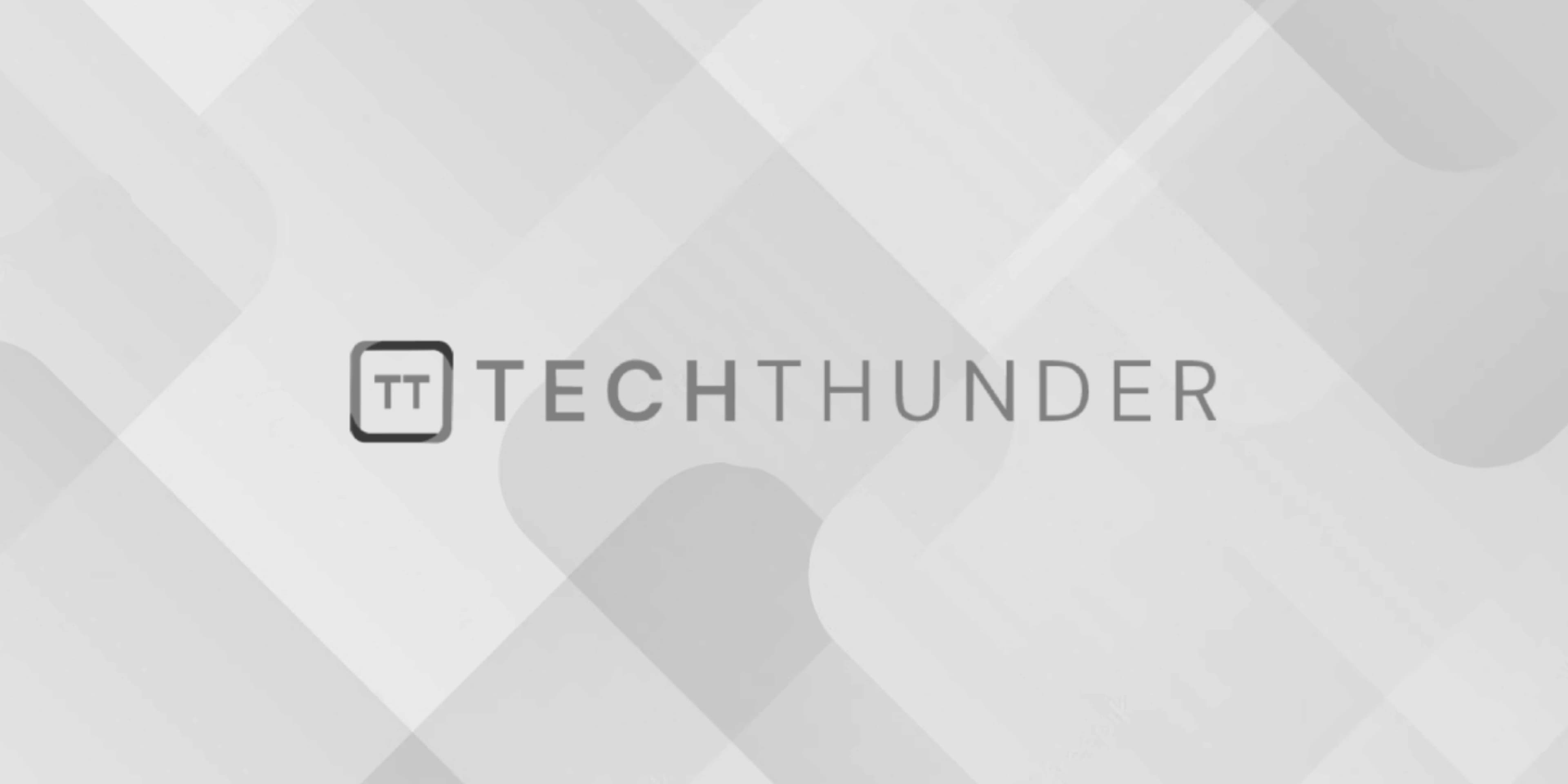
151 views
C++ Inheritance
The C++ inheritance is one of the fundamental concepts of object-oriented programming (OOP). It allows you to create new classes that are based on existing classes, inheriting their properties and behaviors. Inheritance helps in reusing and extending code, which is a key principle of OOP.
Here are some important aspects of C++ inheritance:
- Base Class and Derived Class: Inheritance involves two types of classes – the base class (also called the parent class) and the derived class (also called the child class). The derived class inherits attributes and behaviors from the base class.
- Syntax for Inheritance:
C++
class BaseClass {
// Base class members and methods
};
class DerivedClass : access-specifier BaseClass {
// Derived class members and methods
};
access-specifier
can be one of three:public
,protected
, orprivate
. It determines the access level of the base class members in the derived class.public
: The public members of the base class remain public in the derived class.protected
: The public members of the base class become protected in the derived class.private
: The public members of the base class become private in the derived class.
- Access Control:
- Public members of the base class are accessible in the derived class with the same access control.
- Protected members of the base class become protected in the derived class.
- Private members of the base class are not accessible in the derived class.
- Constructor and Destructor Inheritance: Constructors and destructors are also inherited, but they are not directly callable from the derived class. The derived class can call them using the base class’s constructor and destructor.
- Types of Inheritance:
- Single Inheritance: A class inherits from only one base class.
- Multiple Inheritance: A class can inherit from more than one base class.
- Multilevel Inheritance: A class inherits from another derived class.
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
- Hybrid Inheritance: A combination of the above types.
- Virtual Functions: In C++, you can use virtual functions to achieve runtime polymorphism and dynamic binding. When a function is declared as virtual in the base class, it can be overridden in derived classes, and the appropriate function is called based on the object’s actual type at runtime.
Here’s a simple example of C++ inheritance:
C++
#include <iostream>
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() {
std::cout << "Dog barks" << std::endl;
}
};
int main() {
Animal animal;
Dog dog;
animal.speak(); // Output: "Animal speaks"
dog.speak(); // Output: "Dog barks"
Animal* ptr = &dog;
ptr->speak(); // Output: "Dog barks" due to polymorphism
return 0;
}
In this example, Dog
is a derived class of Animal
, and it overrides the speak
method. Polymorphism allows us to call the correct speak
method based on the actual object type, as demonstrated when calling speak
through a pointer to the base class.