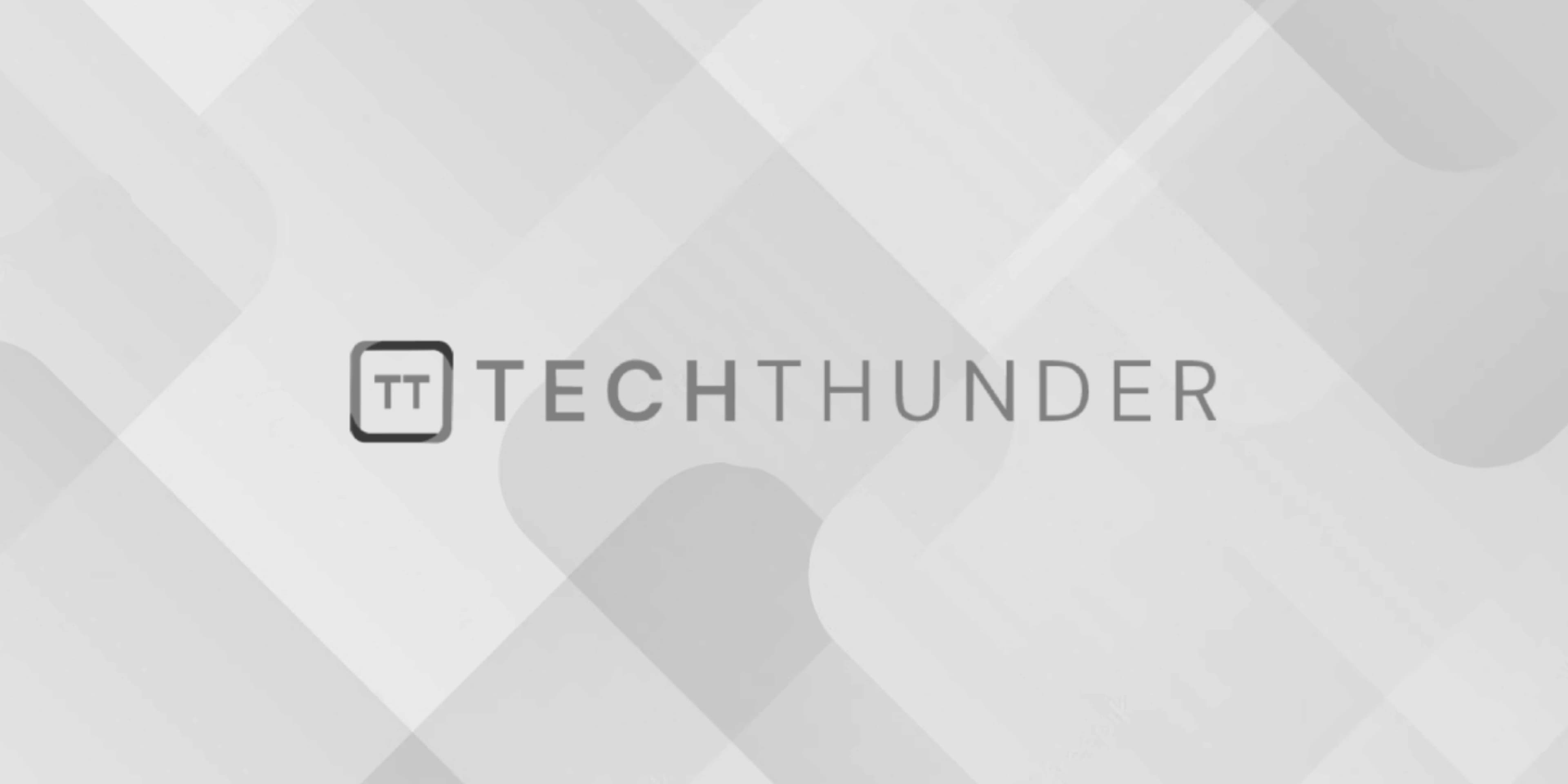
275 views
Vector Pair in C++
The C++ can use a vector of pairs to store and manipulate a collection of pairs of elements. A std::pair
is a simple template class provided by the C++ Standard Library that allows you to store two values of potentially different types together. Here’s how you can create and use a vector of pairs in C++:
C++
#include <iostream>
#include <vector>
#include <utility> // Include the utility header for std::pair
int main() {
// Create a vector of pairs
std::vector<std::pair<int, std::string>> vec;
// Add elements to the vector of pairs
vec.push_back(std::make_pair(1, "One"));
vec.push_back(std::make_pair(2, "Two"));
vec.push_back(std::make_pair(3, "Three"));
// Access elements in the vector of pairs
for (const auto& pair : vec) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
In this example:
- We include the
<utility>
header to usestd::pair
. - We create a vector
vec
that stores pairs where the first element is an integer (int
) and the second element is a string (std::string
). - We use
std::make_pair
to create pairs andpush_back
to add them to the vector. - We iterate through the vector using a range-based for loop to access and print the elements of each pair.
Output:
Plaintext
1: One
2: Two
3: Three
You can use vectors of pairs to store and manipulate collections of pairs, such as key-value pairs or any other combination of two values. They are especially useful when you need to maintain associations between two related pieces of data.