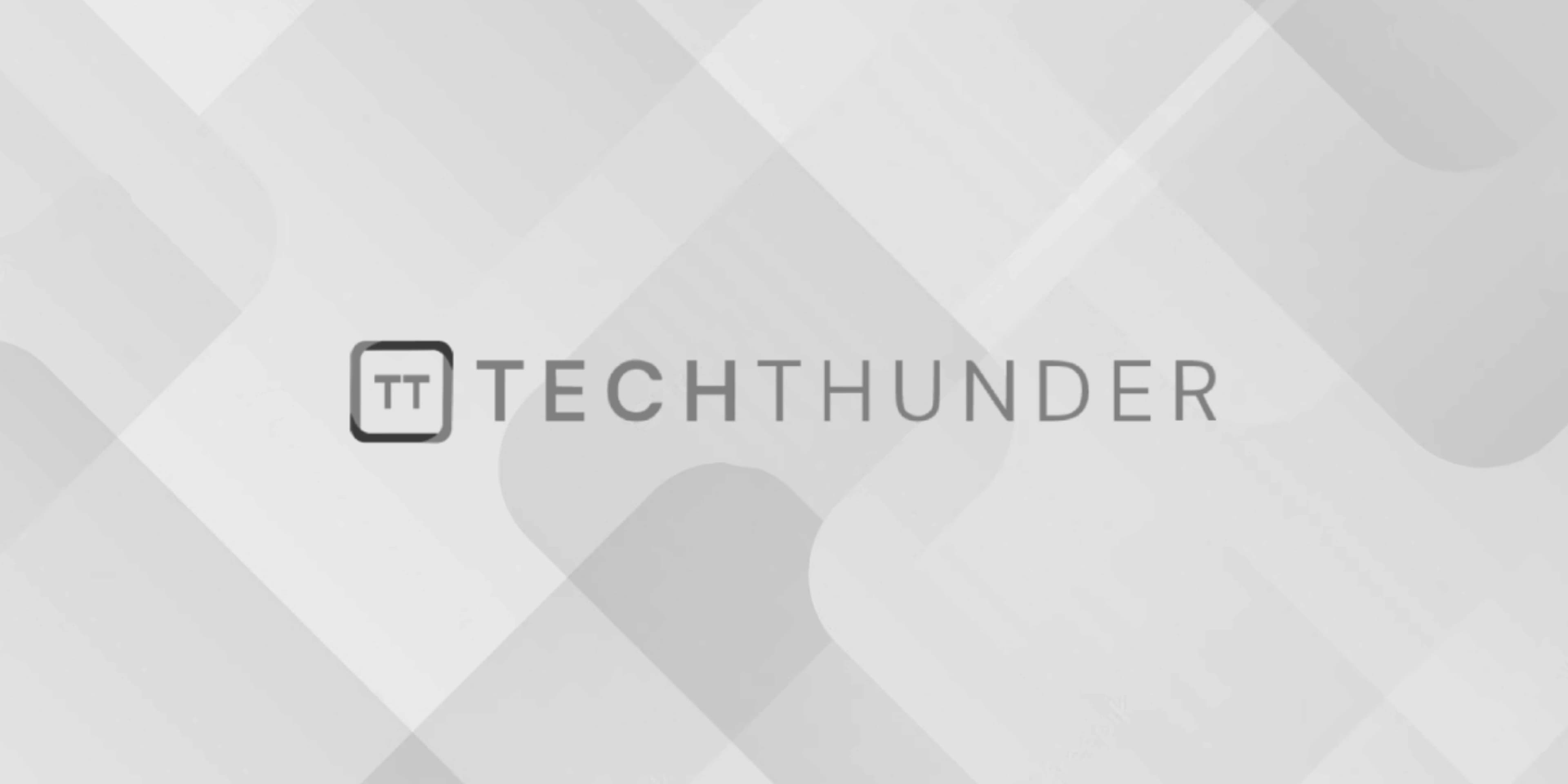
309 views
Modulus of two Float or Double Numbers in C++
The C++ can find the modulus (remainder) of two floating-point numbers (float or double) using the fmod()
function from the <cmath>
(or <math.h>
in C) header. Here’s how you can use it:
C++
#include <iostream>
#include <cmath>
int main() {
double dividend = 10.5;
double divisor = 3.2;
double result = fmod(dividend, divisor);
std::cout << "Modulus (remainder) of " << dividend << " and " << divisor << " is: " << result << std::endl;
return 0;
}
In this example:
- We include the
<cmath>
header to access thefmod()
function. - We define two double variables,
dividend
anddivisor
, which represent the numbers for which we want to find the modulus. - We call the
fmod()
function with thedividend
anddivisor
as arguments, and it returns the modulus of these two numbers. - Finally, we print the result.
Keep in mind that floating-point arithmetic has its limitations, and the result may not always be exact due to rounding errors. Also, be aware of potential division by zero when using this function, as it does not handle division by zero gracefully. You should ensure that the divisor is not zero before calling fmod()
if such a situation is possible in your code.