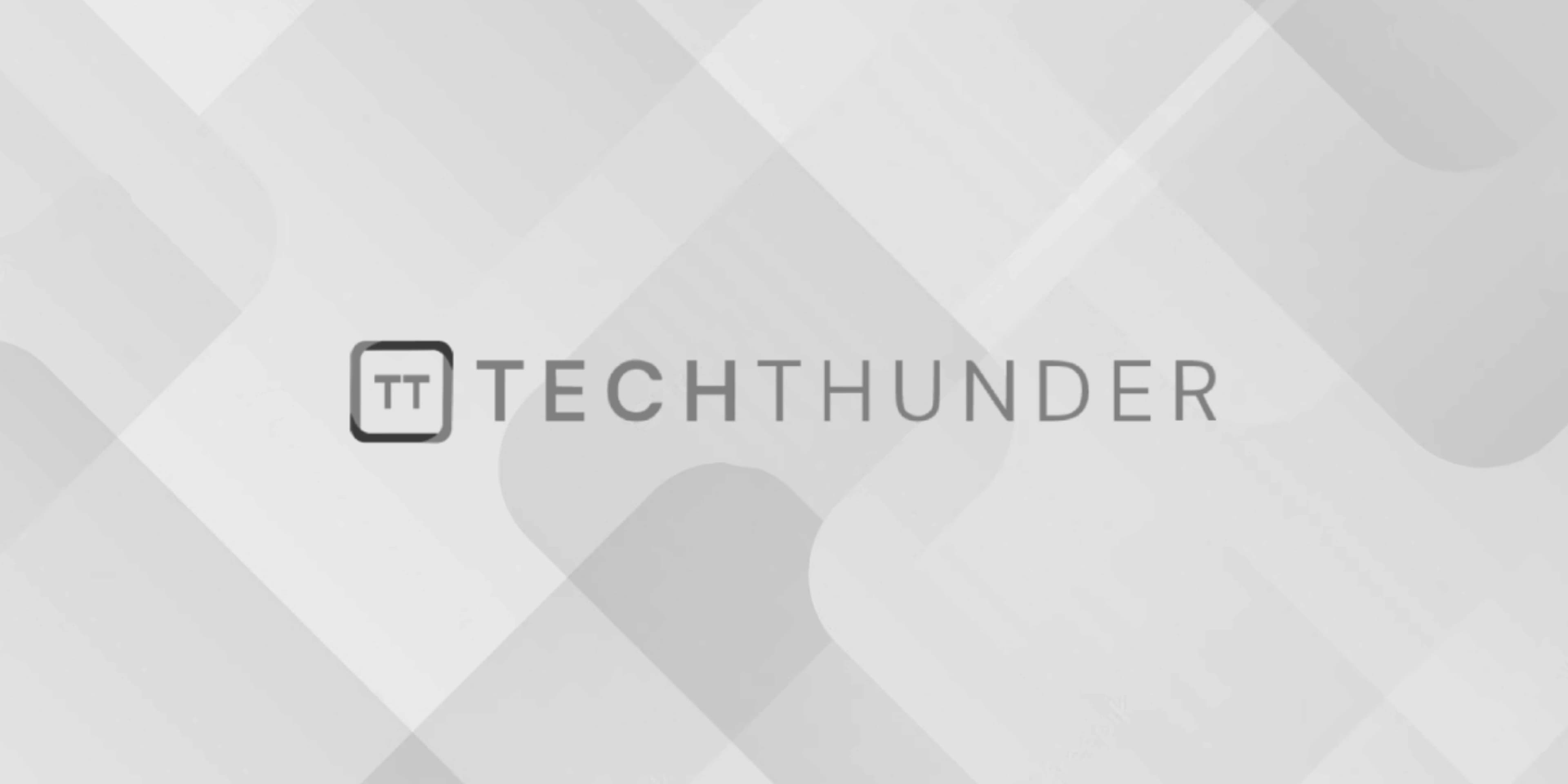
277 views
ToLOWER In C++
The C++, you can convert a string to lowercase using the Standard Library’s tolower
function from the <cctype>
header. You can apply this function to each character in the string to change it to lowercase. Here’s how to do it:
C++
#include <iostream>
#include <string>
#include <cctype> // Include the header for the tolower function
int main() {
std::string originalString = "Hello World";
// Convert each character in the string to lowercase
for (char& c : originalString) {
c = std::tolower(c);
}
std::cout << "Original string: Hello World" << std::endl;
std::cout << "Lowercase string: " << originalString << std::endl;
return 0;
}
In this example:
- We include the
<cctype>
header to access thetolower
function. - We define a string named
originalString
that contains the text you want to convert to lowercase. - We use a
for
loop to iterate through each character in the string. - Inside the loop, we apply
std::tolower(c)
to each characterc
, which converts it to lowercase. We use thestd::tolower
function to ensure proper handling of characters that may have uppercase representations in the current locale.
After running this code, the originalString
will contain the lowercase version of the input string, and it will be printed as “hello world.”