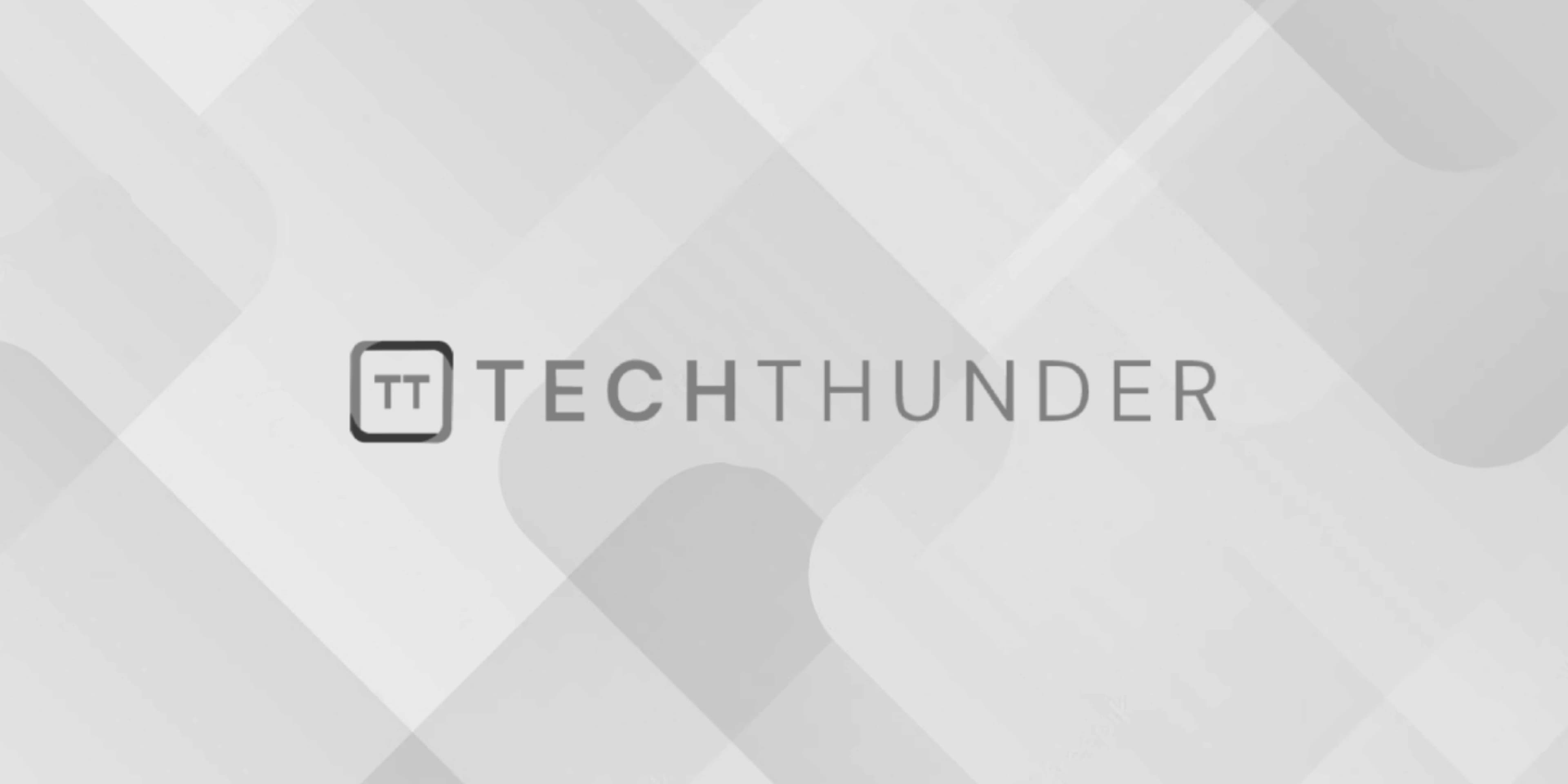
238 views
C++ Reference vs Pointer
The C++ both references and pointers are used to work with memory addresses, but they have different characteristics and use cases. Here’s a comparison of C++ references and pointers:
References:
- Declaration and Initialization:
- References must be initialized when declared.
- Once a reference is bound to an object, it cannot be re-bound to another object.
C++
int x = 10;
int& ref = x; // 'ref' is a reference to 'x'
- Null References: References cannot be null; they must always refer to a valid object. Trying to create a null reference results in a compilation error.
C++
int& ref = nullptr; // Compilation error
- Syntax for Access: References are used like regular variables. You don’t need to use the dereference operator (
*
) to access the value they refer to.
C++
int y = ref; // 'y' is assigned the value of 'x' through 'ref'
- Function Parameters:
- References are often used in function parameters when you want to modify the original object directly or avoid copying large objects.
- Passing by reference allows changes made inside a function to affect the original object.
C++
void modifyValue(int& val) {
val *= 2;
}
int num = 5;
modifyValue(num); // 'num' is modified directly
- Use in Operator Overloading:
- References can be used to return objects by reference in operator overloading.
C++
class MyVector {
public:
int& operator[](int index) {
return data[index];
}
};
Pointers:
- Declaration and Initialization:
- Pointers can be declared without immediate initialization.
- They can be re-assigned to point to different objects.
C++
int x = 10;
int* ptr; // Declare a pointer
ptr = &x; // Initialize the pointer with the address of 'x'
- Null Pointers: Pointers can be null (pointing to no object) by default or explicitly.
C++
int* ptr = nullptr; // Initialize with a null pointer
- Syntax for Access: To access the value pointed to by a pointer, you need to use the dereference operator (
*
).
C++
int y = *ptr; // 'y' is assigned the value pointed to by 'ptr'
- Function Parameters:
- Pointers are used in function parameters when you want to pass an object by reference, but you can also pass null pointers.
- Changes made inside a function using a pointer do affect the original object.
C++
void modifyValue(int* ptr) {
*ptr *= 2;
}
int num = 5;
modifyValue(&num); // 'num' is modified directly
- Dynamic Memory Allocation: Pointers are commonly used for dynamic memory allocation and deallocation using
new
anddelete
.
C++
int* dynamicPtr = new int; // Allocate memory
delete dynamicPtr; // Deallocate memory
The choice between using references and pointers depends on your specific requirements. References are typically preferred when you want to work with an existing object, and you want to ensure that the reference always points to a valid object. Pointers are more flexible and are commonly used for dynamic memory management and in scenarios where null pointers are needed. Each has its strengths and limitations, and understanding when to use each is important for effective C++ programming.