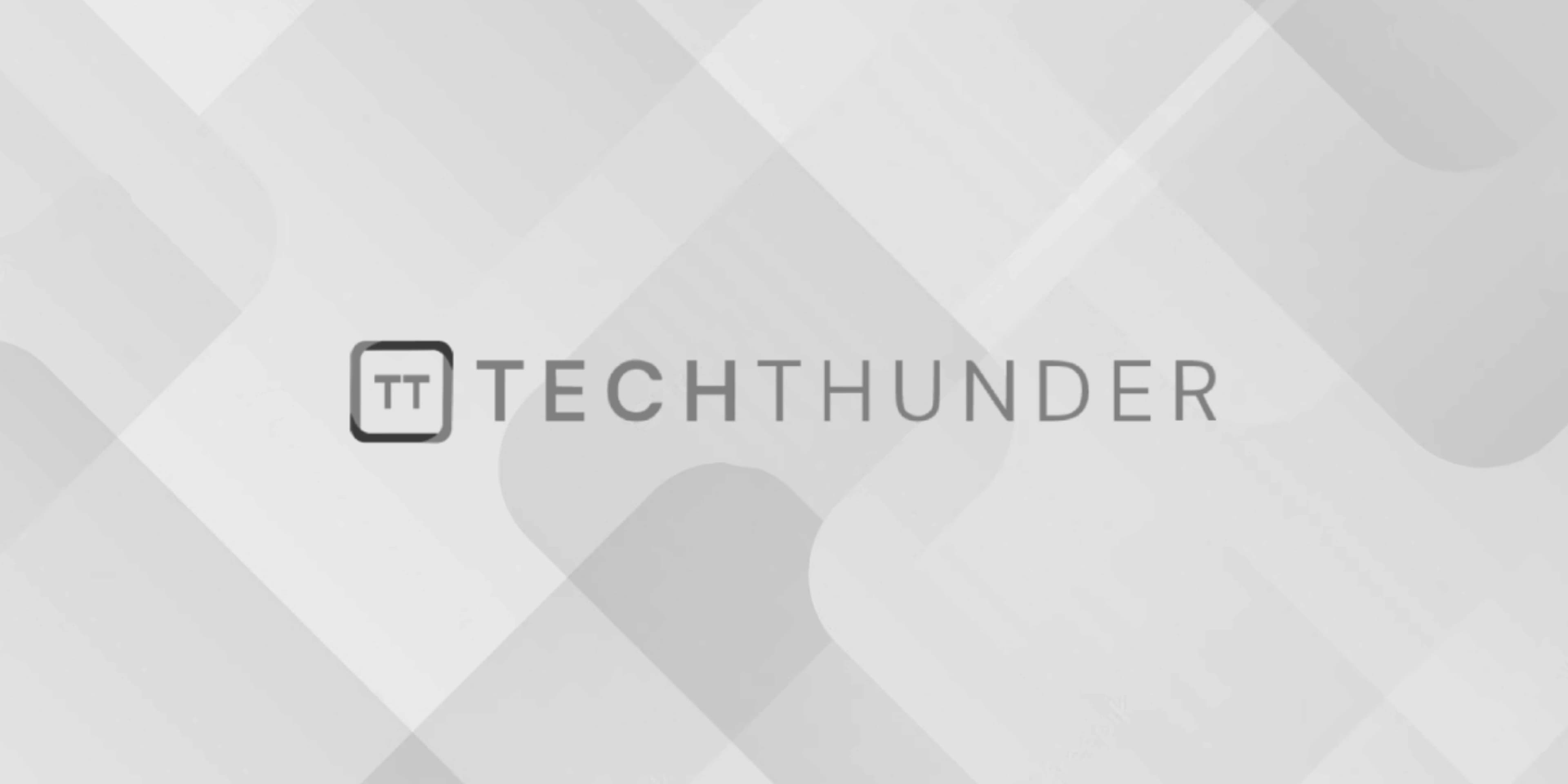
C++ Call by value & reference
The C++ function parameters can be passed by value or by reference, and this has important implications for how the function interacts with the arguments. Let’s explore the differences between these two methods:
Call by Value:
When you pass a parameter by value, a copy of the argument’s value is made, and the function operates on this copy. Any changes made to the parameter within the function do not affect the original argument.
Here’s an example of passing by value:
#include <iostream>
void modifyValue(int x) {
x = 10;
}
int main() {
int num = 5;
modifyValue(num);
std::cout << "Original value: " << num << std::endl; // Output: Original value: 5
return 0;
}
In the above code, num
is passed by value to the modifyValue
function. Inside the function, x
is a copy of num
, and changing x
does not affect the original num
.
Call by Reference:
When you pass a parameter by reference, you are passing a reference to the original object, not a copy. Any changes made to the parameter within the function directly affect the original argument.
Here’s an example of passing by reference:
#include <iostream>
void modifyReference(int &x) {
x = 10;
}
int main() {
int num = 5;
modifyReference(num);
std::cout << "Modified value: " << num << std::endl; // Output: Modified value: 10
return 0;
}
In this case, num
is passed by reference to the modifyReference
function. Any changes made to x
inside the function are reflected in the original num
.
When to Use Which:
- Call by Value: Use this when you want to work with a copy of the original data and do not want to modify the original data. It’s safer when you don’t want the function to unintentionally modify the original value.
- Call by Reference: Use this when you want to modify the original data within the function. It’s more efficient since it avoids copying large objects, but you should be cautious about unintended side effects.
You can also use const
with references to indicate that the function will not modify the original data:
void readOnly(const int &x) {
// Cannot modify x here
}
This prevents accidental modifications within the function.