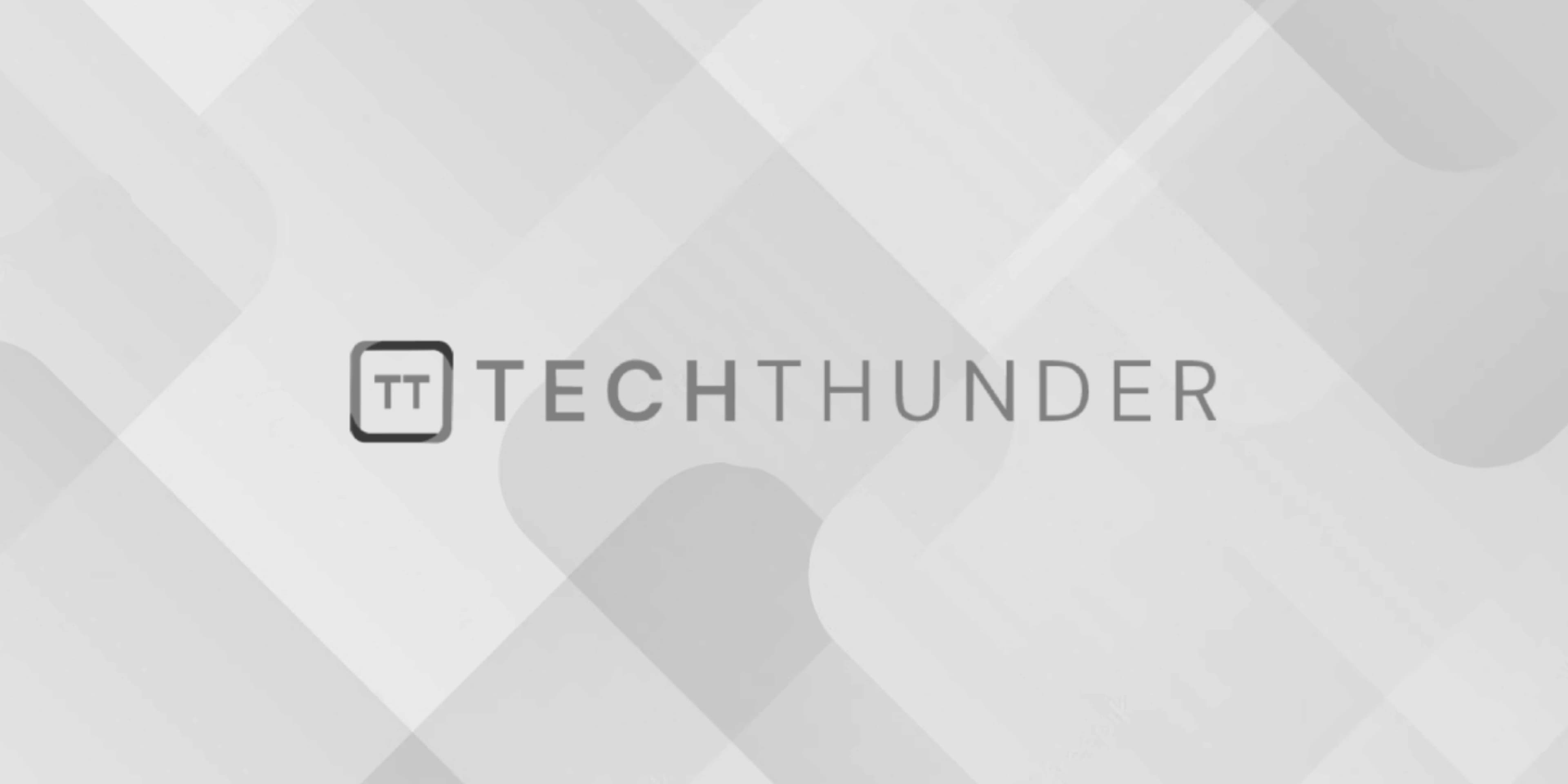
C++ switch
The switch
statement in C++ is a control flow statement that allows a program to evaluate the value of an expression and execute a block of code based on that value. It’s often used as an alternative to long chains of if-else
statements.
The basic syntax of a switch
statement is as follows:
switch (expression) {
case constant1:
// code to execute if expression equals constant1
break; // optional; exits the switch block
case constant2:
// code to execute if expression equals constant2
break;
// ...
default:
// code to execute if expression doesn't match any case
break;
}
Here’s an example of a switch
statement:
#include <iostream>
int main() {
int choice;
std::cout << "Enter a number (1-3): ";
std::cin >> choice;
switch (choice) {
case 1:
std::cout << "You chose option 1." << std::endl;
break;
case 2:
std::cout << "You chose option 2." << std::endl;
break;
case 3:
std::cout << "You chose option 3." << std::endl;
break;
default:
std::cout << "Invalid choice." << std::endl;
break;
}
return 0;
}
In this example:
- The user is prompted to enter a number.
- The program then uses a
switch
statement to evaluate the value ofchoice
. - If
choice
matches acase
constant, the corresponding code block is executed. In this case, it prints a message. - If
choice
doesn’t match any of thecase
constants, thedefault
case is executed.
Remember to include break
statements after each case to prevent “fall-through” behavior. Without break
, the code will continue to execute the statements of subsequent cases.
The default
case is optional. It provides a fallback in case none of the case
constants match the expression.
Note that the expression inside the switch
statement must be of an integral type (like int
, char
, enum
, etc.). It cannot be a floating-point type or a string.
The switch
statement is a useful tool for efficiently handling multiple possible values of a variable. However, it’s important to use it judiciously and consider using other control structures or data structures when they may be more appropriate.