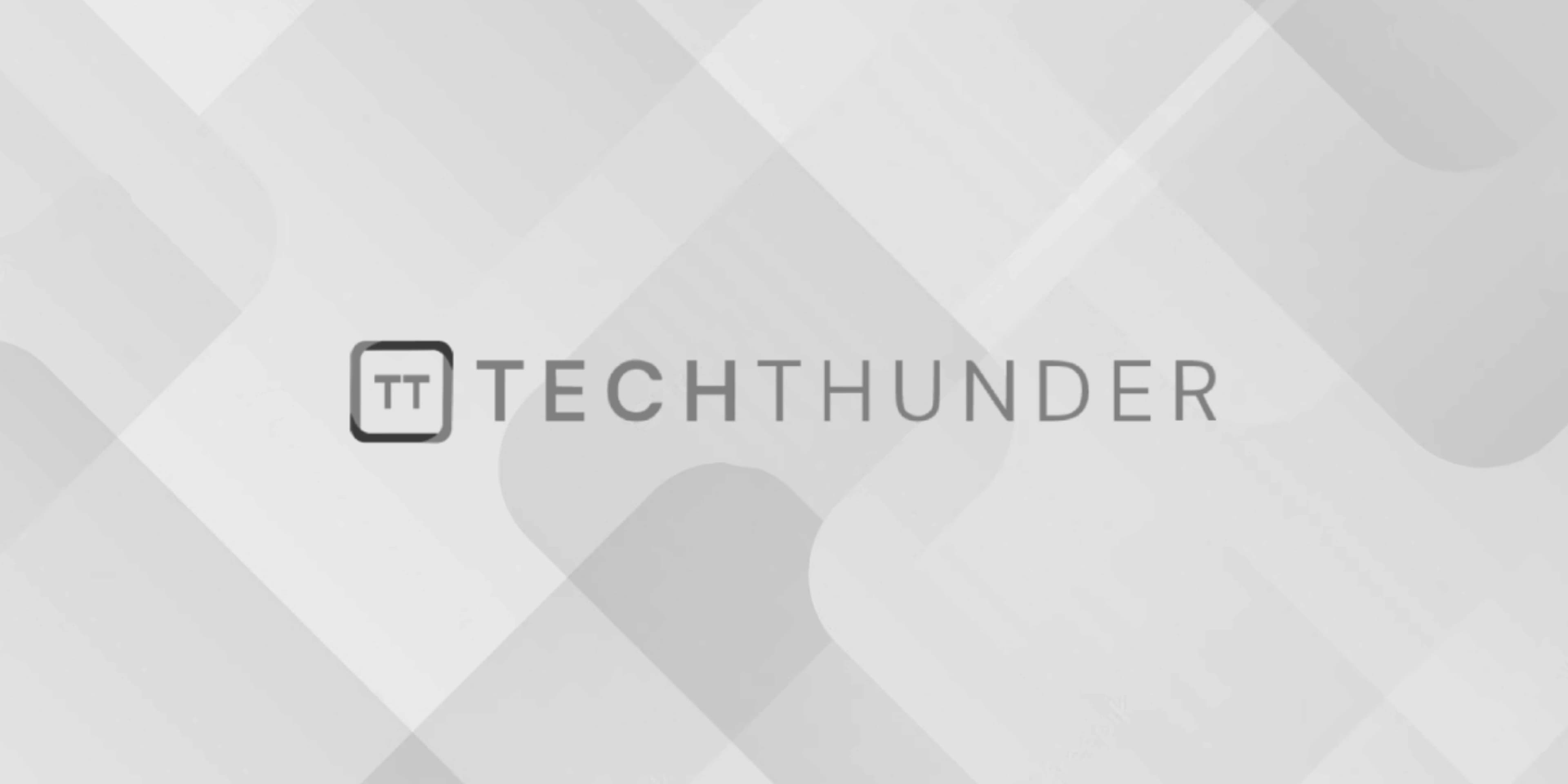
177 views
Billing Management System in C++
Creating a billing management system in C++ involves designing a program that can handle customer orders, calculate bills, and manage product information. Below is a simplified example of a billing management system in C++. Please note that this is a basic implementation and does not cover all the complexities that a real-world system would entail, such as a database for product information or user authentication.
C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a Product struct to represent products
struct Product {
string name;
double price;
Product(const string& n, double p) : name(n), price(p) {}
};
// Define an Order struct to represent customer orders
struct Order {
string productName;
int quantity;
Order(const string& n, int q) : productName(n), quantity(q) {}
};
// Function to calculate the total cost of an order
double calculateTotalCost(const vector<Product>& products, const vector<Order>& orders) {
double totalCost = 0.0;
for (const Order& order : orders) {
for (const Product& product : products) {
if (order.productName == product.name) {
totalCost += order.quantity * product.price;
break;
}
}
}
return totalCost;
}
int main() {
vector<Product> products;
vector<Order> orders;
// Add some products to the system
products.push_back(Product("Product1", 10.0));
products.push_back(Product("Product2", 15.0));
products.push_back(Product("Product3", 20.0));
// Allow the user to create an order
while (true) {
string productName;
int quantity;
cout << "Enter product name (or 'exit' to finish): ";
cin >> productName;
if (productName == "exit") {
break;
}
cout << "Enter quantity: ";
cin >> quantity;
orders.push_back(Order(productName, quantity));
}
// Calculate and display the total cost
double totalCost = calculateTotalCost(products, orders);
cout << "Total Cost: $" << totalCost << endl;
return 0;
}
In this simplified example:
- We define a
Product
struct to represent products with a name and price. - We define an
Order
struct to represent customer orders with a product name and quantity. - The
calculateTotalCost
function calculates the total cost of the customer’s order by matching the products in the order with the products in the system. - In the
main
function, we create a list of products and allow the user to create an order by entering product names and quantities. - The program calculates and displays the total cost of the order.
This is a basic billing management system. In a real-world scenario, you would likely need to add more features such as customer information, product databases, and a more user-friendly interface.