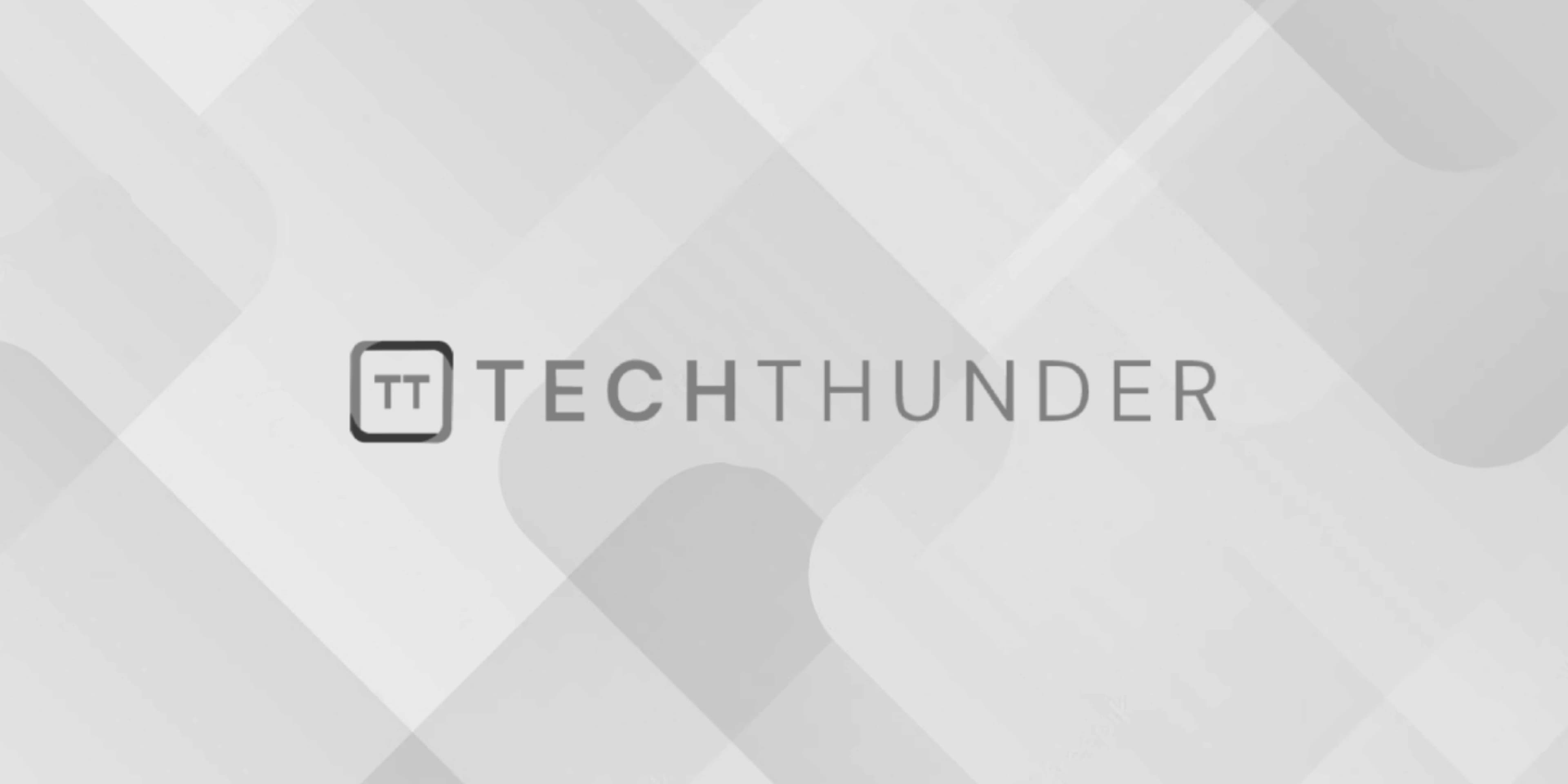
156 views
How To Convert a Qstring to Hexadecimal in C++
To convert a QString
to a hexadecimal representation in C++, you can use the following approach. Here’s an example using Qt:
C++
#include <iostream>
#include <QString>
#include <QByteArray>
#include <QTextCodec>
int main() {
QString originalString = "Hello, World!";
// Convert QString to QByteArray using the UTF-8 encoding
QByteArray byteArray = originalString.toUtf8();
// Convert QByteArray to a hexadecimal string
QString hexString = byteArray.toHex();
std::cout << "Original QString: " << originalString.toStdString() << std::endl;
std::cout << "Hexadecimal representation: " << hexString.toStdString() << std::endl;
return 0;
}
In this example:
- We start with a
QString
calledoriginalString
that contains the text you want to convert to a hexadecimal representation. - We use
toUtf8()
to convert theQString
to aQByteArray
using the UTF-8 encoding. This step is necessary to ensure that non-ASCII characters are handled correctly. - We then use
toHex()
on theQByteArray
to obtain the hexadecimal representation as aQString
. - Finally, we print the original
QString
and the hexadecimal representation to the console.
This example assumes that you’re working with a Qt project and have the necessary Qt libraries included. If you’re working with plain C++ and not using Qt, you can use standard C++ libraries for string encoding and conversion.