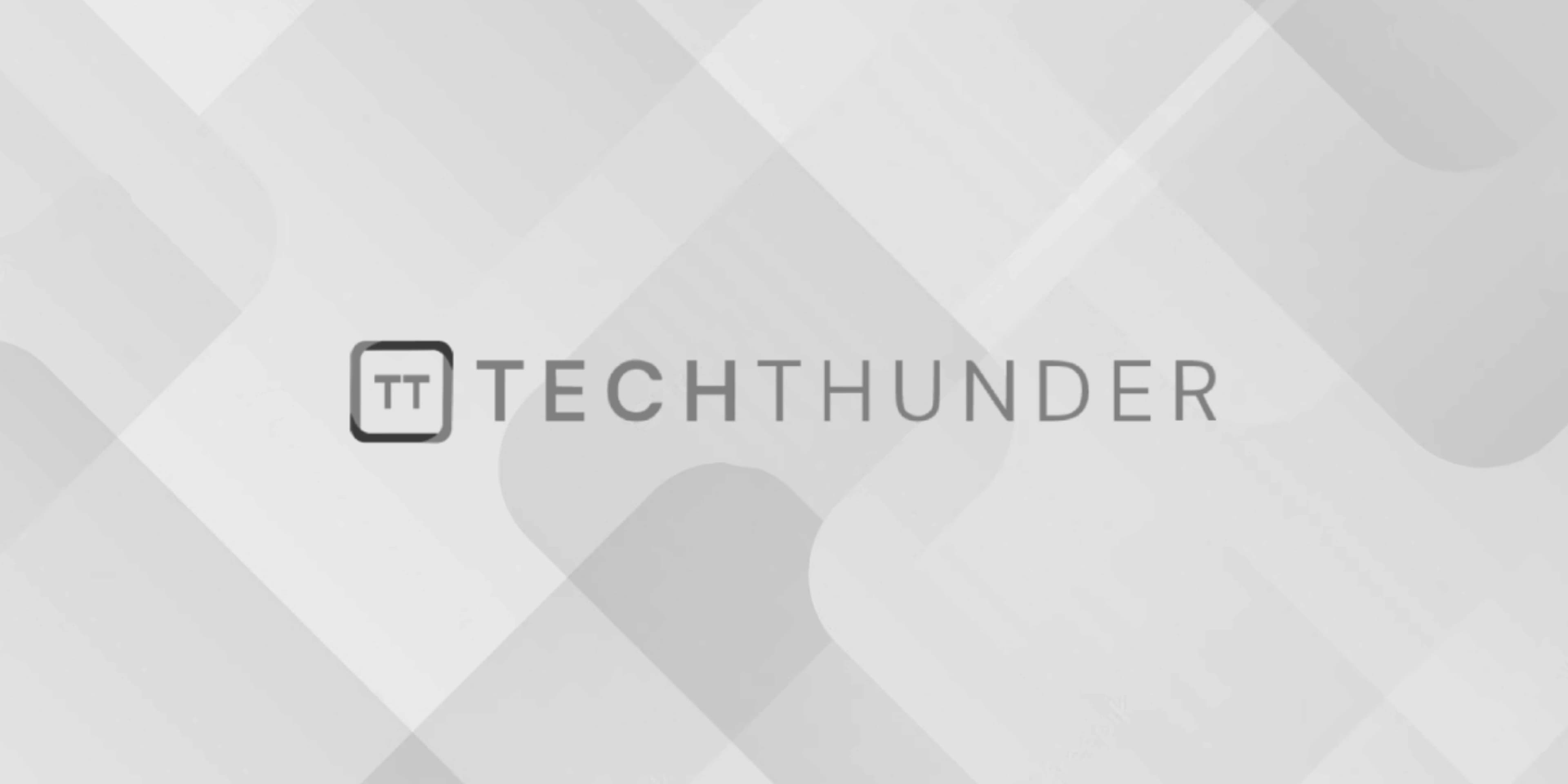
Binary Operator Overloading in C++
Binary operator overloading in C++ allows you to define custom behaviors for operators when they are used with user-defined types (classes or structs). This is achieved by overloading binary operators by providing custom implementations for them. Binary operators are operators that take two operands, such as addition (+
), subtraction (-
), multiplication (*
), division (/
), and many others. Here’s how you can overload binary operators in C++:
- Syntax for Overloading Binary Operators: To overload a binary operator, you define a member function or a non-member function with a specific format:
return_type operator op(const T1& operand1, const T2& operand2) {
// Custom behavior for the operator
}
return_type
is the type of the result of the operator.op
is the operator you want to overload (e.g.,+
,-
,*
,/
, etc.).T1
andT2
are the types of the operands.
- Member Function Overloading: You can overload binary operators as member functions within a class. For example:
class Complex {
public:
Complex operator+(const Complex& other) const {
Complex result;
result.real = this->real + other.real;
result.imaginary = this->imaginary + other.imaginary;
return result;
}
private:
double real;
double imaginary;
};
This allows you to use the +
operator with instances of the Complex
class.
- Non-Member Function Overloading: You can also overload binary operators as non-member functions, which is often necessary when one or both operands are not of the class for which you want to overload the operator. For example:
class Complex {
public:
Complex(double real, double imaginary) : real(real), imaginary(imaginary) {}
private:
double real;
double imaginary;
};
Complex operator+(const Complex& a, const Complex& b) {
return Complex(a.real + b.real, a.imaginary + b.imaginary);
}
This allows you to use the +
operator with two Complex
objects.
- Overloading for Built-in Types: You can also overload operators for built-in types by providing non-member function overloads. For example, you can overload the
+
operator for custom arithmetic types like vectors or matrices.
Binary operator overloading allows you to provide custom semantics for operators when working with user-defined types, making your code more expressive and natural. However, it should be used judiciously, and overloads should follow expected conventions to avoid confusion.