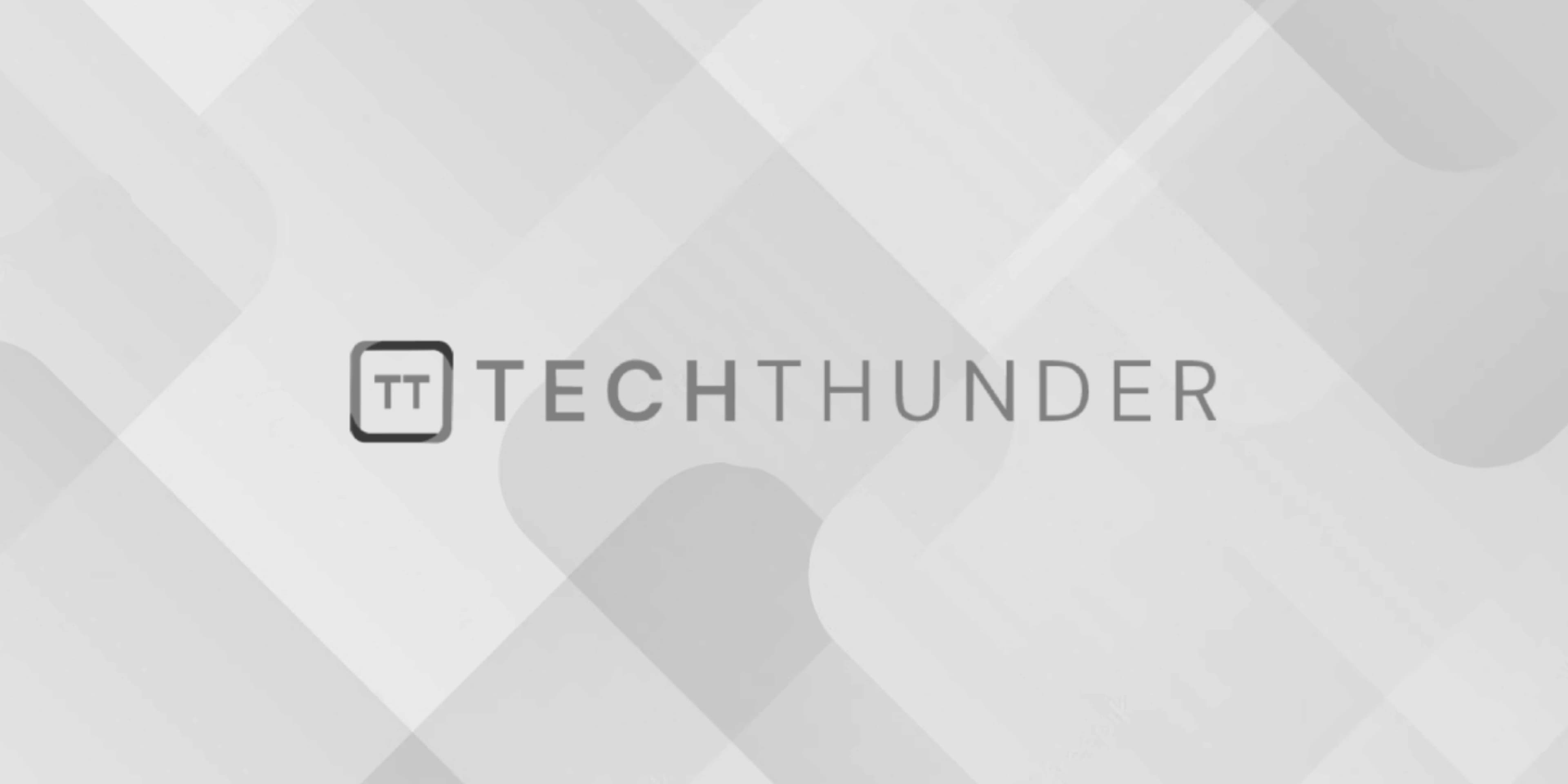
166 views
4-Dimensional Array in C++
A 4-dimensional array in C++ is an array that has four dimensions. It is essentially an array of arrays of arrays of arrays. You can declare and use a 4D array as follows:
C++
#include <iostream>
int main() {
// Define the dimensions of the 4D array
int dim1 = 2;
int dim2 = 3;
int dim3 = 4;
int dim4 = 5;
// Declare and initialize a 4D array
int arr4D[dim1][dim2][dim3][dim4];
// Initialize elements in the 4D array
for (int i = 0; i < dim1; ++i) {
for (int j = 0; j < dim2; ++j) {
for (int k = 0; k < dim3; ++k) {
for (int l = 0; l < dim4; ++l) {
arr4D[i][j][k][l] = i * 1000 + j * 100 + k * 10 + l;
}
}
}
}
// Access and print elements in the 4D array
for (int i = 0; i < dim1; ++i) {
for (int j = 0; j < dim2; ++j) {
for (int k = 0; k < dim3; ++k) {
for (int l = 0; l < dim4; ++l) {
std::cout << "arr4D[" << i << "][" << j << "][" << k << "][" << l << "] = "
<< arr4D[i][j][k][l] << std::endl;
}
}
}
}
return 0;
}
In this example:
- We define the dimensions of the 4D array using
dim1
,dim2
,dim3
, anddim4
. - We declare and initialize a 4D array
arr4D
with the specified dimensions. - We initialize the elements in the 4D array using nested loops.
- We access and print the elements in the 4D array using nested loops.
Keep in mind that working with multi-dimensional arrays can become complex, especially as the number of dimensions increases. In practice, it is often more convenient to use alternative data structures like nested std::vector
containers or consider whether a higher-dimensional array is truly necessary or if a different data structure might better suit your needs.