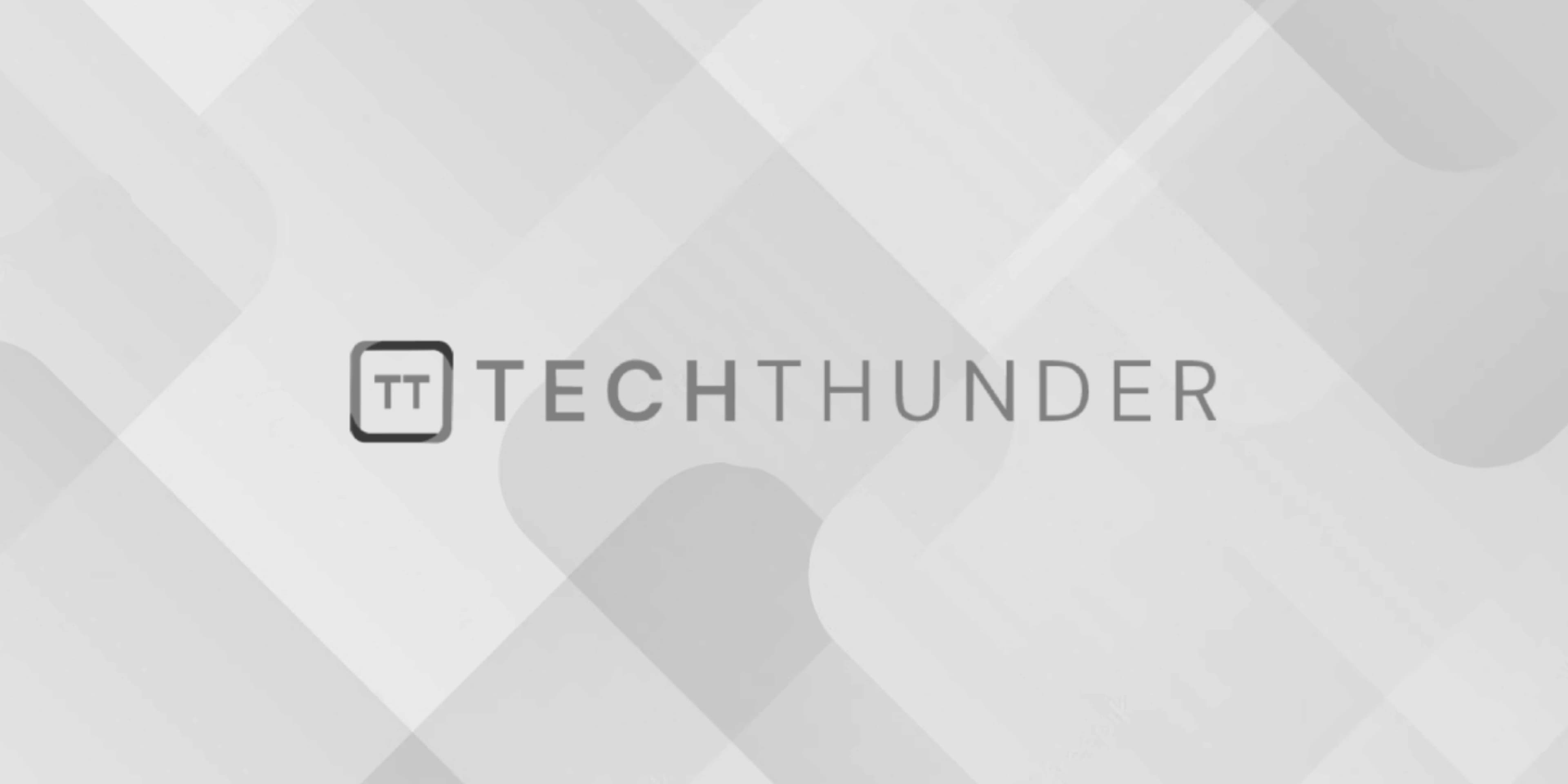
Constructor overloading in C++
Constructor overloading in C++ is a feature that allows you to define multiple constructors for a class with the same name but different parameter lists. This enables you to create objects of the class using different sets of arguments when you instantiate them. The appropriate constructor is called based on the number and types of arguments passed during object creation. Constructor overloading is a form of polymorphism and is commonly used to provide flexibility in object initialization.
Here’s an example of constructor overloading in C++:
#include <iostream>
class MyClass {
public:
// Default constructor with no parameters
MyClass() {
std::cout << "Default constructor called" << std::endl;
}
// Constructor with one parameter
MyClass(int x) {
std::cout << "Constructor with one parameter called. x = " << x << std::endl;
}
// Constructor with two parameters
MyClass(int x, int y) {
std::cout << "Constructor with two parameters called. x = " << x << ", y = " << y << std::endl;
}
};
int main() {
MyClass obj1; // Calls the default constructor
MyClass obj2(42); // Calls the constructor with one parameter
MyClass obj3(10, 20); // Calls the constructor with two parameters
return 0;
}
In this example, the MyClass
class has three constructors: a default constructor with no parameters, a constructor with one integer parameter, and a constructor with two integer parameters. Depending on how you create MyClass
objects, the appropriate constructor is called.
Constructor overloading can be helpful when you want to initialize objects in different ways or when you want to provide default values for some of the object’s attributes while allowing customization through different constructor arguments.