you to define multiple functions with the same name within the same scope, as long as they have different parameter lists. Overloaded functions have the same name but different parameter types or a different number of parameters. The compiler determines which function to call based on the number and types of arguments provided during a function call. Overloading enables you to create functions with similar functionality but different argument types or behaviors.
Here are the key concepts related to function overloading in C++:
- Function Signature: The function signature consists of the function’s name and its parameter list. Two functions with the same name and different parameter lists are considered overloaded.
int add(int a, int b); // Overloaded function 1
double add(double a, double b); // Overloaded function 2
- Overload Resolution: During a function call, the C++ compiler determines which overloaded function to invoke based on the arguments provided. This process is called overload resolution. The compiler matches the function call to the most appropriate overloaded function based on the number and types of arguments.
int result1 = add(1, 2); // Calls add(int, int)
double result2 = add(2.5, 3.5); // Calls add(double, double)
- Overloaded Functions with Default Arguments: You can also overload functions with default arguments. In this case, the function call can omit some arguments, and the compiler will select the overloaded function with the best match.
int add(int a, int b, int c = 0); // Overloaded function with a default argument
Here, you can call add(1, 2)
without providing the third argument, and it will use the default value of 0
.
- Function Overloading and Return Types: Function overloading is determined solely by the function’s signature (parameter list) and not by the return type. Overloaded functions can have different return types as long as the parameter lists differ.
int multiply(int a, int b); // Overloaded function 1
double multiply(double a, double b); // Overloaded function 2
- Operator Overloading: C++ allows you to overload operators for user-defined types. For example, you can define custom behavior for the
+
,-
,*
,/
, and other operators for your own classes.
class Complex {
public:
Complex operator+(const Complex& other) const;
};
This enables you to use the +
operator to add two Complex
objects.
- Function Overloading vs. Function Templates: While function overloading is useful for providing different implementations for different parameter types, function templates are used to create generic functions that work with various data types. Function templates provide more flexibility when you want to create functions that work with a wide range of argument types.
template <typename T>
T add(T a, T b) {
return a + b;
}
Here, the add
function template can work with integers, doubles, or any other type that supports addition.
Function overloading is a powerful feature in C++ that allows you to write code that is both concise and flexible. It makes it easier to work with different data types and create more intuitive and readable code by using descriptive function names.