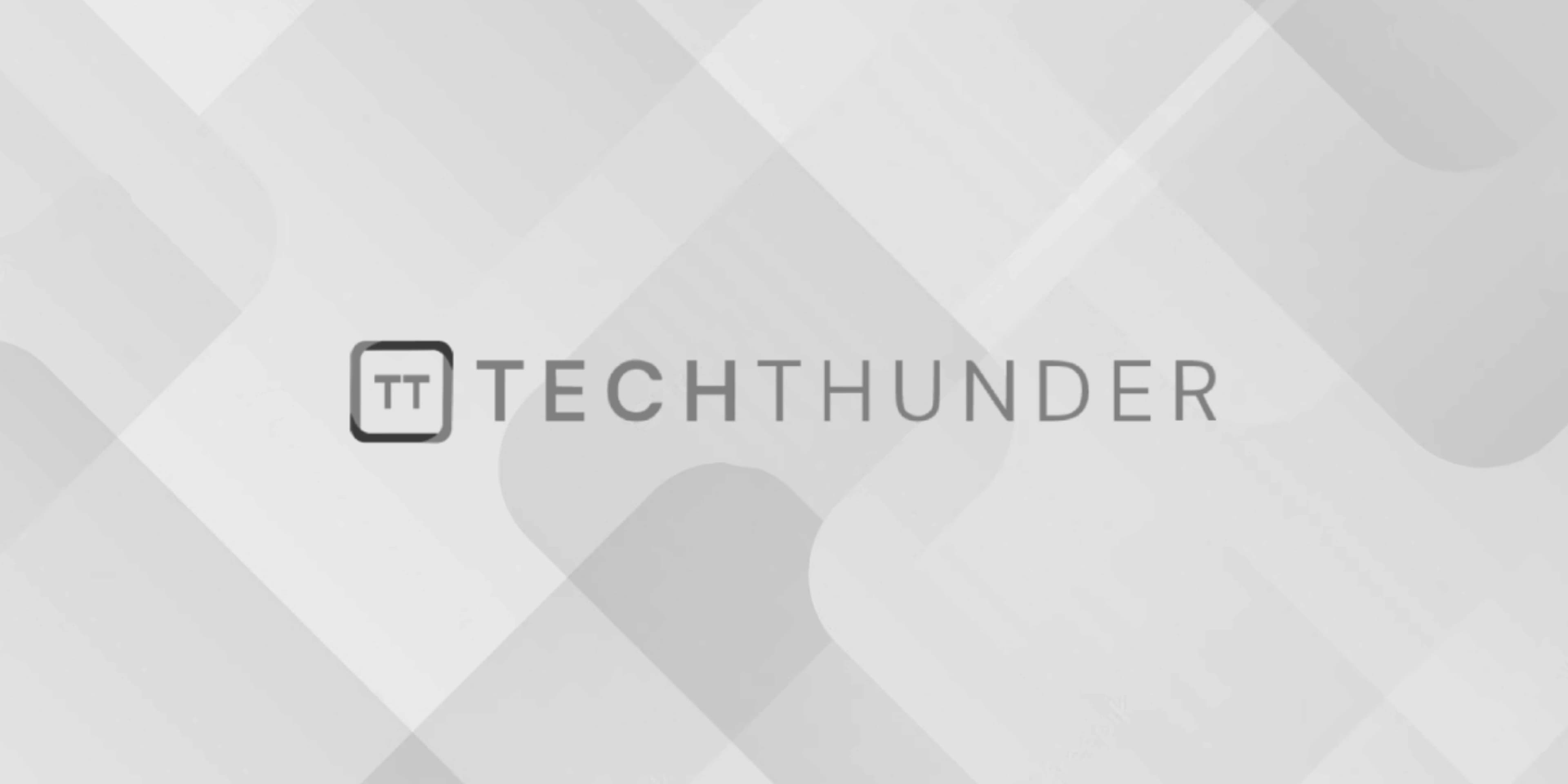
151 views
How to concatenate two strings in c++
The C++ can concatenate two strings using several methods. Here are three common approaches:
- Using the
+
Operator:
You can use the+
operator to concatenate two strings just like you would concatenate two numbers.
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2;
std::cout << result << std::endl;
return 0;
}
In this example, str1
and str2
are concatenated to create result
.
- Using the
+=
Operator:
You can also use the+=
operator to append one string to the end of another.
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
str1 += str2;
std::cout << str1 << std::endl;
return 0;
}
In this example, str2
is appended to the end of str1
.
- Using the
append()
Function:
You can use theappend()
function to concatenate two strings.
C++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
str1.append(str2);
std::cout << str1 << std::endl;
return 0;
}
Here, str2
is appended to the end of str1
using the append()
function.
All three methods mentioned above will concatenate the two strings and produce the same result. The choice of method depends on your preference and the specific requirements of your code. The +
operator is generally more concise and readable, while the +=
operator and append()
function can be useful when you want to modify an existing string in place.