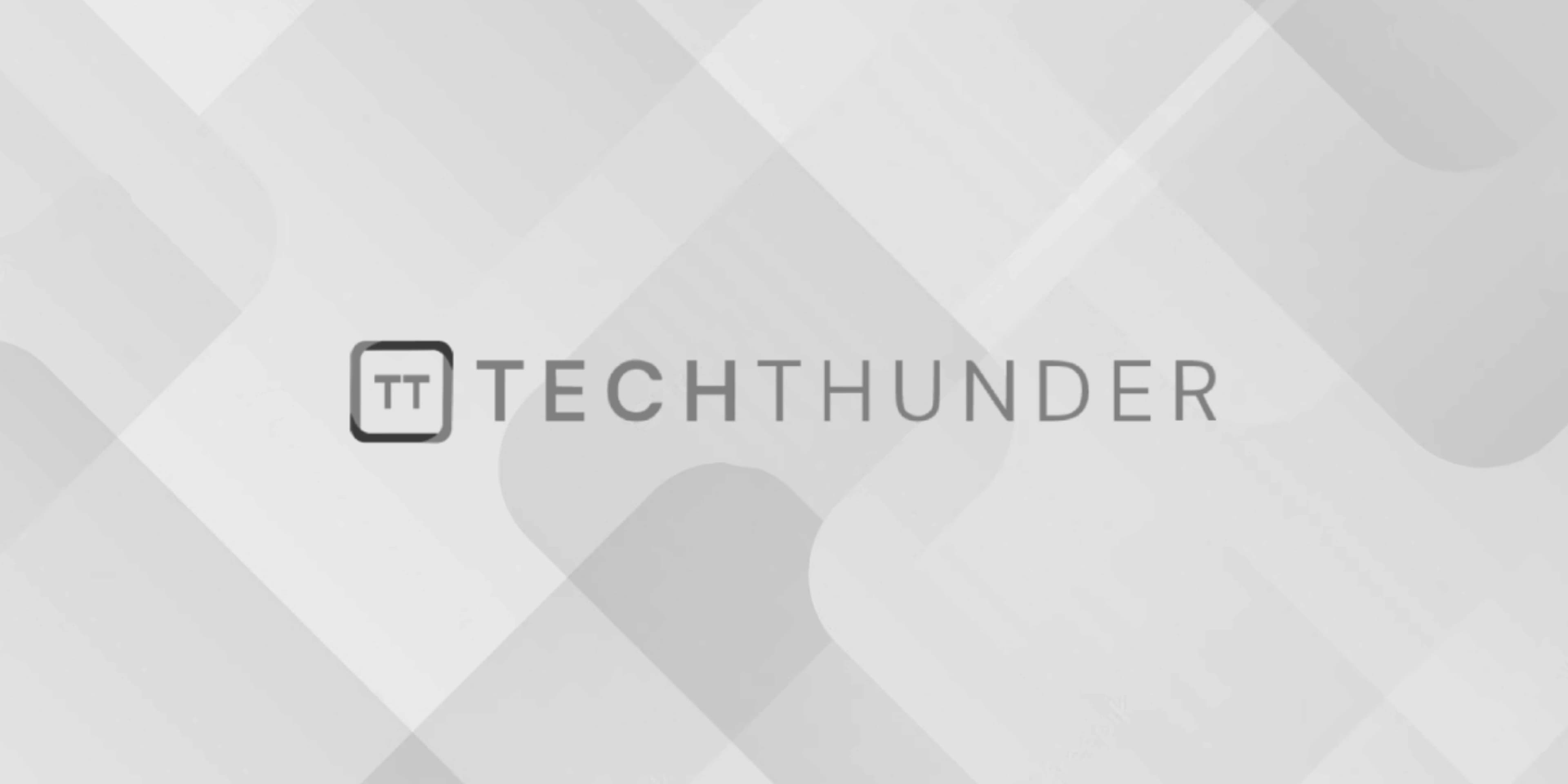
138 views
C++ Program to Count Positive and Negative Numbers in an Array
You can create a C++ program to count positive and negative numbers in an array using a simple loop. Here’s an example program to do that:
C++
#include <iostream>
int main() {
int n; // Size of the array
int positiveCount = 0; // Count of positive numbers
int negativeCount = 0; // Count of negative numbers
// Input the size of the array
std::cout << "Enter the size of the array: ";
std::cin >> n;
// Declare an array of size n
int arr[n];
// Input the elements of the array
std::cout << "Enter the elements of the array:" << std::endl;
for (int i = 0; i < n; i++) {
std::cin >> arr[i];
// Check if the element is positive or negative and update the counts
if (arr[i] > 0) {
positiveCount++;
} else if (arr[i] < 0) {
negativeCount++;
}
}
// Display the counts
std::cout << "Number of positive numbers: " << positiveCount << std::endl;
std::cout << "Number of negative numbers: " << negativeCount << std::endl;
return 0;
}
In this program:
- We first input the size of the array (
n
) and create an integer arrayarr
of that size. - We then input the elements of the array using a
for
loop. - Inside the loop, we check each element of the array to determine if it’s positive (greater than 0) or negative (less than 0), and we update the respective counts accordingly.
- Finally, we display the counts of positive and negative numbers.
Compile and run this program, and it will count the positive and negative numbers in the array you provide as input.