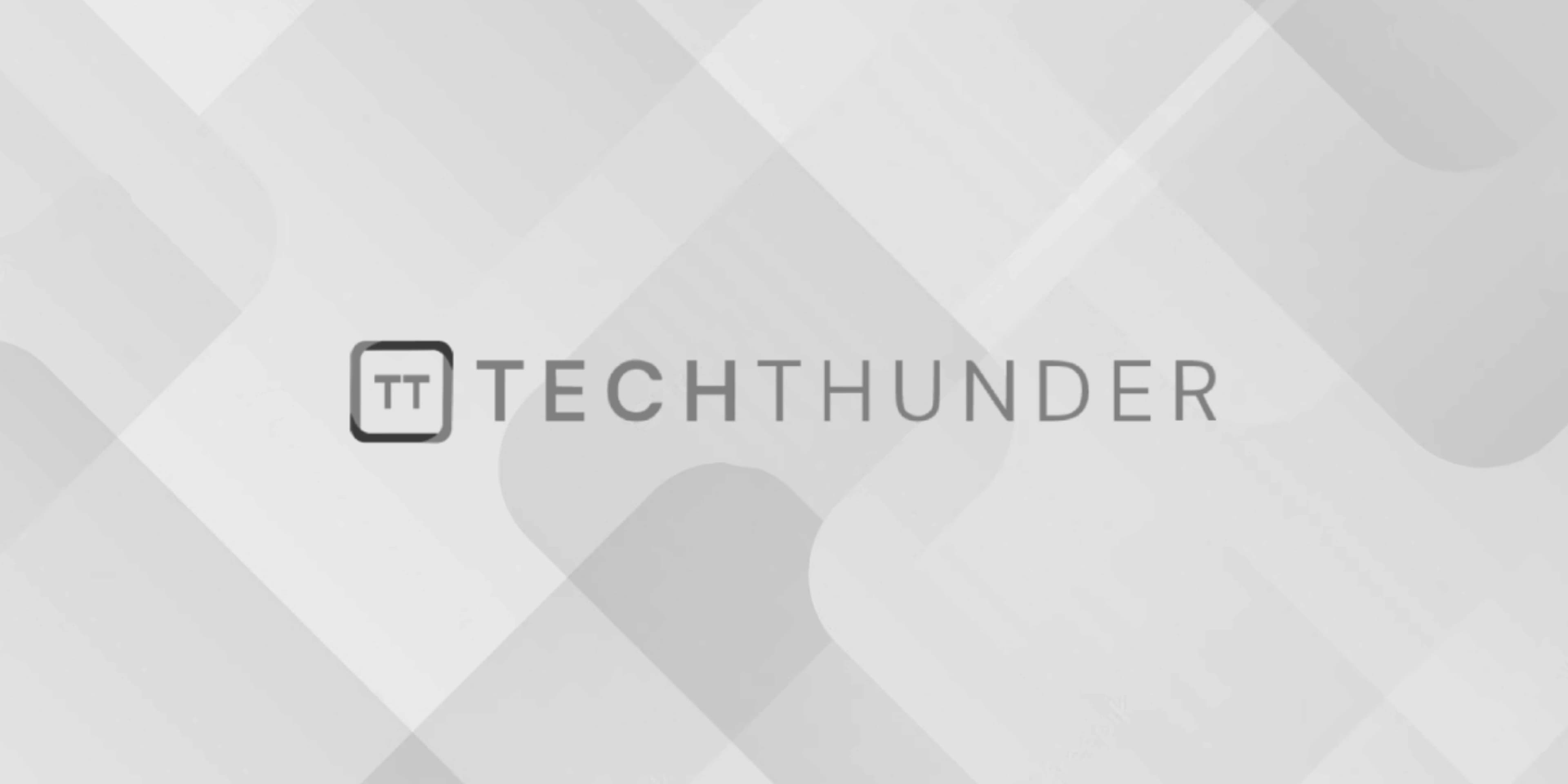
113 views
How to Create and Use unique_ptr Instances in C++
The C++ std::unique_ptr
is a smart pointer that provides automatic memory management for dynamically allocated objects. It ensures that the memory allocated for an object is automatically released when the std::unique_ptr
goes out of scope. Here’s how you can create and use std::unique_ptr
instances:
- Creating a
std::unique_ptr
: You can create astd::unique_ptr
using thestd::make_unique
function (C++14 and later) or by directly constructing it with thenew
operator (not recommended for safety reasons).
C++
// Using std::make_unique (preferred in C++14 and later)
std::unique_ptr<int> ptr = std::make_unique<int>(42);
// Using direct construction with new (less safe)
std::unique_ptr<int> ptr(new int(42));
- Accessing the Managed Object: You can access the managed object through the
std::unique_ptr
using theget()
method or by dereferencing the pointer with the*
operator.
C++
int* rawPtr = ptr.get(); // Get a raw pointer to the managed object
int value = *ptr; // Dereference the unique_ptr to access the object
- Checking if the
std::unique_ptr
is valid: You can check if astd::unique_ptr
is managing an object or is in a “null” state (i.e., not managing any object) using thenullptr
comparison:
C++
if (ptr) {
// The unique_ptr is managing an object
} else {
// The unique_ptr is in a null state
}
- Transferring Ownership: Since
std::unique_ptr
enforces exclusive ownership, you can transfer ownership of a managed object to anotherstd::unique_ptr
using move semantics. This effectively transfers the responsibility of releasing the memory to the newstd::unique_ptr
.
C++
std::unique_ptr<int> ptr1 = std::make_unique<int>(42);
std::unique_ptr<int> ptr2 = std::move(ptr1); // Ownership transferred to ptr2
- Releasing Ownership: If you want to release ownership of the managed object without destroying it, you can use the
release()
method. After callingrelease()
, thestd::unique_ptr
will be in a null state, and you’ll be responsible for managing the memory.
C++
int* releasedPtr = ptr.release(); // Release ownership
// Now ptr is null, and you are responsible for deleting the object manually
delete releasedPtr;
- Automated Cleanup: The primary advantage of
std::unique_ptr
is that it automatically cleans up the managed object when it goes out of scope. You don’t need to explicitly calldelete
to deallocate the memory.
C++
// Automatically cleans up the managed object when ptr goes out of scope
std::unique_ptr
is an excellent choice for managing dynamically allocated objects and helps prevent memory leaks by design. Use it when you have exclusive ownership of an object or when you want to transfer ownership between scopes.