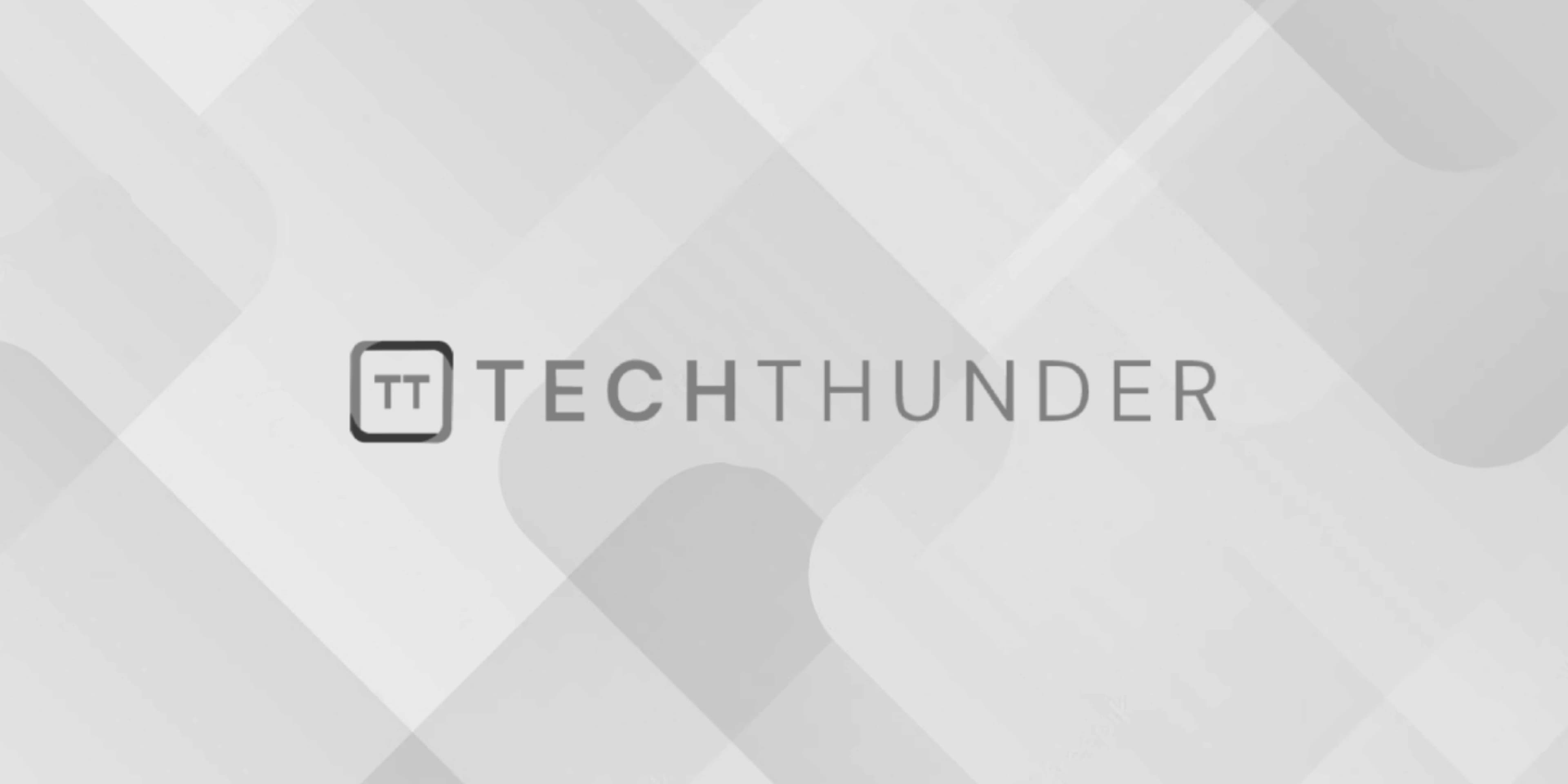
216 views
C++ Map
The C++ std::map
is a container provided by the C++ Standard Library that allows you to store and manage a collection of key-value pairs. It is implemented as a balanced binary search tree, typically a Red-Black Tree, which ensures that the keys are stored in sorted order. Each key in the map is associated with a value, and you can use the key to look up and retrieve the corresponding value efficiently.
Here’s how to use std::map
in C++:
C++
#include <iostream>
#include <map>
#include <string>
int main() {
// Create a map with integer keys and string values
std::map<int, std::string> myMap;
// Insert key-value pairs into the map
myMap[1] = "Alice";
myMap[2] = "Bob";
myMap[3] = "Charlie";
// Access values using keys
std::cout << "Value for key 2: " << myMap[2] << std::endl;
// Check if a key exists in the map
if (myMap.find(4) != myMap.end()) {
std::cout << "Key 4 found in the map." << std::endl;
} else {
std::cout << "Key 4 not found in the map." << std::endl;
}
// Iterate over the map
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
In this example:
- We include the
<map>
header to usestd::map
. - We create a map with integer keys and string values (
std::map<int, std::string>
). - Key-value pairs are inserted using the square bracket operator (
[]
). - We can access values in the map using keys, and we use
find
to check if a key exists. - To iterate over the map, we use a range-based for loop, which provides access to each key-value pair in the map.
std::map
is a powerful and versatile data structure for maintaining collections of key-value pairs in sorted order. It’s commonly used in scenarios where you need efficient key-based lookup and automatic sorting of data.