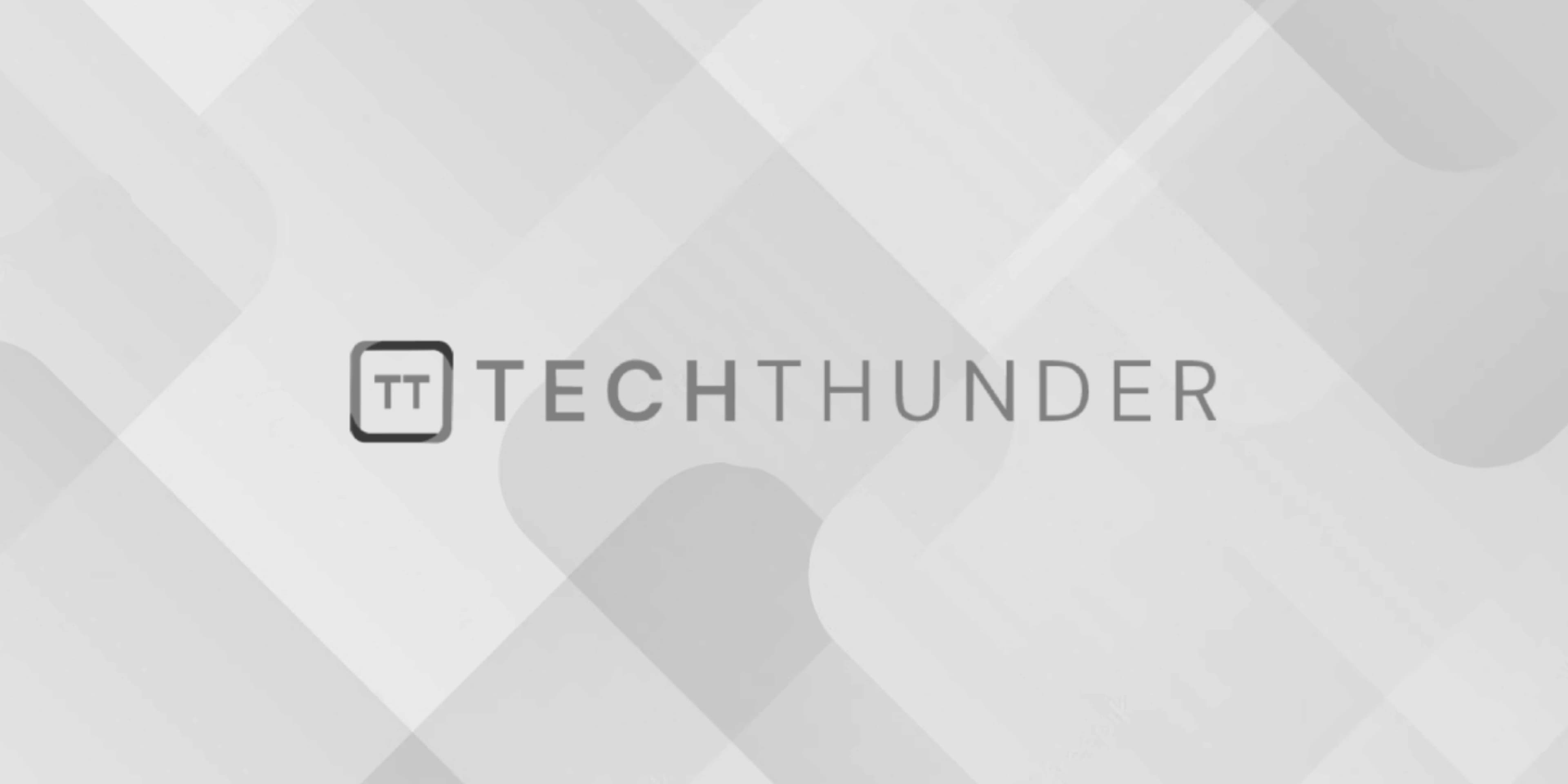
C++ File & Stream
The C++ file handling and stream manipulation are essential features for reading and writing data to and from files. You can work with various types of streams, including input streams (for reading from files), output streams (for writing to files), and string streams (for reading and writing to/from strings).
Here’s an overview of how to work with files and streams in C++:
Opening and Closing Files:
To work with files, you need to open them first and close them when you’re done. The fstream
class is commonly used for file I/O in C++. Here’s how you can open and close files:
#include <iostream>
#include <fstream> // Include the file stream library
int main() {
// Opening a file for writing (creates a new file or overwrites an existing one)
std::ofstream outFile("example.txt");
// Check if the file opened successfully
if (!outFile) {
std::cerr << "Failed to open the file!" << std::endl;
return 1;
}
// Writing data to the file
outFile << "Hello, World!" << std::endl;
outFile << 42 << std::endl;
// Closing the file
outFile.close();
// Opening a file for reading
std::ifstream inFile("example.txt");
// Check if the file opened successfully
if (!inFile) {
std::cerr << "Failed to open the file!" << std::endl;
return 1;
}
// Reading data from the file
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
// Closing the file
inFile.close();
return 0;
}
Stream Operators:
You can use the stream operators (<<
for output and >>
for input) to write and read data from streams. These operators work for various data types, including integers, floating-point numbers, strings, and custom data types (if you’ve overloaded the operators).
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
if (!outFile) {
std::cerr << "Failed to open the file!" << std::endl;
return 1;
}
int num = 42;
double pi = 3.14159;
std::string text = "Hello, World!";
// Writing data to the file
outFile << "Integer: " << num << std::endl;
outFile << "Double: " << pi << std::endl;
outFile << "String: " << text << std::endl;
outFile.close();
return 0;
}
Stream Manipulators:
Stream manipulators are used to control the formatting of data when writing or reading from streams. Some common manipulators include setw
, setprecision
, fixed
, left
, right
, and more. They are typically found in the <iomanip>
header.
#include <iostream>
#include <iomanip> // Include the header for stream manipulators
int main() {
double pi = 3.14159265359;
std::cout << "Default pi: " << pi << std::endl;
std::cout << "Formatted pi: " << std::setprecision(4) << pi << std::endl;
std::cout << "Fixed width: " << std::setw(10) << pi << std::endl;
return 0;
}
These are some of the basics of working with files and streams in C++. You can perform more advanced operations, such as binary file I/O, error handling, and working with custom data types, as needed for your specific applications.