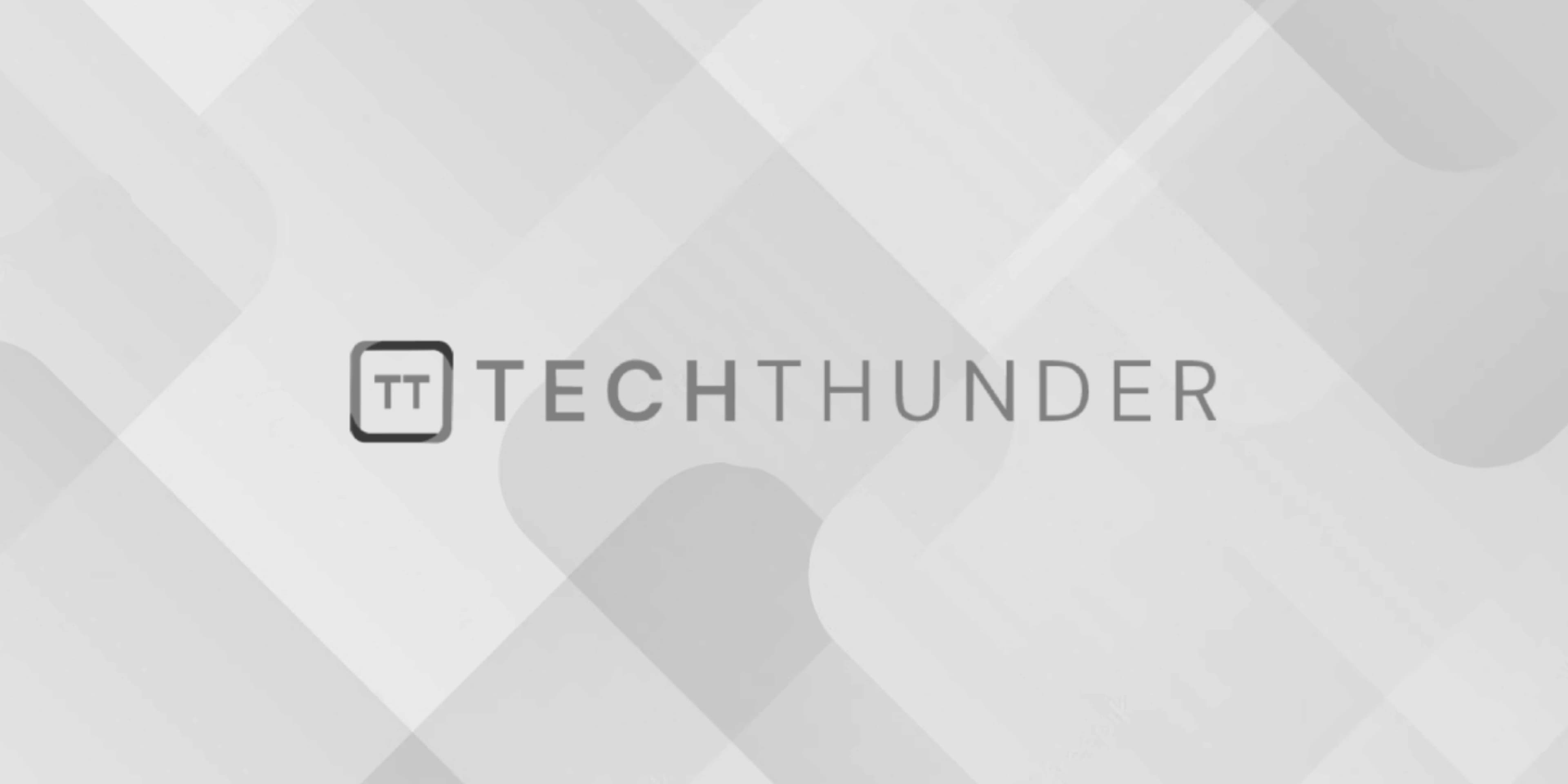
312 views
C++ Signal Handling
The C++ signal handling allows you to handle asynchronous events, known as signals, that can occur during the execution of a program. Signals are typically used to notify a program of various events, such as user interrupts (e.g., pressing Ctrl+C), segmentation faults, and more. You can write signal handlers to specify how your program should respond when a specific signal is received.
Here’s an overview of how to handle signals in C++:
- Include Required Headers: You need to include the necessary headers for working with signals. In C++, you can include the
<csignal>
header for this purpose.
C++
#include <csignal>
- Declare a Signal Handler Function: To handle a specific signal, you need to declare a function with the appropriate signature. The function should take an integer parameter representing the signal number.
C++
void signalHandler(int signum) {
// Your signal handling code here
}
- Register the Signal Handler: You can register your signal handler function using the
signal
function provided by the<csignal>
header. Thesignal
function associates your signal handler function with a specific signal.
C++
#include <iostream>
#include <csignal>
void signalHandler(int signum) {
std::cout << "Received signal: " << signum << std::endl;
}
int main() {
// Register the signal handler for SIGINT (Ctrl+C)
signal(SIGINT, signalHandler);
// Keep the program running
while (true) {
// Your program logic here
}
return 0;
}
- Handling the Signal: When a signal is raised, your signal handler function (
signalHandler
in this example) will be called. Inside this function, you can implement the logic to handle the signal. Be cautious when performing operations inside a signal handler, as it should be kept simple and not rely on complex or unsafe operations. In the example above, when Ctrl+C is pressed (sending a SIGINT signal), thesignalHandler
function will be called, and it will print a message indicating the received signal. - Restoring Default Behavior (Optional): You can restore the default behavior for a signal by registering the signal handler with the
SIG_DFL
constant. For example:
C++
signal(SIGINT, SIG_DFL); // Restore default behavior for SIGINT
- Blocking Signals (Optional): You can block specific signals temporarily within a section of code using functions like
sigprocmask
orpthread_sigmask
. Blocking signals prevents them from being delivered to your program during the blocked period.
C++
#include <csignal>
#include <iostream>
int main() {
sigset_t sigset;
sigemptyset(&sigset);
sigaddset(&sigset, SIGINT);
sigprocmask(SIG_BLOCK, &sigset, nullptr);
// Your critical code section here
sigprocmask(SIG_UNBLOCK, &sigset, nullptr);
return 0;
}
Remember that handling signals in C++ can be tricky, as it introduces asynchronous behavior into your program. It’s essential to keep signal handlers simple and avoid complex operations, especially memory allocation, within signal handlers to prevent potential issues like deadlocks or corruption.