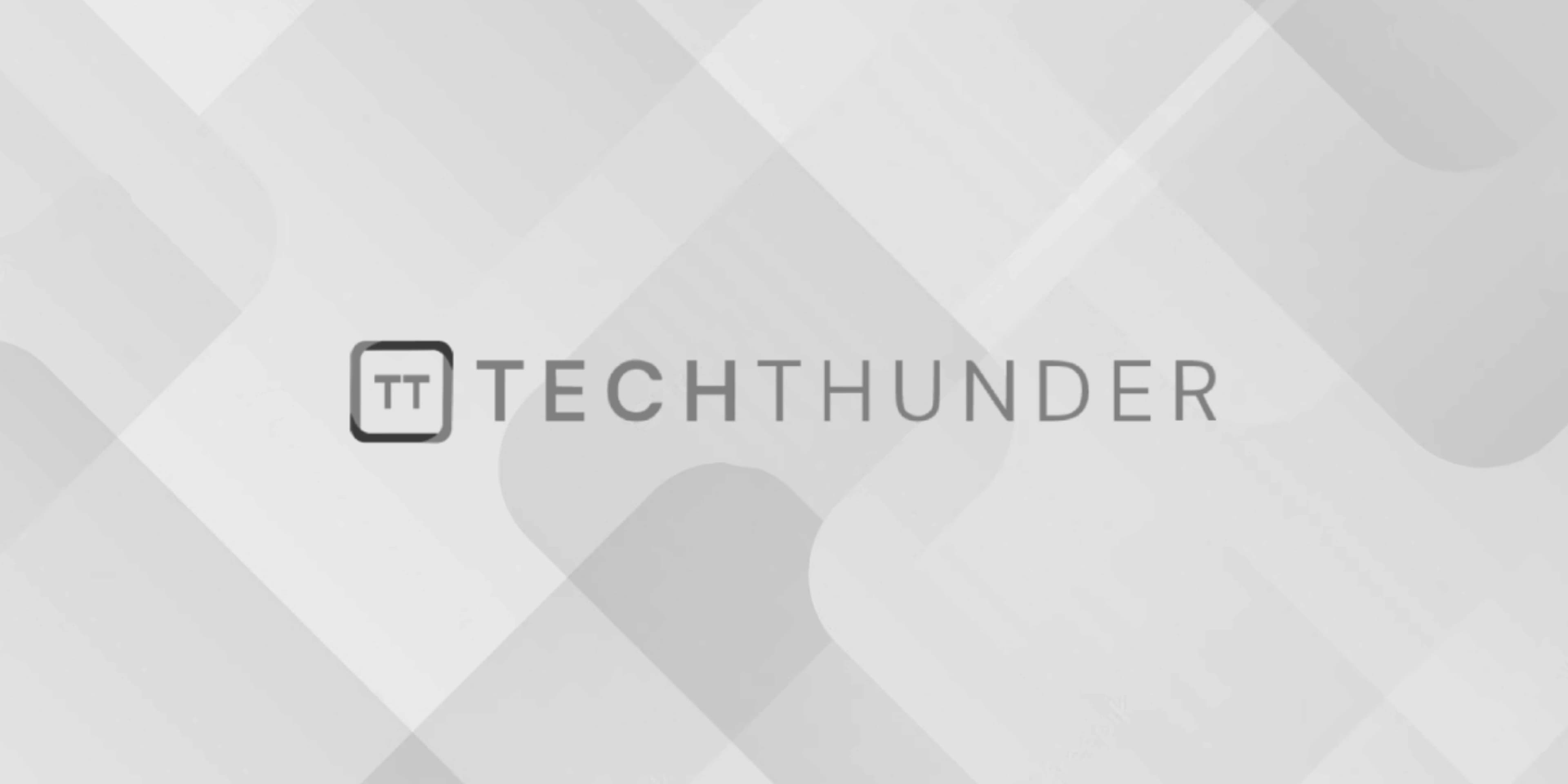
296 views
Forward List in C++ Manipulating Functions
A std::forward_list
in C++ is a singly-linked list container from the Standard Template Library (STL). It provides a limited set of operations compared to other containers like std::vector
or std::list
because it only allows forward iteration and does not support bidirectional or random access.
Here are some common manipulation functions for std::forward_list
:
- push_front(): Inserts an element at the beginning of the list.
C++
std::forward_list<int> myList;
myList.push_front(42);
- pop_front(): Removes the first element from the list.
C++
myList.pop_front();
- insert_after(): Inserts an element after a specified position (an iterator).
C++
auto it = myList.begin();
myList.insert_after(it, 30);
- emplace_after(): Constructs an element in-place after a specified position (an iterator).
C++
auto it = myList.begin();
myList.emplace_after(it, 55);
- erase_after(): Removes an element after a specified position (an iterator).
C++
auto it = myList.begin();
myList.erase_after(it);
- remove(): Removes all elements with a specified value.
C++
myList.remove(42);
- remove_if(): Removes elements that satisfy a given predicate.
C++
myList.remove_if([](int x){ return x % 2 == 0; }); // Removes even numbers
- splice_after(): Transfers elements from one list to another or within the same list.
C++
std::forward_list<int> otherList = {1, 2, 3};
auto it = myList.before_begin();
myList.splice_after(it, otherList);
- merge(): Merges two sorted lists into one sorted list.
C++
std::forward_list<int> otherList = {4, 5, 6};
myList.sort();
otherList.sort();
myList.merge(otherList);
- reverse(): Reverses the order of elements in the list.
myList.reverse();
- unique(): Removes consecutive duplicate elements.
myList.unique();
- clear(): Removes all elements from the list.
myList.clear();
These are some of the key manipulation functions available for std::forward_list
. Keep in mind that std::forward_list
is optimized for insertion and deletion at the beginning of the list, but it has limitations when it comes to random access or operations at the end of the list.