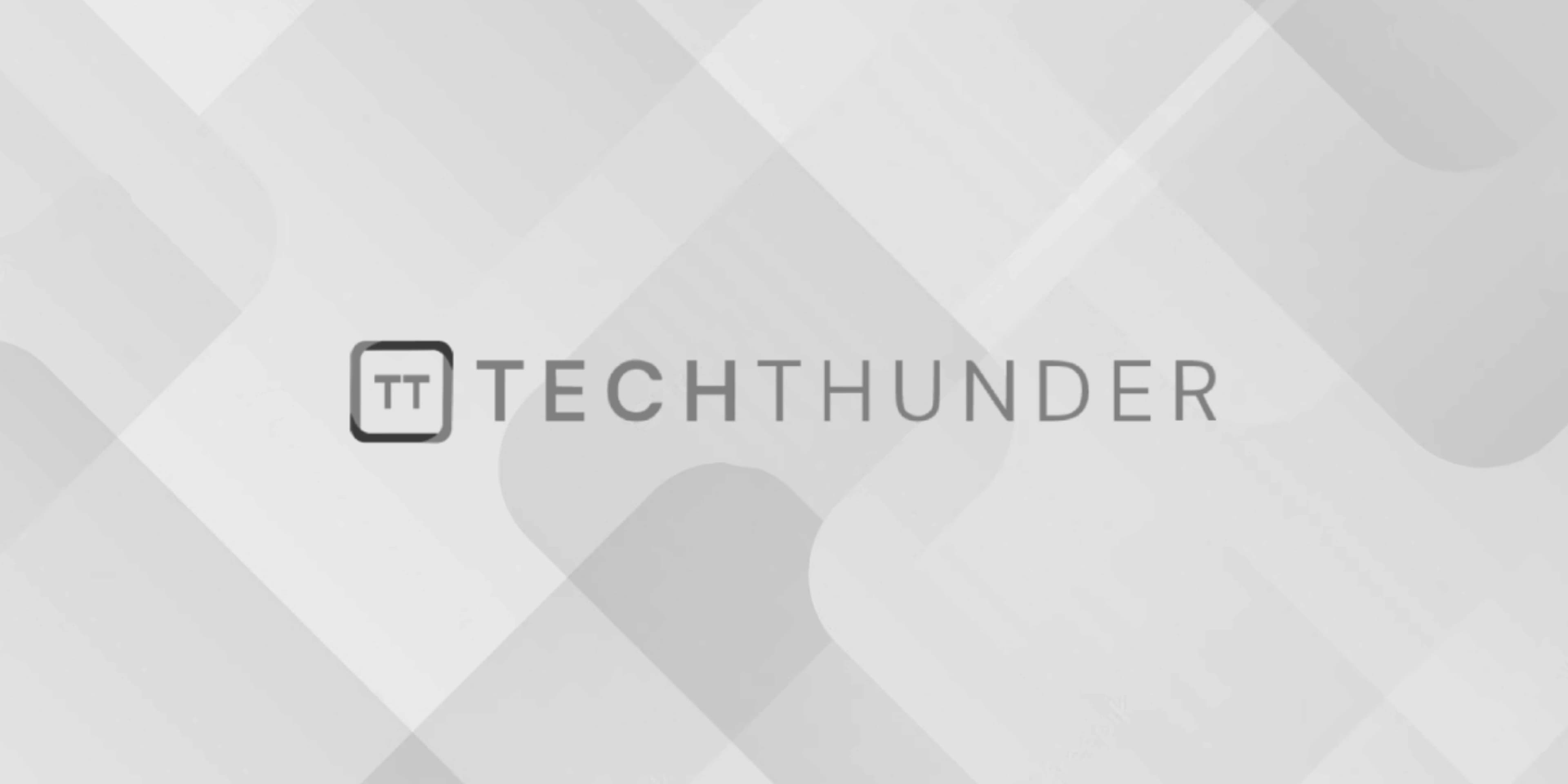
Convert string to integer in C++
The C++ can convert a string to an integer using the std::stoi
(string to integer) function. This function is part of the <string>
header in the C++ Standard Library and is available in C++11 and later versions. Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str = "12345";
int num = std::stoi(str);
std::cout << "The converted integer is: " << num << std::endl;
return 0;
}
In this example, std::stoi
takes a string str
as an argument and returns the integer equivalent. If the conversion is successful, it returns the converted integer. If the conversion fails (for example, if the string contains non-numeric characters), it throws a std::invalid_argument
or std::out_of_range
exception.
If you want to handle conversion failures, you should wrap the call in a try-catch
block:
#include <iostream>
#include <string>
int main() {
std::string str = "12345";
try {
int num = std::stoi(str);
std::cout << "The converted integer is: " << num << std::endl;
}
catch (const std::invalid_argument& ia) {
std::cerr << "Invalid argument: " << ia.what() << std::endl;
}
catch (const std::out_of_range& oor) {
std::cerr << "Out of range: " << oor.what() << std::endl;
}
return 0;
}
This way, you can catch exceptions thrown by std::stoi
if the conversion fails.
Remember that std::stoi
is suitable for converting strings containing integer values. If you need to convert strings to floating-point numbers, you can use std::stof
(string to float) or std::stod
(string to double) for single-precision and double-precision floating-point numbers, respectively. These functions are also available in the <string>
header.