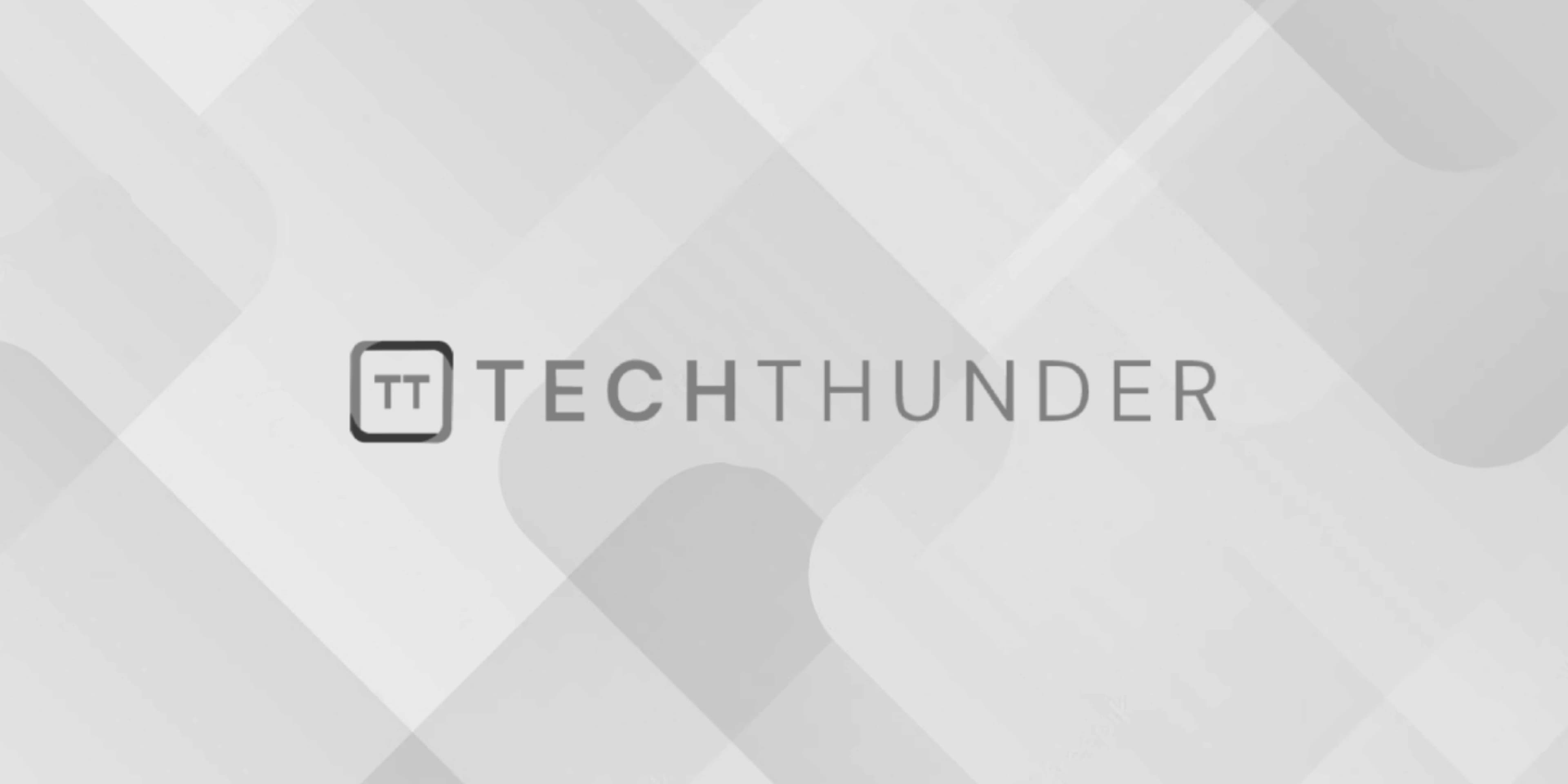
C++ Operators
The C++ operators are symbols that perform operations on one or more operands (variables or values). C++ supports a wide variety of operators that can be categorized into different groups based on their functionality. Here are some of the most commonly used operators in C++:
- Arithmetic Operators:
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulus or Remainder)
- Relational Operators:
==
(Equal to)!=
(Not equal to)<
(Less than)>
(Greater than)<=
(Less than or equal to)>=
(Greater than or equal to)
- Logical Operators:
&&
(Logical AND)||
(Logical OR)!
(Logical NOT)
- Assignment Operators:
=
(Assignment)+=
(Addition assignment)-=
(Subtraction assignment)*=
(Multiplication assignment)/=
(Division assignment)%=
(Modulus assignment)
- Increment and Decrement Operators:
++
(Increment)--
(Decrement)
- Conditional (Ternary) Operator:
condition ? expr1 : expr2
(Conditional operator, also known as the ternary operator)
- Bitwise Operators:
&
(Bitwise AND)|
(Bitwise OR)^
(Bitwise XOR)~
(Bitwise NOT)<<
(Left shift)>>
(Right shift)
- Member Access Operators:
.
(Dot operator for accessing members of a class or structure)->
(Arrow operator for accessing members through pointers)
- Other Operators:
sizeof
(Size of an object or data type),
(Comma operator, used to separate expressions in a statement)()
(Function call operator)[]
(Array subscript operator)&
(Address-of operator)*
(Dereference operator)
- Type Casting Operators:
static_cast
dynamic_cast
const_cast
reinterpret_cast
These operators are used in various combinations to perform mathematical calculations, make logical decisions, and manipulate data in C++ programs. The specific behavior of an operator depends on the data types of its operands and its context within an expression.
Here are some examples of how operators are used in C++:
int a = 5, b = 3;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int product = a * b; // Multiplication
int quotient = a / b; // Division
int remainder = a % b; // Modulus
bool isGreater = (a > b); // Relational operator
bool andResult = (true && false); // Logical AND
bool orResult = (true || false); // Logical OR
bool notResult = !true; // Logical NOT
a++; // Increment
b--; // Decrement
int max = (a > b) ? a : b; // Conditional operator (ternary)
int x = 10, y = 3;
int bitwiseAnd = x & y; // Bitwise AND
int bitwiseOr = x | y; // Bitwise OR
int bitwiseXor = x ^ y; // Bitwise XOR
int bitwiseNot = ~x; // Bitwise NOT
int leftShift = x << 2; // Left shift by 2 bits
int rightShift = x >> 1; // Right shift by 1 bit
Understanding operators is fundamental to writing C++ code, as they provide the means to perform various operations on data, control program flow, and manipulate memory at a low level.