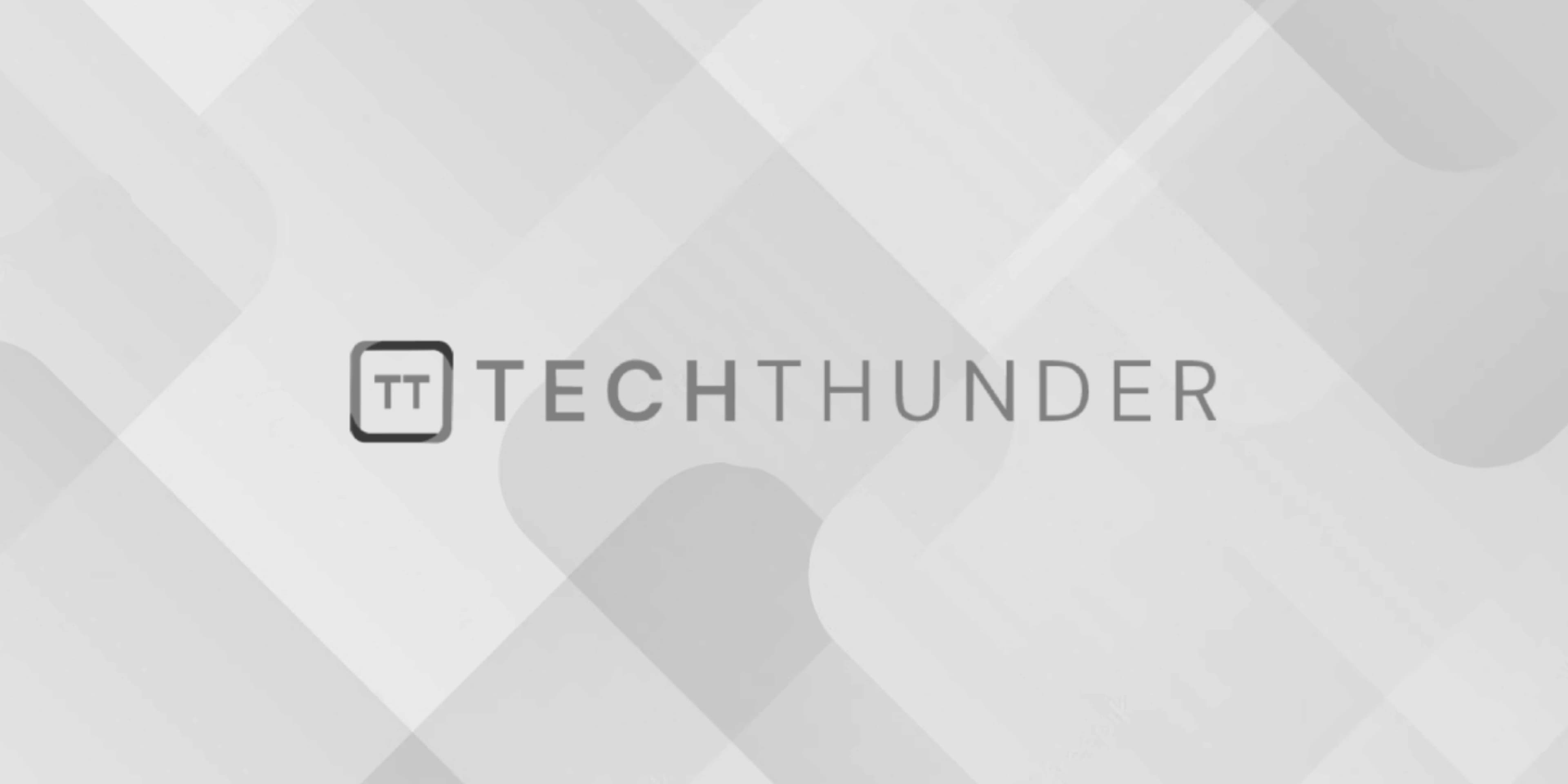
269 views
C++ OOPs Concepts
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects, which are instances of classes. C++ is a language that fully supports OOP principles. Here are the key OOP concepts in C++:
- Class: A class is a blueprint or template for creating objects. It defines the attributes (data members) and behaviors (member functions) that the objects of the class will have. For example:
C++
class Car {
private:
std::string brand;
int year;
public:
// Constructor
Car(std::string b, int y) : brand(b), year(y) {}
// Member function
void start() {
std::cout << "The car is starting." << std::endl;
}
};
- Object: An object is an instance of a class. It represents a real-world entity and can have its own unique data and state. For example:
C++
Car myCar("Toyota", 2022);
myCar.start();
- Encapsulation: Encapsulation is the bundling of data (attributes) and functions (methods) that operate on that data into a single unit, i.e., the class. Access to the data is controlled through public, private, and protected access specifiers. This concept helps in data hiding and abstraction.
- Abstraction: Abstraction is the process of simplifying complex reality by modeling classes based on essential properties and behaviors. It allows you to focus on what an object does rather than how it does it. In C++, you can achieve abstraction through classes and interfaces.
- Inheritance: Inheritance is the mechanism that allows a class (the derived or child class) to inherit properties and behaviors from another class (the base or parent class). It promotes code reuse and establishes a hierarchical relationship between classes.
C++
class ElectricCar : public Car {
private:
int batteryCapacity;
public:
ElectricCar(std::string b, int y, int cap) : Car(b, y), batteryCapacity(cap) {}
void charge() {
std::cout << "Charging the electric car." << std::endl;
}
};
- Polymorphism: Polymorphism means “many shapes” and refers to the ability of objects of different classes to respond to the same method call in their own unique way. C++ supports compile-time (function overloading) and runtime (virtual functions) polymorphism.
C++
void driveCar(Car& car) {
car.start();
}
Car regularCar("Honda", 2021);
ElectricCar electricCar("Tesla", 2023, 75);
driveCar(regularCar); // Calls Car::start()
driveCar(electricCar); // Calls ElectricCar::start()
- Polymorphic Behavior Using Virtual Functions: To achieve runtime polymorphism, C++ uses virtual functions. A virtual function is declared in the base class with the
virtual
keyword, and it can be overridden in derived classes.
C++
class Vehicle {
public:
virtual void start() {
std::cout << "Starting the vehicle." << std::endl;
}
};
class Bike : public Vehicle {
public:
void start() override {
std::cout << "Starting the bike." << std::endl;
}
};
Vehicle* vehicle = new Bike();
vehicle->start(); // Calls Bike::start() due to dynamic binding
- Composition: Composition is a design principle where a class can contain objects of other classes as data members. It allows you to build complex objects by combining simpler ones.
C++
class Engine {
public:
void start() {
std::cout << "Engine started." << std::endl;
}
};
class Car {
private:
Engine carEngine;
public:
void start() {
carEngine.start();
std::cout << "Car started." << std::endl;
}
};
These are the fundamental Object-Oriented Programming concepts in C++. Using these concepts, you can design and implement robust and maintainable software by modeling real-world entities and their interactions.