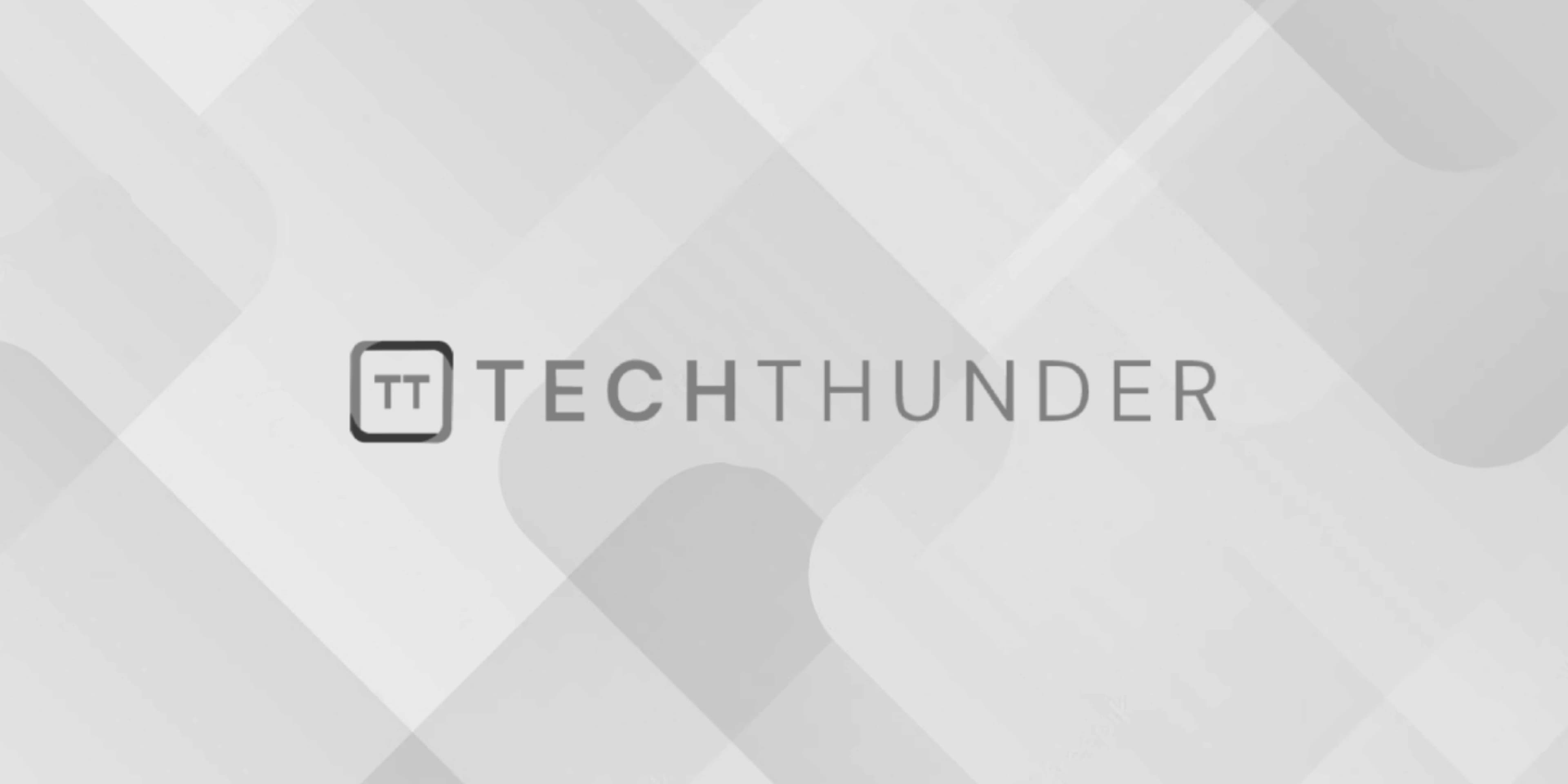
C++ Aggregation
Aggregation in C++ is a type of association between objects that represents a “has-a” relationship. It allows one class to contain or be composed of objects of another class. Aggregation is a form of object composition and is used to model relationships where one class contains or owns another class, but the contained class has an independent existence and can exist outside the scope of the containing class.
Here are the key characteristics and concepts related to aggregation in C++:
- Has-A Relationship: Aggregation represents a “has-a” relationship between classes. It implies that one class (the container or aggregating class) contains objects of another class (the aggregated class).
- Independence: The aggregated class has an independent existence and can exist outside the scope of the containing class. It is not tightly coupled to the container class.
- Ownership: The container class is responsible for the creation, destruction, and management of the aggregated objects. When the container is destroyed, it can destroy the aggregated objects it owns.
- Association: Aggregation is a type of association, but it represents a stronger relationship compared to a simple association. In an association, objects of different classes can interact, but they are not necessarily owned by each other.
Here’s an example of aggregation in C++:
class Department {
public:
Department(const std::string& name) : name(name) {}
// Other Department-related methods and members
private:
std::string name;
};
class Employee {
public:
Employee(const std::string& empName, Department& empDept)
: name(empName), department(empDept) {}
void displayInfo() {
std::cout << "Name: " << name << ", Department: " << department.getName() << std::endl;
}
private:
std::string name;
Department& department; // Aggregated object (Department)
};
int main() {
Department hr("Human Resources");
Employee emp("John Doe", hr);
emp.displayInfo();
return 0;
}
In this example:
Department
is an independent class representing a department.Employee
is another class that represents an employee. It has a member variabledepartment
, which is a reference to aDepartment
object. This represents the aggregation relationship. An employee “has” a department.- In the
main
function, we create aDepartment
objecthr
and anEmployee
objectemp
. TheEmployee
object aggregates aDepartment
object.
Aggregation is a fundamental concept in object-oriented programming that helps model complex relationships between objects. It promotes code reusability, encapsulation, and separation of concerns by allowing objects to collaborate while maintaining their independence.