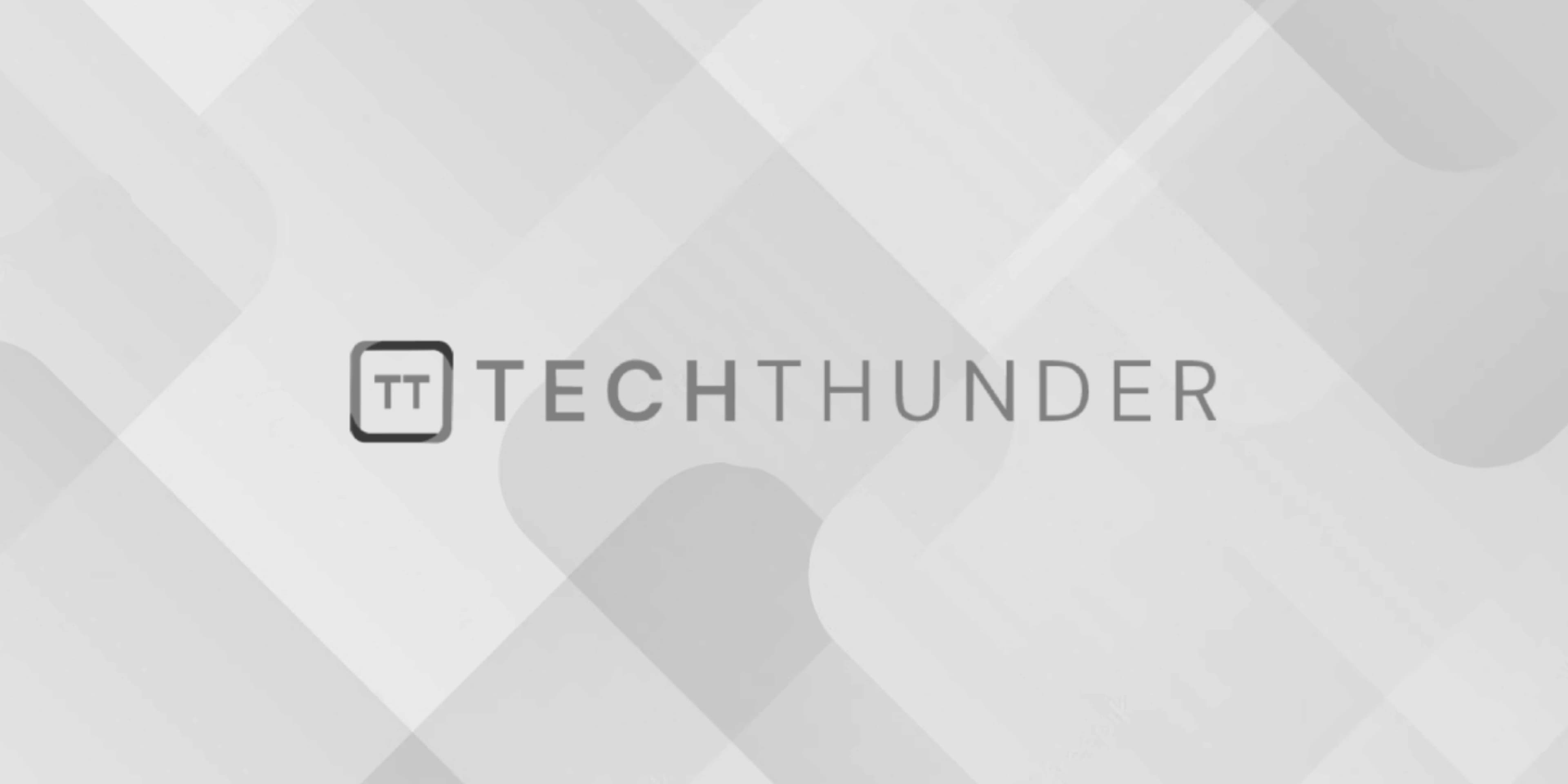
213 views
C++ Program
The simple C++ program that calculates the factorial of a number entered by the user using a for
loop:
C++
#include <iostream>
int main() {
int num;
long long factorial = 1;
std::cout << "Enter a positive integer: ";
std::cin >> num;
if (num < 0) {
std::cout << "Factorial is not defined for negative numbers." << std::endl;
} else {
for (int i = 1; i <= num; ++i) {
factorial *= i;
}
std::cout << "Factorial of " << num << " is " << factorial << std::endl;
}
return 0;
}
This program does the following:
- Includes the
<iostream>
header for input and output operations. - Defines the
main
function as the entry point of the program. - Declares an integer variable
num
to store the user’s input and along long
variablefactorial
to store the result. - Prompts the user to enter a positive integer.
- Reads the user’s input into the
num
variable usingcin
. - Checks if the entered number is negative. If it’s negative, it displays a message that factorial is not defined for negative numbers.
- If the number is non-negative, it calculates the factorial using a
for
loop and displays the result.
Compile and run this program, and it will calculate the factorial of the input number.