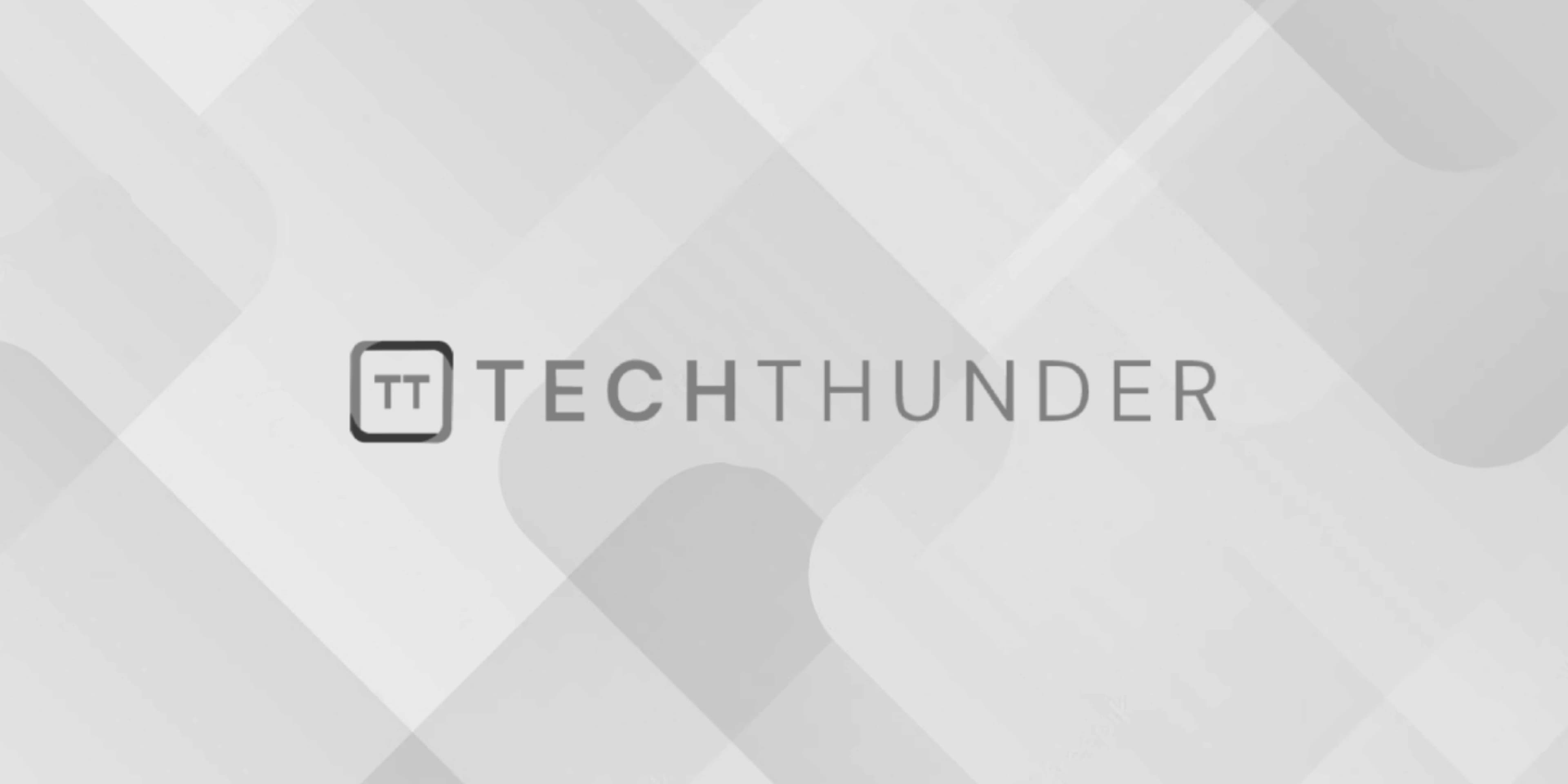
138 views
Remove Duplicates from Sorted Array in C++
To remove duplicates from a sorted array in C++, you can implement a simple algorithm that iterates through the array and eliminates any duplicate elements in-place. Here’s an example of how to do this:
C++
#include <iostream>
#include <vector>
// Function to remove duplicates from a sorted array
int removeDuplicates(std::vector<int>& nums) {
if (nums.empty()) {
return 0; // Return 0 for an empty array
}
int uniqueCount = 1; // Initialize the count of unique elements to 1
for (int i = 1; i < nums.size(); ++i) {
// If the current element is different from the previous one, update it
if (nums[i] != nums[i - 1]) {
nums[uniqueCount] = nums[i];
++uniqueCount;
}
}
return uniqueCount; // Return the count of unique elements
}
int main() {
std::vector<int> nums = {1, 1, 2, 2, 2, 3, 4, 4, 5, 5, 5};
int uniqueCount = removeDuplicates(nums);
std::cout << "Unique Count: " << uniqueCount << std::endl;
std::cout << "Unique Array: ";
for (int i = 0; i < uniqueCount; ++i) {
std::cout << nums[i] << " ";
}
std::cout << std::endl;
return 0;
}
In this example:
- We define a
removeDuplicates
function that takes a reference to astd::vector<int>
as its parameter. - The function iterates through the sorted array and keeps track of a
uniqueCount
variable, which represents the count of unique elements encountered so far. - If the current element is different from the previous one, it is considered a unique element, and we update the array at the position
uniqueCount
with the current element. - After processing the entire array, the function returns the value of
uniqueCount
, representing the count of unique elements. - In the
main
function, we callremoveDuplicates
on a sorted vector of integers and print the count of unique elements as well as the unique elements themselves.
The result of running this program will be:
Unique Count: 5
Unique Array: 1 2 3 4 5
The duplicate elements have been removed from the sorted array, and the unique elements are left in the array with the appropriate count.