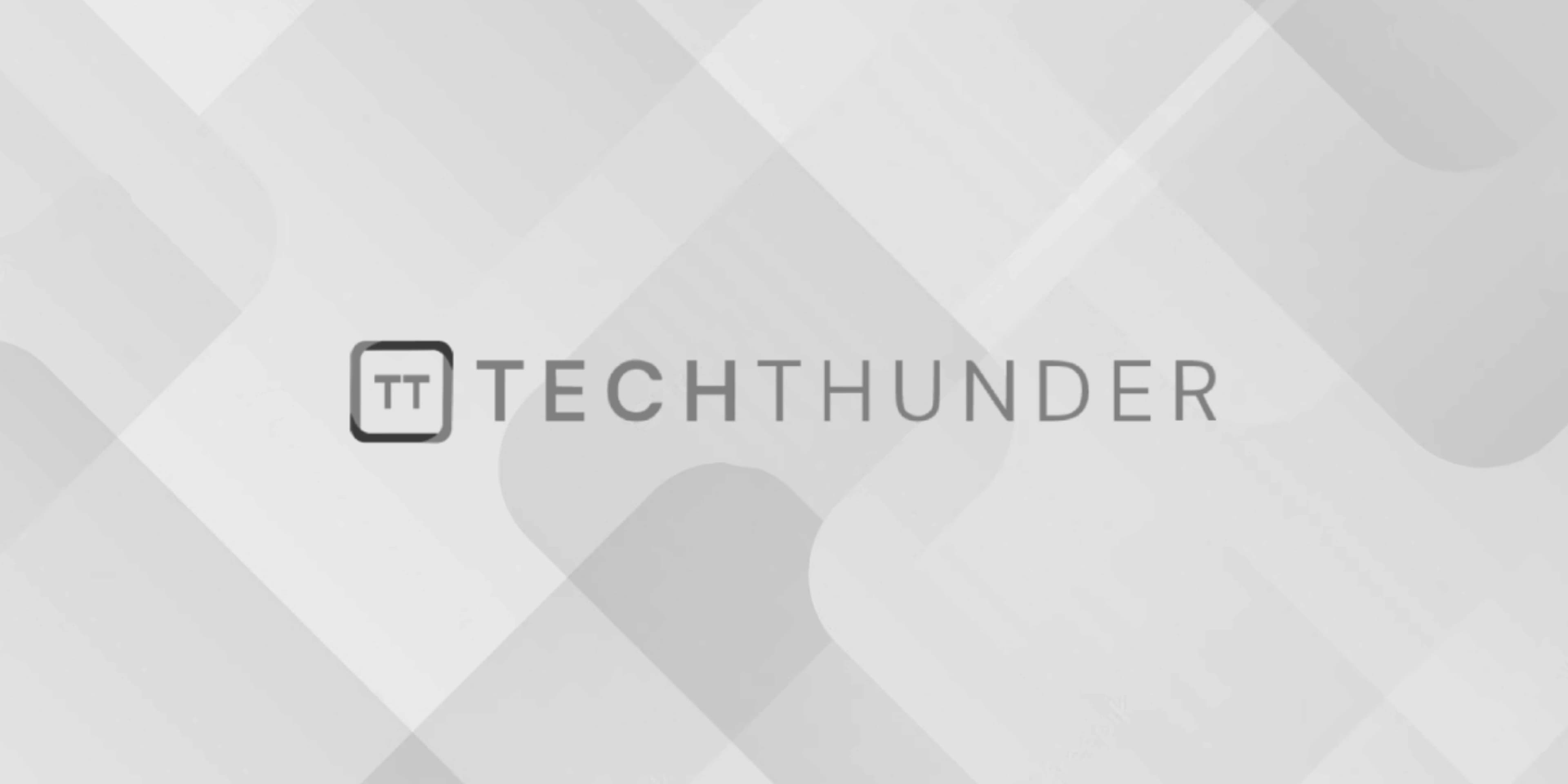
C++ Arrays
The C++ array is a collection of elements of the same data type stored in contiguous memory locations. Arrays provide a convenient way to store and manipulate multiple values of the same type. Here’s how you can work with arrays in C++:
1. Declaring Arrays:
You declare an array by specifying its data type and name, followed by square brackets []
with the size of the array. For example:
int numbers[5]; // Declares an integer array of size 5
2. Initializing Arrays:
Arrays can be initialized at the time of declaration:
int numbers[5] = {1, 2, 3, 4, 5}; // Initialize with values
If you don’t specify values for all elements, the remaining elements will be initialized to zero (or the default value for the data type):
int numbers[5] = {1, 2}; // Initializes the first two elements to 1 and 2, and the rest to 0
3. Accessing Elements:
Array elements are accessed using square brackets []
and an index. The index starts at 0 for the first element and goes up to size - 1
for an array of size size
. For example:
int thirdNumber = numbers[2]; // Accesses the third element (index 2)
4. Modifying Elements:
You can modify array elements by assigning new values to them:
numbers[1] = 42; // Changes the second element to 42
5. Array Size:
The size of an array is fixed and determined at compile time. You can use the sizeof
operator to find the size of an array in bytes:
int size = sizeof(numbers); // Gives the size of the entire array in bytes
6. Iterating Through Arrays:
You can use loops, such as for
or while
, to iterate through array elements:
for (int i = 0; i < 5; ++i) {
// Access and use numbers[i]
}
7. Multi-Dimensional Arrays:
C++ supports multi-dimensional arrays. For example, a two-dimensional array is like a matrix with rows and columns:
int matrix[3][3]; // Declares a 3x3 matrix
matrix[0][0] = 1; // Assigns a value to an element
8. Arrays and Pointers:
Arrays and pointers are closely related in C++. An array’s name can be used as a pointer to its first element:
int numbers[5];
int* ptr = numbers; // 'ptr' points to the first element of 'numbers'
9. Standard Library Arrays:
C++ also provides std::array
and std::vector
from the Standard Library, which offer more safety and flexibility compared to built-in arrays. std::array
has a fixed size, while std::vector
is dynamic in size.
#include <array>
#include <vector>
std::array<int, 5> arr = {1, 2, 3, 4, 5};
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector
is often preferred for its dynamic resizing capabilities and safety features.
Arrays are a fundamental data structure in C++, and understanding how to work with them is essential for many programming tasks. However, consider using Standard Library containers like std::array
or std::vector
for improved safety and ease of use, especially in modern C++ code.